Convert JSON string to dict using Python
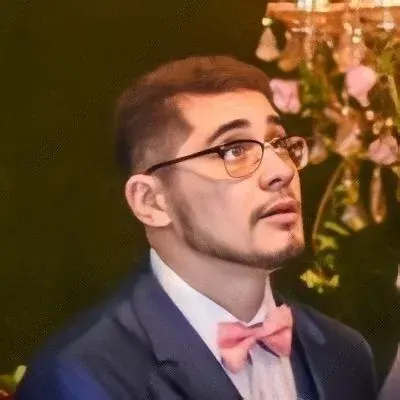
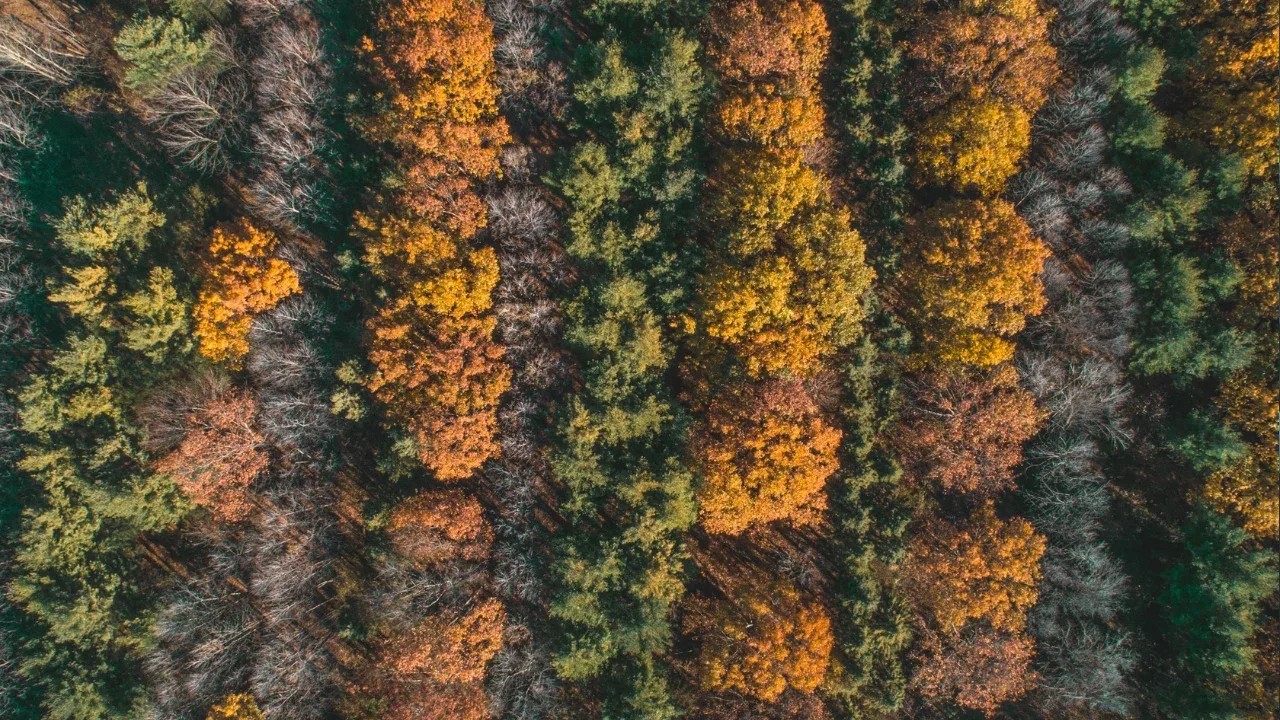
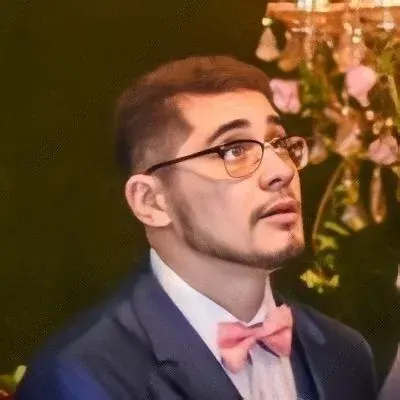
Converting JSON String to Dictionary in Python π
So, you're a bit confused about JSON in Python. You think it looks like a dictionary, and you're wondering how you can convert a JSON string into a dictionary structure. No worries, I got you covered! π
Let's dive into the steps of converting a JSON string into a dictionary in Python.
Step 1: Import the Required Modules π
To work with JSON in Python, we need to import the json
module. So, make sure you have it installed.
import json
Step 2: Load the JSON String π₯
The first thing we need to do is load the JSON string. We can use the json.loads()
method to accomplish this.
json_string = '''
{
"glossary":
{
"title": "example glossary",
"GlossDiv":
{
"title": "S",
"GlossList":
{
"GlossEntry":
{
"ID": "SGML",
"SortAs": "SGML",
"GlossTerm": "Standard Generalized Markup Language",
"Acronym": "SGML",
"Abbrev": "ISO 8879:1986",
"GlossDef":
{
"para": "A meta-markup language, used to create markup languages such as DocBook.",
"GlossSeeAlso": ["GML", "XML"]
},
"GlossSee": "markup"
}
}
}
}
}
'''
data = json.loads(json_string)
Here, we've assigned the JSON string to the json_string
variable. Then, we use json.loads()
to load the string into the data
variable as a dictionary.
Step 3: Access the Desired Value π
Now that we have our JSON string converted to a dictionary, we can easily access its values. In your case, you mentioned wanting to obtain the value for the "title"
key.
print(data["glossary"]["title"])
When you run the above code, you'll get the output: "example glossary"
. Amazing! π
Quick Recap π
To convert a JSON string to a dictionary in Python:
Import the
json
moduleLoad the JSON string using
json.loads()
Access the desired value using dictionary indexing
Take the Next Step! πΆββοΈ
Now that you know how to convert a JSON string to a dictionary, you can take your Python skills to the next level. Try working with more complex JSON structures or explore other features of the json
module.
Remember, practice makes perfect! So keep challenging yourself and have fun with Python and JSON! π€
If you found this guide helpful, be sure to share it with your friends and fellow Python enthusiasts. And don't forget to leave a comment below sharing your thoughts or any questions you may have. Happy coding! π»β¨