Convert columns to string in Pandas
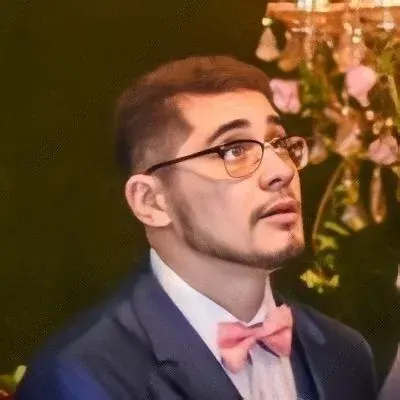
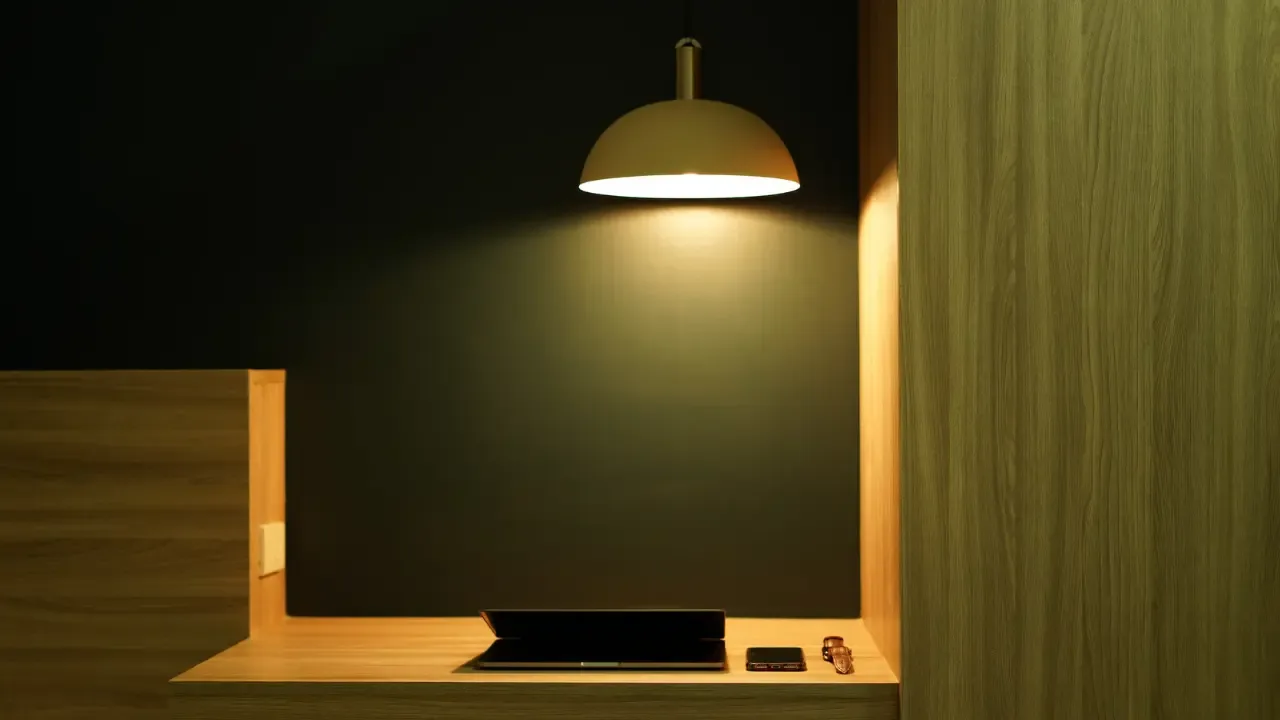
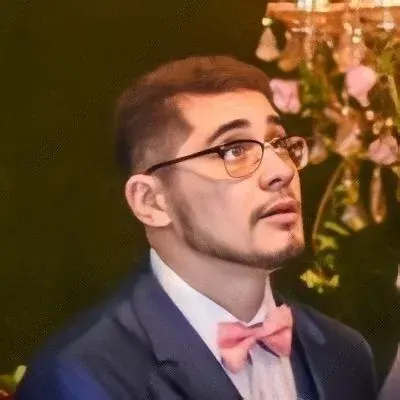
Convert Columns to String in Pandas: A Guide πΌπͺ
Are you facing the challenge of converting columns to strings in Pandas? Look no further! In this guide, we'll walk you through the common issues, provide easy solutions, and help you cast those columns as strings like a pro. Let's dive in! π
The Challenge: Converting Columns to Strings
Consider the following scenario: you have a DataFrame obtained from a SQL query. It looks like this:
ColumnID RespondentCount
0 -1 2
1 3030096843 1
2 3030096845 1
You then pivot the DataFrame using the pivot_table
function, resulting in this:
ColumnID -1 3030096843 3030096845
RespondentCount 2 1 1
[1 rows x 3 columns]
When you convert this pivoted DataFrame into a dictionary using the to_dict('records')[0]
method, you encounter an issue. The keys corresponding to the column IDs, such as 3030096843
and 3030096845
, are converted to integers instead of strings. However, you want those column IDs to be cast as strings, while keeping -1
as an integer:
{'3030096843': 1, '3030096845': 1, -1: 2}
The Solution: Casting Columns as Strings
To achieve the desired result, you can apply a simple transformation to your DataFrame using the astype
method. Here's an example:
total_data.columns = total_data.columns.astype(str)
By applying this code to your pivoted DataFrame, you convert all column labels to strings. Now, when you convert the DataFrame into a dictionary, you will get the expected output:
{'3030096843': 1, '3030096845': 1, -1: 2}
π It's Your Turn!
Now that you know how to convert columns to strings in Pandas, give it a try with your own data! Share your results with us and let us know if you encountered any issues or have further questions.
If you found this guide helpful, don't forget to share it with your fellow Pandas enthusiasts. Happy coding! ππΌ
Sources: