Convert DataFrame column type from string to datetime
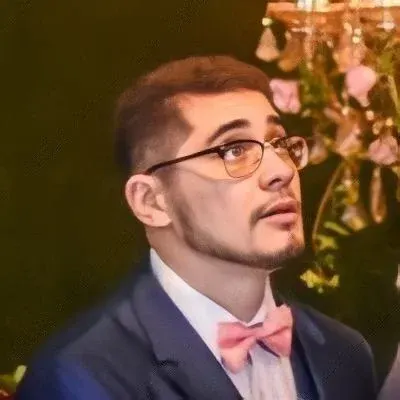
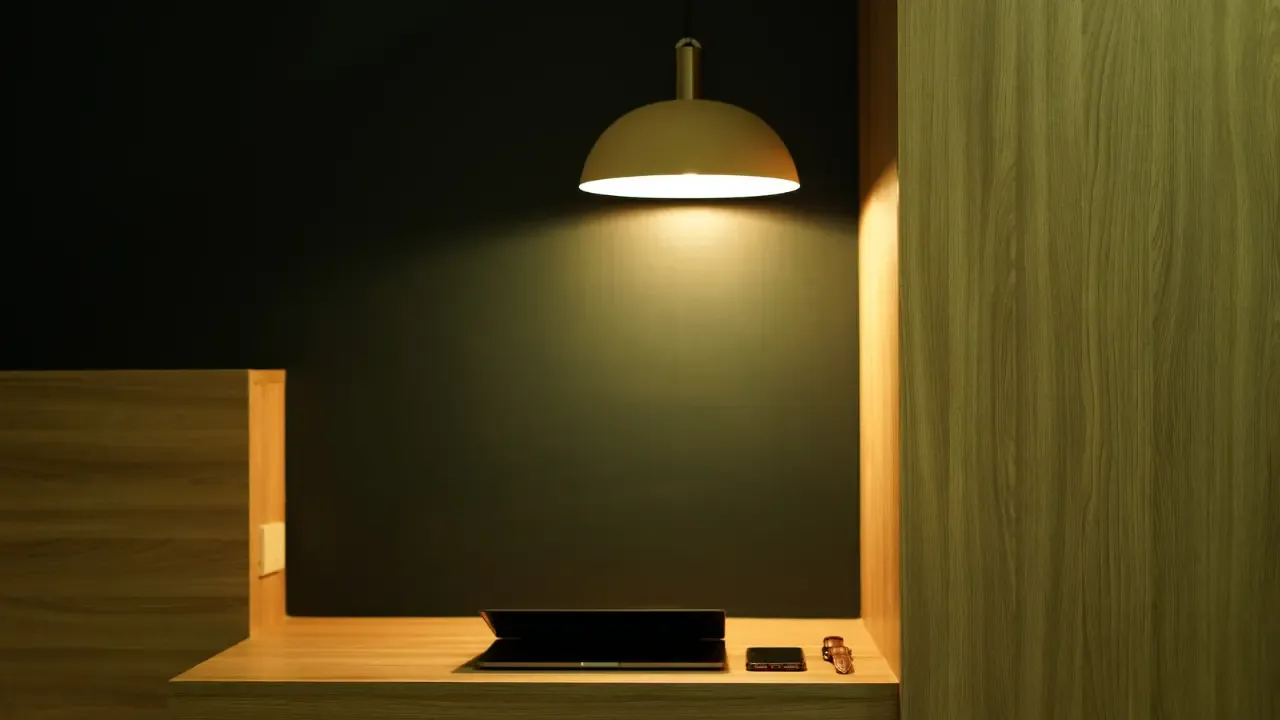
Convert DataFrame Column Type from String to Datetime: The Easy Way! 😎📅
You wouldn't believe how simple it is to convert a DataFrame column from string to datetime! Let me show you how in this step-by-step guide! 🚀🔥
The Problem 😰
So, you have a DataFrame with a column full of dates represented as strings in the dd/mm/yyyy format. But, for some reason, you need to work with this column as actual datetime data. Maybe you want to sort the DataFrame based on dates or perform time-based calculations. Whatever the reason, you're now stuck wondering how to convert this column into the datetime dtype. Don't worry, I got you covered! 😉
The Solution 💡
Thanks to the power of the Pandas library, converting your DataFrame column from string to datetime is just a couple of lines away! Here's what you need to do:
import pandas as pd
df['your_column_name'] = pd.to_datetime(df['your_column_name'], format='%d/%m/%Y')
Let's break this down together, shall we? Here's what each part of the code does:
First, we import the Pandas library and make it accessible through the
pd
alias. (Because we're cool like that! 😎)Then, we apply the
pd.to_datetime()
function to the desired column:df['your_column_name']
. This function performs the actual conversion from string to datetime.We provide the
format
parameter to specify the date format of your string. In our case, it's dd/mm/yyyy, so we useformat='%d/%m/%Y'
. If your format differs, make sure to adjust it accordingly.Finally, we assign the converted column back to the original column in the DataFrame using
df['your_column_name'] =
.
That's it! 🎉 Your column is now transformed into a glorious datetime dtype, ready for all your time-based manipulations!
Common Issues and Troubleshooting 🚧🛠️
Although the solution is pretty straightforward, you might stumble upon a few common issues while converting your DataFrame column to datetime. Let's address some of these potential problems:
ValueError: time data 'your_date_string' does not match format - This error occurs when your date string doesn't match the specified format. Double-check your date format and ensure it matches the actual strings in your column. Also, make sure that the
%d
represents the day,%m
represents the month, and%Y
represents the year correctly.TypeError: Argument 'arg' has incorrect type - This error can occur if your DataFrame column contains non-string or NaN (null) values. Ensure that your column only contains strings representing dates in the expected format.
Date format is not consistent across the column - If your DataFrame column contains multiple date formats, Pandas might raise a
ValueError
. In this case, you'll need to clean and format your column before converting it to datetime. Using string manipulation functions, regular expressions, or Pandas' built-in functions likestr.replace()
can help you achieve consistency in your date column.
Remember, practice makes perfect! Feel free to experiment with different examples and datasets to become a true datetime conversion ninja! 💪
Have Fun with Your Datetime Column! 🎉
Now that you know how to effortlessly convert your DataFrame column from string to datetime, go ahead and apply your newfound knowledge! Sort your DataFrame based on dates, calculate time differences, or plot some cool time-series visualizations. The possibilities are endless! 😍
Don't forget to comment below about your experience or if you faced any additional challenges. Let's learn from each other and geek out together! 🤓💬
So, what are you waiting for? Dive into the world of datetime data conversion and let the possibilities unfold! Happy coding! 😄💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
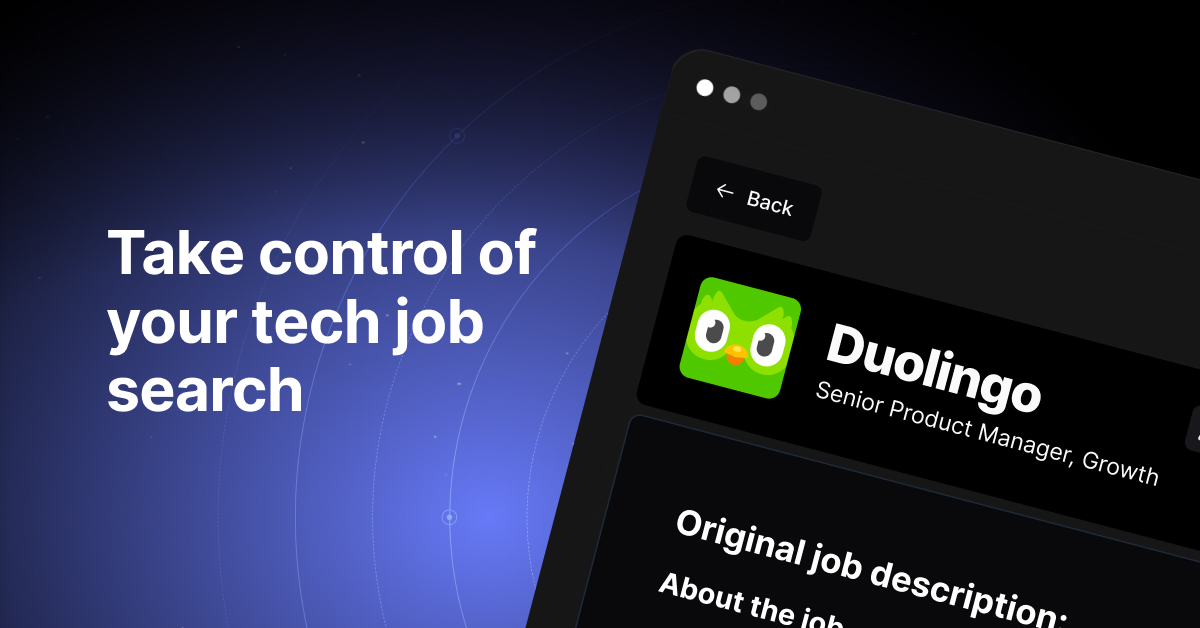