Convert list of dictionaries to a pandas DataFrame
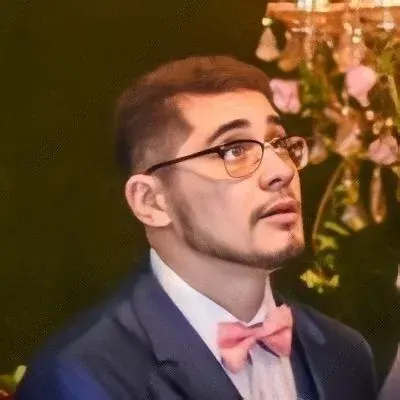
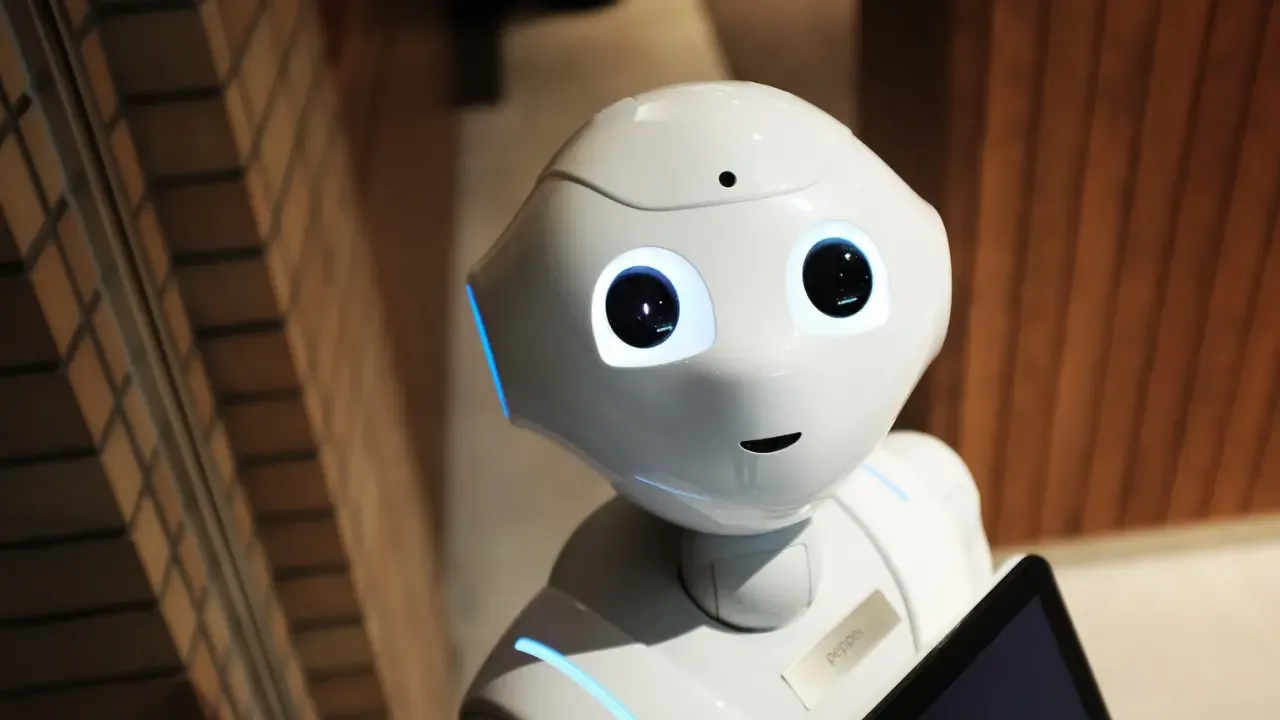
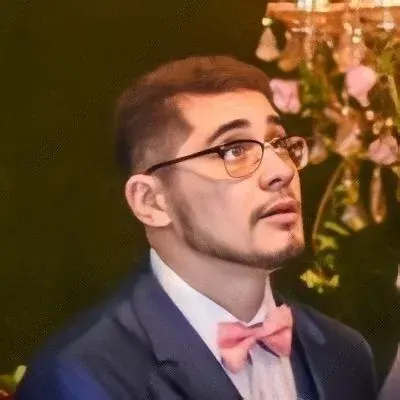
Convert List of Dictionaries to a Pandas DataFrame
So you have a list of dictionaries and you want to convert it into a Pandas DataFrame? No worries, I've got you covered! 📊
The Problem
The problem statement states that we have a list of dictionaries, and we want to transform it into a Pandas DataFrame. The desired output DataFrame is also given for reference.
The Solution
Step 1: Import the Pandas Library
First things first, let's import the necessary libraries. We'll be using Pandas, so make sure you have it installed. If not, you can install it using pip
.
import pandas as pd
Step 2: Define the List of Dictionaries
Next, let's define the list of dictionaries that we want to convert into a DataFrame. In this example, the list is already provided:
data = [{'points': 50, 'time': '5:00', 'year': 2010},
{'points': 25, 'time': '6:00', 'month': 'february'},
{'points': 90, 'time': '9:00', 'month': 'january'},
{'points_h1': 20, 'month': 'june'}]
Step 3: Convert the List of Dictionaries to a DataFrame
Now comes the exciting part! We'll use the pd.DataFrame()
function to convert the list of dictionaries into a Pandas DataFrame.
df = pd.DataFrame(data)
Step 4: Check the Output
You did it! 🎉 Now, let's check the resulting DataFrame by simply printing it.
print(df)
Output:
month points points_h1 time year
0 NaN 50 NaN 5:00 2010.0
1 february 25 NaN 6:00 NaN
2 january 90 NaN 9:00 NaN
3 june NaN 20.0 NaN NaN
Voila! The list of dictionaries has been successfully converted into a Pandas DataFrame.
Common Issues
Missing Values
In the given example, you might have noticed the presence of NaN
values in the DataFrame. These NaN
values occur when a key-value pair is missing in a dictionary. Pandas automatically fills those missing values with NaN
(Not a Number).
Order of Columns
The order of columns in the resulting DataFrame might not always match the order of keys in the dictionaries. But don't worry, the order of columns does not matter as long as you have all the required columns present.
Conclusion
Converting a list of dictionaries into a Pandas DataFrame is simple and straightforward. Just follow the steps outlined in this guide, and you'll be able to transform your data with ease.
Now it's your turn to give it a try! Try converting your own list of dictionaries into a DataFrame and see what happens. Don't forget to share your results in the comments below. Happy coding! 💻🐍
References:
Pandas Documentation: pandas.DataFrame