Convert Pandas column containing NaNs to dtype `int`
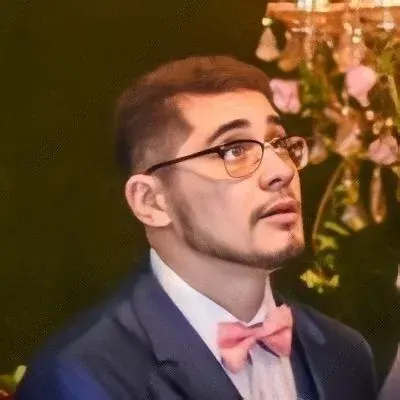
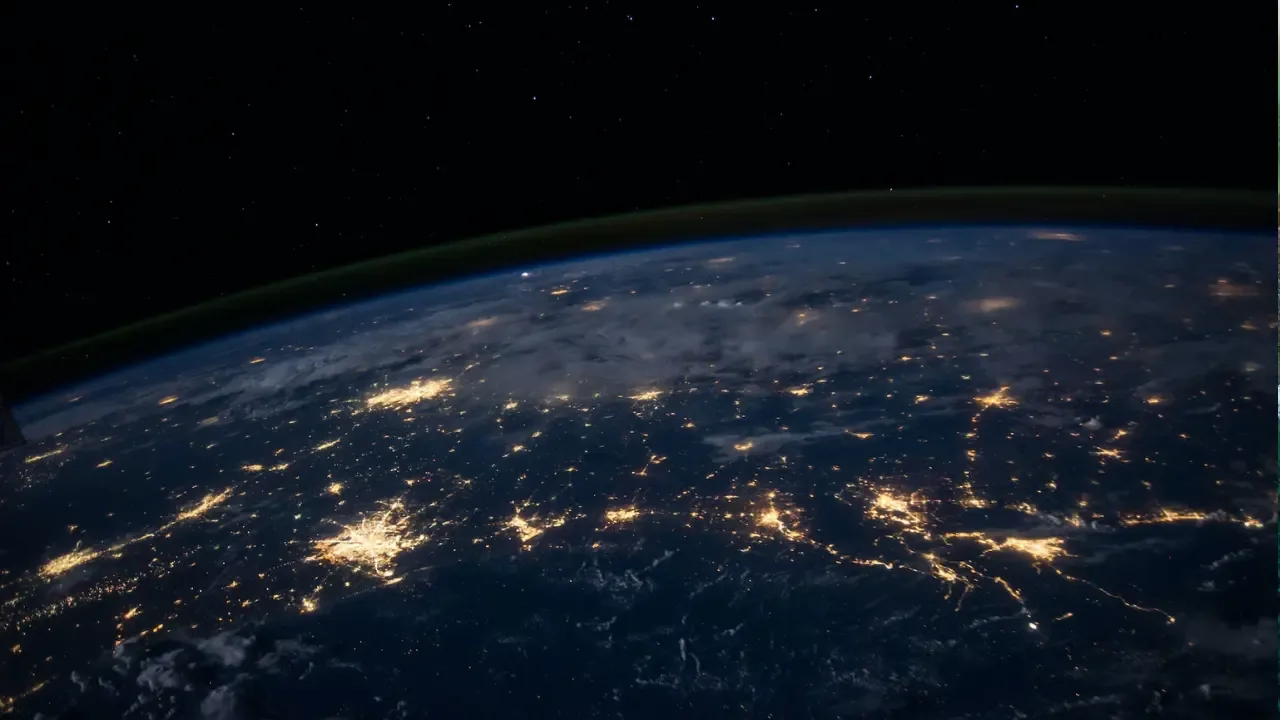
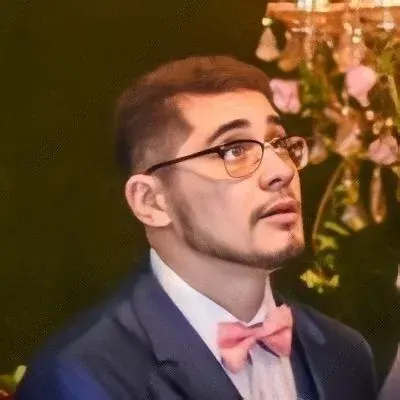
How to Convert Pandas Column Containing NaNs to dtype int
So, you want to convert a column in your Pandas dataframe to int
datatype, but you're running into issues because the column contains NaNs (missing values). Don't worry, I've got you covered! In this blog post, I'll walk you through common issues and provide easy solutions to help you tackle this problem.
The Error Message
Let's start by addressing the error messages you encountered while trying to convert the id
column to an integer datatype. The first error you received when trying to specify the datatype during the .csv
file read operation was:
df = pd.read_csv("data.csv", dtype={'id': int})
error: Integer column has NA values
The second error occurred when you attempted to convert the column type after reading the .csv
file:
df = pd.read_csv("data.csv")
df[['id']] = df[['id']].astype(int)
error: Cannot convert NA to integer
Understanding the Issue
The error messages indicate that the presence of NaNs in the id
column is causing the conversion to fail. NaNs represent missing or undefined values, and they cannot be converted directly to integers.
Solution: Handling NaNs
Now that we understand the issue, let's explore some easy solutions to tackle it.
Solution 1: Replace NaNs with a Default Value
One way to handle NaNs is by replacing them with a default value before converting the column to int
. You can use the fillna()
method to replace NaNs with a specific value, like 0, before converting the column type:
df = pd.read_csv("data.csv")
df[['id']].fillna(0, inplace=True) # Replace NaNs with 0
df[['id']] = df[['id']].astype(int) # Convert to int
Solution 2: Convert NaNs to a Nullable Integer Type
Another approach is to convert the column to a nullable integer type that allows NaNs. You can achieve this by using the Int64
datatype from the pandas
library:
import pandas as pd
df = pd.read_csv("data.csv")
df['id'] = pd.to_numeric(df['id'], errors='coerce') # Convert to nullable integer
By using pd.to_numeric()
with errors='coerce'
, NaNs will be replaced with None
, making the column nullable. Note that this solution requires pandas version 1.0 or higher.
Call-to-Action: Engage and Share!
I hope these solutions help you convert a Pandas column containing NaNs to the int
datatype without any issues. Now it's your turn to put this knowledge into practice! Try out the solutions and let me know in the comments which one worked best for you.
If you found this blog post helpful, don't forget to share it with your friends and colleagues who might also benefit from it. Happy coding! 😄🚀🔥