Convert columns into rows with Pandas
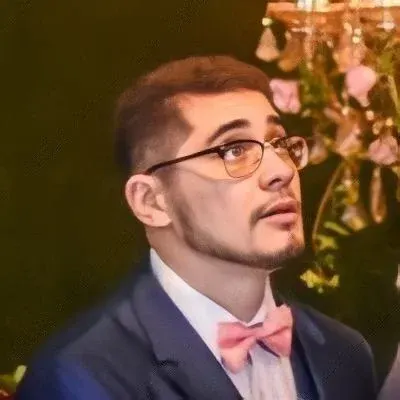
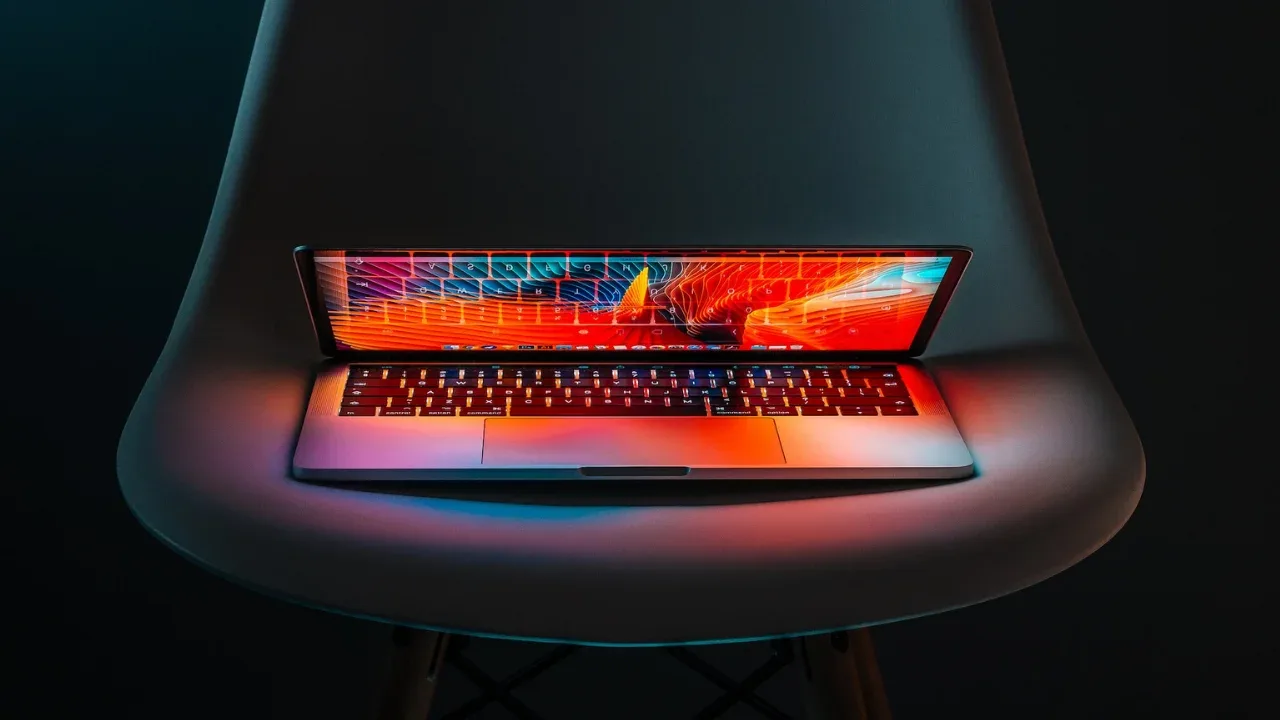
Converting Columns into Rows with Pandas: A Complete Guide 👩💻📊
So you have a dataset with information by location for different dates, but the dates are currently spread out as column headers. You want to convert these columns into rows to make your data more organized and easier to analyze. No worries, we've got you covered! In this blog post, we will walk you through the process of converting columns into rows using the powerful Python library, Pandas. Let's dive in! 🏊♀️
The Problem: Column-Based Dates 😕
Imagine you have a CSV file and it looks like this:
location name Jan-2010 Feb-2010 March-2010
A "test" 12 20 30
B "foo" 18 20 25
As you can see, the dates are spread across different columns, making it difficult to work with the data effectively. Your goal is to transform the data into the following structure:
location name Date Value
A "test" Jan-2010 12
A "test" Feb-2010 20
A "test" March-2010 30
B "foo" Jan-2010 18
B "foo" Feb-2010 20
B "foo" March-2010 25
Each row now represents a specific date-value pair for a given location. This transformation will allow for better data manipulation and analysis.
The Solution: Unstacking the Columns ✨
To convert columns into rows in Pandas, we need to "unstack" the dates from the column headers. The melt()
and stack()
functions in Pandas will be our trusty companions for this task. Here's a step-by-step solution:
Import the necessary libraries:
import pandas as pd
Load your dataset into a Pandas DataFrame:
df = pd.read_csv('your_dataset.csv')
Identify the columns to be converted into rows (in our case, the date columns):
date_columns = df.columns[2:]
Unstack the date columns by melting them into rows and renaming the resulting columns:
df = df.melt(id_vars=['location', 'name'], value_vars=date_columns, var_name='Date', value_name='Value')
Optionally, sort the DataFrame by location and date:
df = df.sort_values(by=['location', 'Date'])
Enjoy your new transformed dataset! 🎉
Now, your DataFrame should resemble the desired structure:
location name Date Value
A "test" Jan-2010 12
A "test" Feb-2010 20
A "test" March-2010 30
B "foo" Jan-2010 18
B "foo" Feb-2010 20
B "foo" March-2010 25
Dealing with Unknown Number of Dates ❓
You mentioned that you don't know how many dates are in the column, but they will always start after the 'name' column. Not to worry, Pandas has you covered! By using the .iloc
indexer, you can select columns by their integer position instead of their name. So, instead of manually identifying the date columns, you can modify step 3 to select all remaining columns after the 'name' column:
date_columns = df.columns[df.columns.get_loc('name')+1:]
This approach ensures that all date columns after the 'name' column will be considered for the unstacking process, regardless of their number or specific names.
Call-to-Action: Unleash the Power of Pandas! 🚀
Now that you know how to convert columns into rows with Pandas, you can unlock the full potential of your data! Go ahead and give it a try, and don't be afraid to experiment with different datasets and scenarios.
If you found this guide helpful, don't forget to share it with your friends and colleagues who might also benefit from it.
Have any questions or facing any issues? Drop a comment below, and we'll be more than happy to assist you! Happy coding! 💻😄
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
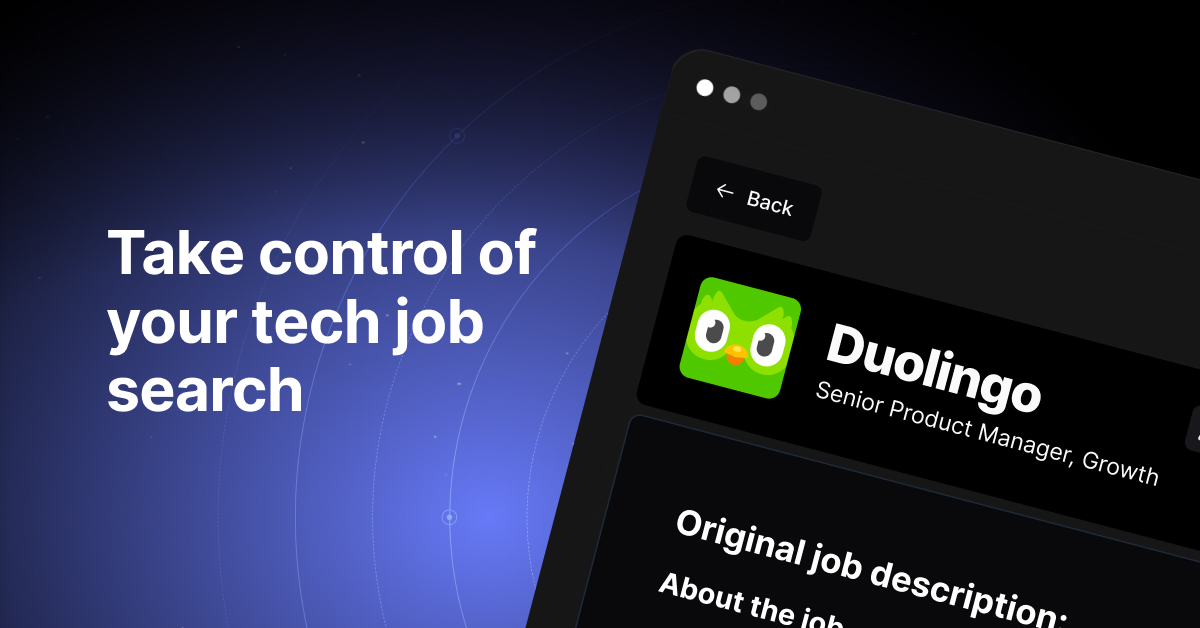