Convert Django Model object to dict with all of the fields intact
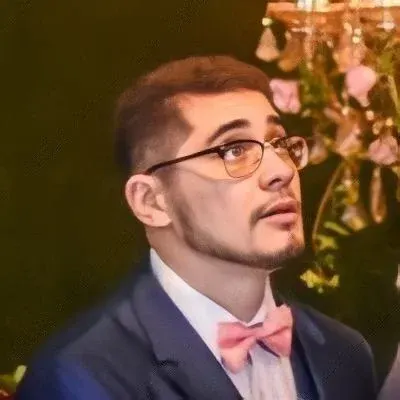
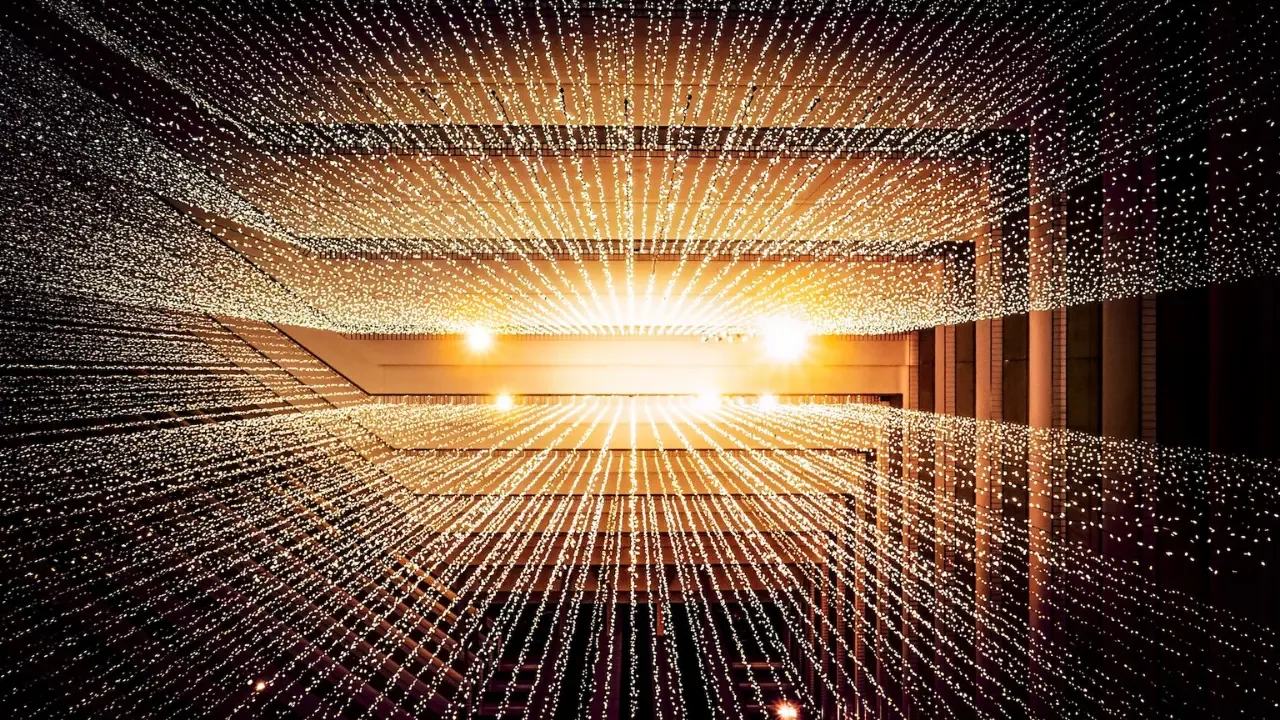
Convert Django Model object to dict with all of the fields intact: A Complete Guide
š Do you ever find yourself needing to convert a Django Model object to a dictionary while still preserving all of its fields? If so, you're in the right place! In this blog post, we will address this common question and provide easy solutions to help you achieve this conversion effortlessly.
š## Background: Understanding the Challenge
Let's take a moment to understand the problem better. The goal is to convert a Django Model object to a dictionary while retaining all of its fields, including foreign keys and fields with the editable=False
attribute. This can be tricky because Django's default to_dict()
method does not include foreign keys, and it excludes fields with editable=False
.
š§©## Problem: Converting a Django Model Object to a Full Dictionary
Consider the following Django model as an example:
from django.db import models
class OtherModel(models.Model):
pass
class SomeModel(models.Model):
normal_value = models.IntegerField()
readonly_value = models.IntegerField(editable=False)
auto_now_add = models.DateTimeField(auto_now_add=True)
foreign_key = models.ForeignKey(OtherModel, related_name="ref1")
many_to_many = models.ManyToManyField(OtherModel, related_name="ref2")
Suppose, after creating and saving instances of SomeModel
, you want to convert them to a dictionary while preserving all of their fields intact. For instance, you desire a dictionary like this:
{
'auto_now_add': datetime.datetime(2015, 3, 16, 21, 34, 14, 926738, tzinfo=<UTC>),
'foreign_key': 1,
'id': 1,
'many_to_many': [1],
'normal_value': 1,
'readonly_value': 2
}
š”## Solution: Overcoming the Challenge
To convert a Django Model object to a complete dictionary, including all fields and foreign keys, we can use the following approach:
def model_to_dict(model_object):
# Create the initial dictionary with basic fields
model_dict = model_object.__dict__.copy()
# Add foreign key fields
for field in model_object._meta.fields:
if isinstance(field, models.ForeignKey):
fk_value = getattr(model_object, field.name)
model_dict[field.name] = fk_value.pk if fk_value else None
# Add many-to-many fields
for field in model_object._meta.many_to_many:
m2m_values = getattr(model_object, field.name).values_list('pk', flat=True)
model_dict[field.name] = list(m2m_values)
# Remove unnecessary fields
model_dict.pop('_state', None)
return model_dict
This function, model_to_dict()
, takes a Django Model object as an argument and returns the corresponding dictionary with all fields intact. It works by:
Creating an initial dictionary with all the basic fields using the
__dict__
property of the model object.Looping through the fields of the model object and adding foreign key fields along with their primary key values.
Handling many-to-many fields by retrieving their primary key values as a list.
Removing the unnecessary
_state
field from the dictionary.
š## Putting it Into Action
Now, let's use the model_to_dict()
function to convert our SomeModel
instance to a complete dictionary:
other_model = OtherModel()
other_model.save()
instance = SomeModel()
instance.normal_value = 1
instance.readonly_value = 2
instance.foreign_key = other_model
instance.save()
instance.many_to_many.add(other_model)
instance.save()
# Convert instance to a dictionary
instance_dict = model_to_dict(instance)
After executing the above code, the instance_dict
variable will contain the desired dictionary with all fields intact.
š## Wrap Up and Call-to-Action
Converting a Django Model object to a dictionary with all fields, including foreign keys and fields with editable=False
, is now within your reach. By using the model_to_dict()
function provided in this guide, you can effortlessly achieve this conversion.
š But wait, there's more! Share your thoughts and experiences. Have you encountered any challenges or found alternative solutions? Join the conversation by leaving a comment below.
š Now it's your turn: try out the model_to_dict()
function in your own Django projects and let us know how it works for you. Happy coding!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
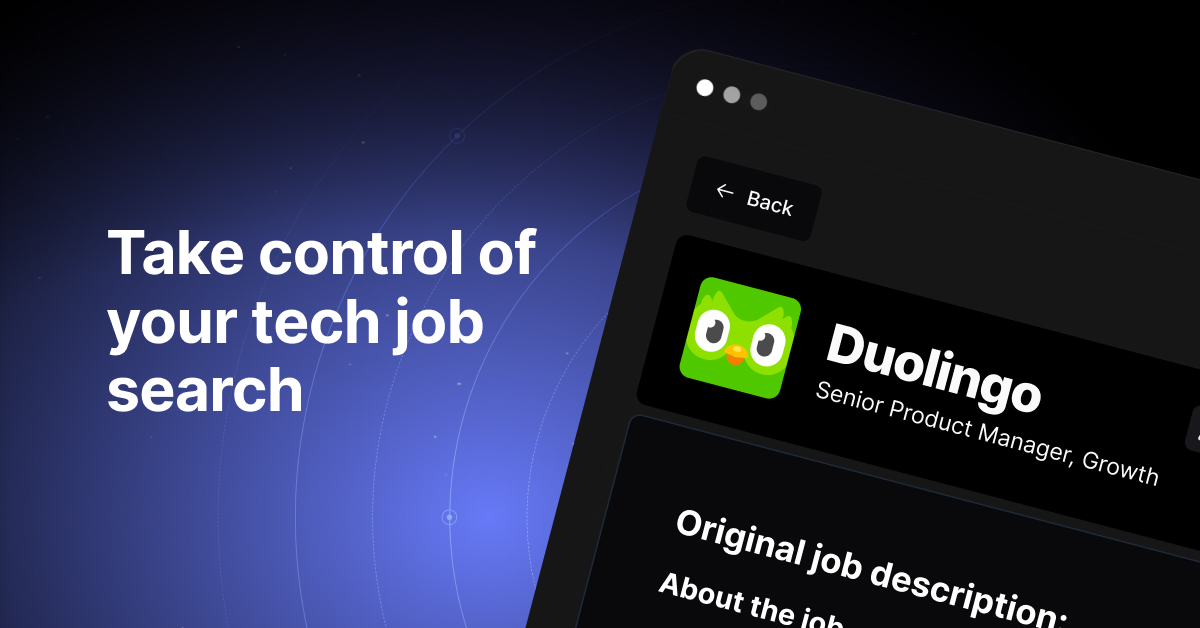