Convert pandas dataframe to NumPy array
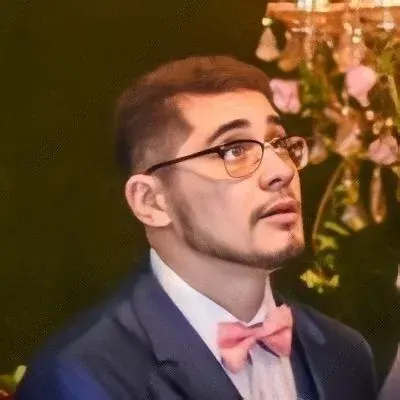
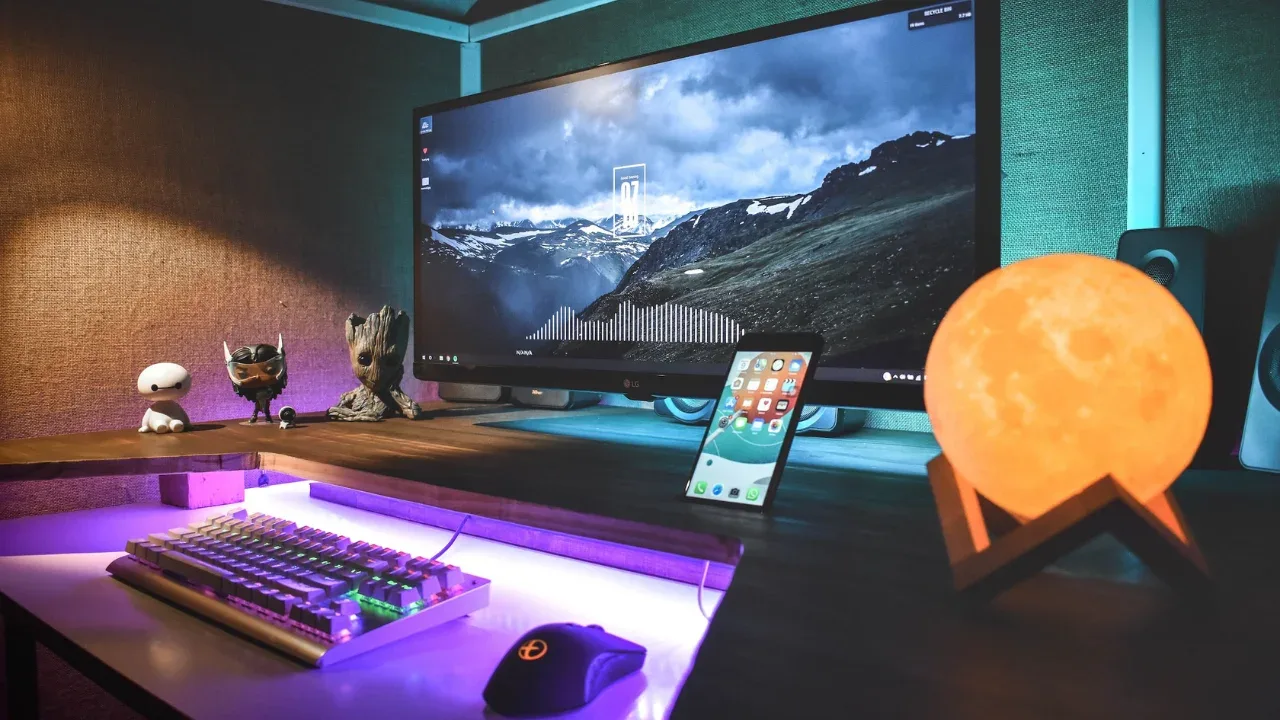
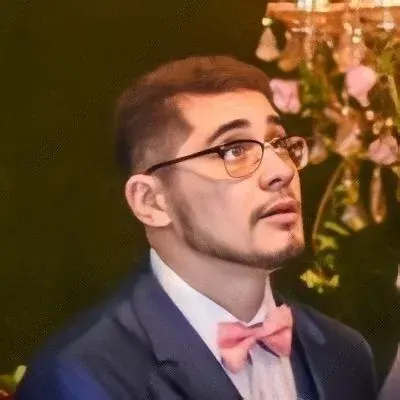
Converting a Pandas DataFrame to a NumPy Array: A Complete Guide π¨βπ»π
Are you struggling with converting a Pandas DataFrame to a NumPy array? Don't worry, you're not alone! It's a common question among data analysts and scientists. In this blog post, we will explore the different ways to convert a Pandas DataFrame to a NumPy array and provide easy solutions to common issues. πΌπ’
The Problem: Converting a Pandas DataFrame to a NumPy Array π
Let's start by understanding the problem at hand. We have a Pandas DataFrame that looks like this:
import numpy as np
import pandas as pd
index = [1, 2, 3, 4, 5, 6, 7]
a = [np.nan, np.nan, np.nan, 0.1, 0.1, 0.1, 0.1]
b = [0.2, np.nan, 0.2, 0.2, 0.2, np.nan, np.nan]
c = [np.nan, 0.5, 0.5, np.nan, 0.5, 0.5, np.nan]
df = pd.DataFrame({'A': a, 'B': b, 'C': c}, index=index)
df = df.rename_axis('ID')
print(df)
This DataFrame looks like:
A B C
ID
1 NaN 0.2 NaN
2 NaN NaN 0.5
3 NaN 0.2 0.5
4 0.1 0.2 NaN
5 0.1 0.2 0.5
6 0.1 NaN 0.5
7 0.1 NaN NaN
Our goal is to convert this DataFrame to a NumPy array. The desired output should look like:
array([[ nan, 0.2, nan],
[ nan, nan, 0.5],
[ nan, 0.2, 0.5],
[ 0.1, 0.2, nan],
[ 0.1, 0.2, 0.5],
[ 0.1, nan, 0.5],
[ 0.1, nan, nan]])
Solution 1: Using the .values
Attribute π₯
The simplest solution to convert a Pandas DataFrame to a NumPy array is to use the .values
attribute. Here's how you can do it:
np_array = df.values
This will transform your DataFrame into a NumPy array. Easy as π₯§!
Solution 2: Using the .to_numpy()
Method π€
If you prefer a more explicit method, you can also use the .to_numpy()
method to achieve the same result:
np_array = df.to_numpy()
This method provides the same outcome as .values
but reads more intuitively for some users.
Preserving dtypes in the NumPy Array π’
Now, let's tackle the second part of the question: preserving the dtypes in the NumPy array. It's definitely possible to do that! Here's how you can achieve it:
structured_array = df.to_records(index=True)
This will give you a structured NumPy array that preserves the dtypes as requested:
array([(1, nan, 0.2, nan), (2, nan, nan, 0.5), (3, nan, 0.2, 0.5),
(4, 0.1, 0.2, nan), (5, 0.1, 0.2, 0.5), (6, 0.1, nan, 0.5),
(7, 0.1, nan, nan)],
dtype=[('ID', '<i4'), ('A', '<f8'), ('B', '<f8'), ('C', '<f8')])
Conclusion and Call-to-Action ππ£
Converting a Pandas DataFrame to a NumPy array is a common task, and now you have two easy solutions up your sleeve: using the .values
attribute or the .to_numpy()
method.
Don't forget that you can also preserve the dtypes in the NumPy array by using the .to_records()
method.
So, next time you need to convert a DataFrame to a NumPy array, you'll know exactly what to do! π
If you found this guide helpful, feel free to share it with others who might benefit from it. And don't hesitate to leave a comment below if you have any questions or want to share your own tips on working with arrays.
Happy coding! π»π