Convert hex string to integer in Python
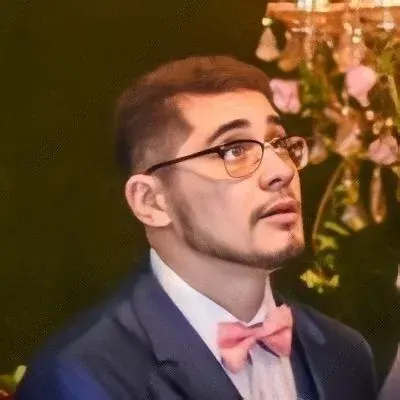
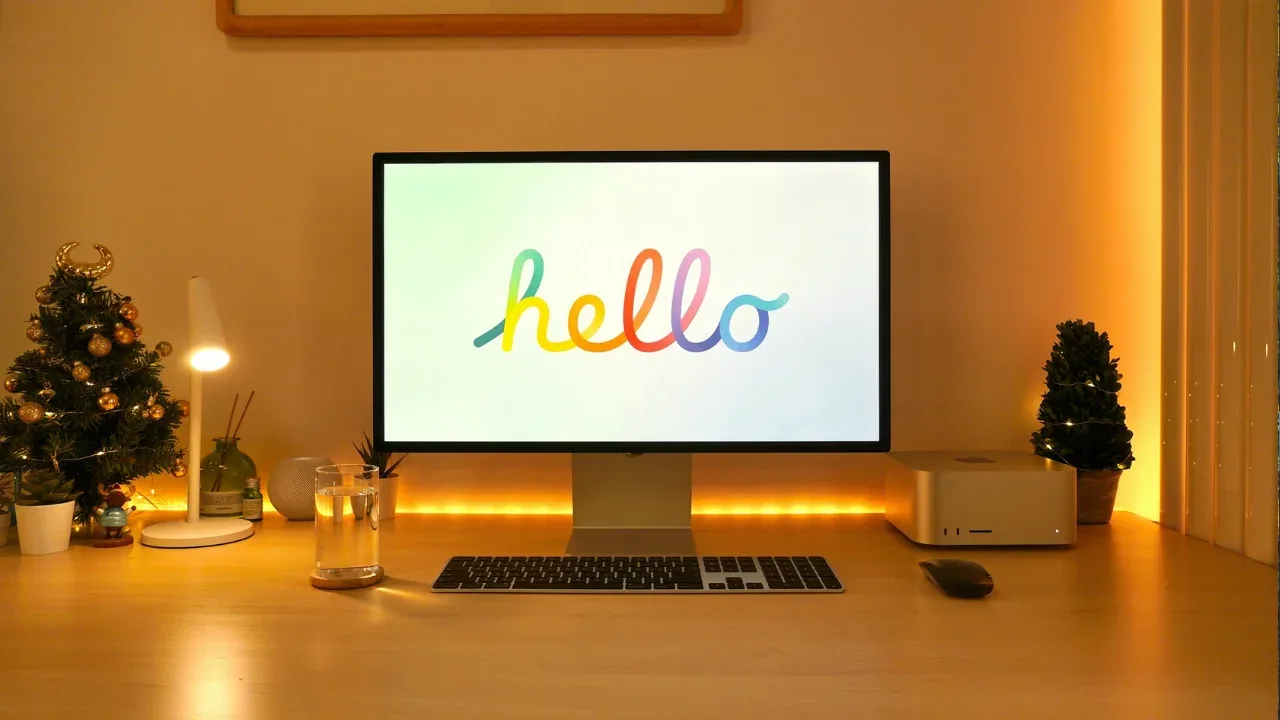
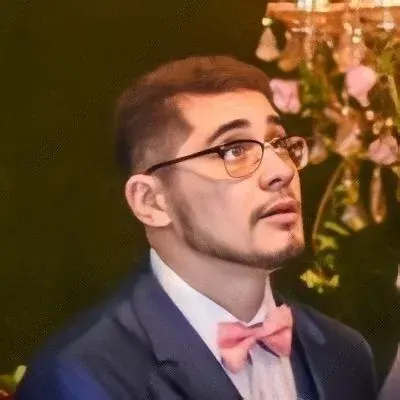
Converting Hex String to Integer in Python: Unraveling the Magic 🧙♀️✨
So, you're faced with the puzzling task of converting a hex string into an integer using Python? Fear not, young Padawan! In this guide, we'll unveil the mystery behind this conversion process and equip you with the tools to handle it like a pro. 🚀
The Conundrum: How to Convert a Hex String to an Integer? 🤔
Let's start by understanding the problem at hand. You have a hex string, such as "0xffff" or "ffff", and you need to convert it into its corresponding decimal integer representation. Imagine transforming "0xffff" into its numerical equivalent, which should be 65535.
Solutions, Step by Step 🔧
Solution 1: The int() Function 🏗️
The simplest way to tackle this problem is by using the built-in int()
function in Python. The int()
function serves as your secret weapon, effortlessly converting the hex string to an integer. Here's a quick demonstration:
hex_string = "0xffff"
decimal_integer = int(hex_string, 16)
print(decimal_integer) # Output: 65535
In the above example, we pass the hex_string
as the first argument to the int()
function. The second argument, 16
, indicates that the string is in base 16 (hexadecimal format). By specifying the base, we ensure that our conversion is accurate, resulting in the expected output.
Solution 2: String Methods + int() 🧩
If your hex string starts with "0x", you can also remove this prefix before converting it to an integer. This can be achieved using Python's string methods, such as lstrip()
or slicing.
hex_string = "0xffff"
hex_string_without_prefix = hex_string.lstrip("0x")
decimal_integer = int(hex_string_without_prefix, 16)
print(decimal_integer) # Output: 65535
The lstrip("0x")
method strips the leading "0x" from the string, leaving only the hex digits. This modified hex string is then passed to the int()
function for conversion.
Time to Level Up! 💪
By now, you should have become a pro at converting hex strings to integers! But don't stop there, my friend. Take it a step further, experiment, and apply this newfound knowledge to solve more challenging problems.
💡 Pro Tips:
Ensure that your hex string is in the correct format before converting it. "0xffff" and "ffff" are valid, but "x0ffff" is not.
Python's
int()
function also supports converting from other number bases (e.g., binary, octal) by appropriately setting the second argument.
Let's Connect! 🌐
Now that you've mastered the art of converting hex strings to integers, why not share your newfound wisdom? Connect with us on social media and let us know how you plan to use this handy skill. Share your code snippets or ask any questions you may have! Together, we'll unlock the potential of Python's magical powers!
Keep coding! 💻✨