Convert bytes to a string in Python 3
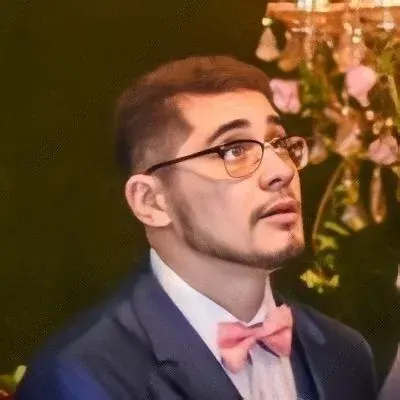
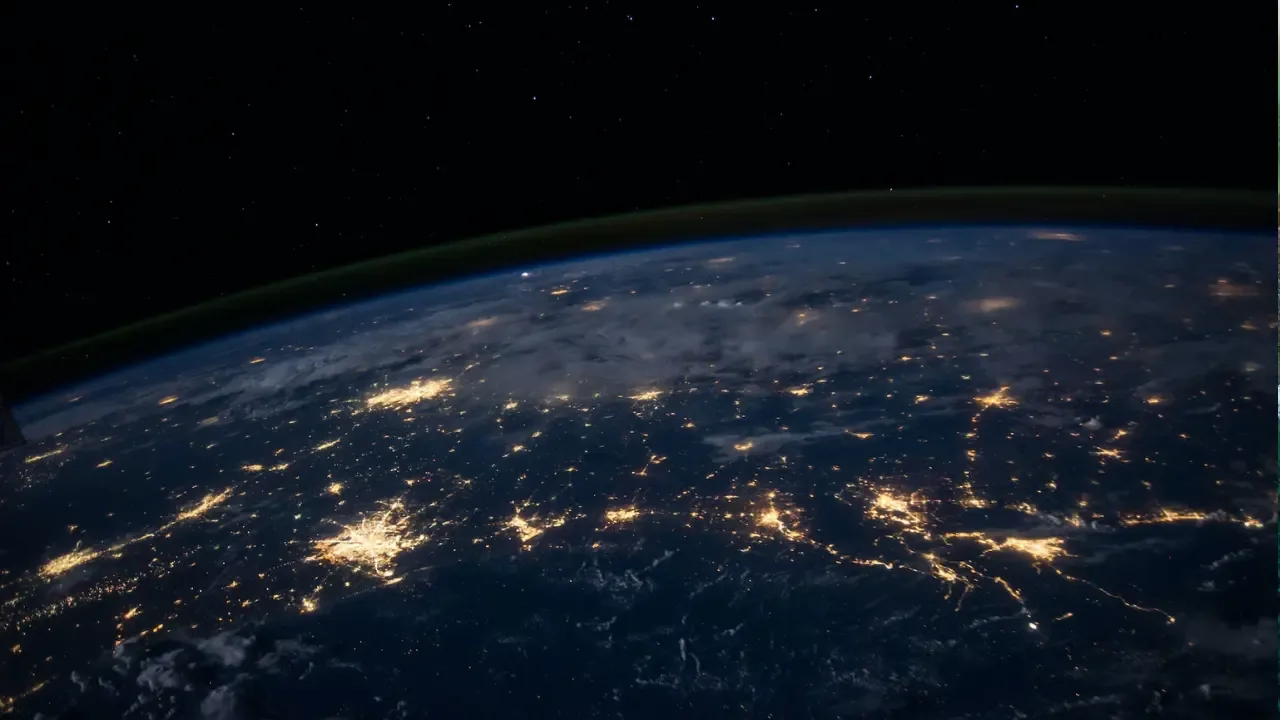
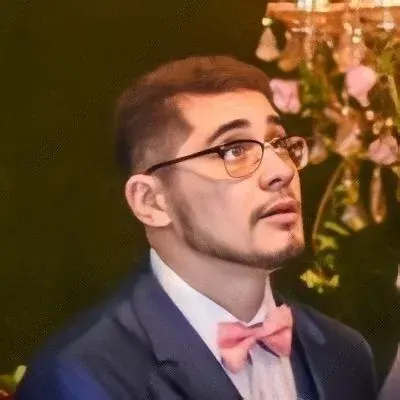
🔍 Converting Bytes to a String in Python 3: A Complete Guide 🔍
Are you facing the challenge of converting a bytes
object to a str
in Python 3? Don't worry, we've got you covered! In this blog post, we will explore common issues related to this conversion and provide easy solutions that will make your life easier. So, let's dive right in and decode those bytes into a readable string! 💪
🎯 The Challenge
Let's start by understanding the problem at hand. Imagine you capture the output of an external program and store it in a bytes
object. In our example, we have the output of the ls -l
command:
>>> from subprocess import *
>>> stdout = Popen(['ls', '-l'], stdout=PIPE).communicate()[0]
>>> stdout
b'total 0\n-rw-rw-r-- 1 thomas thomas 0 Mar 3 07:03 file1\n-rw-rw-r-- 1 thomas thomas 0 Mar 3 07:03 file2\n'
Now, you want to convert this bytes
object into a regular Python string, so that you can print it out nicely like this:
>>> print(stdout)
-rw-rw-r-- 1 thomas thomas 0 Mar 3 07:03 file1
-rw-rw-r-- 1 thomas thomas 0 Mar 3 07:03 file2
🌟 The Solution
To decode the bytes
object and convert it into a string, Python provides a built-in method called decode()
. We can use this method to specify the encoding of the bytes and obtain a readable string representation.
In our case, we assume that the output is encoded in UTF-8. Here's how we can convert the bytes
object to a str
:
decoded_str = stdout.decode('utf-8')
🔥 An Extra Tip
If you are unsure about the encoding of the bytes
object or want to handle different encodings, you can use the chardet
library. This library automatically detects the encoding of a given bytes
object. To use chardet
, you need to install it first using pip
:
pip install chardet
Once installed, you can easily detect the encoding and decode the bytes like this:
import chardet
result = chardet.detect(stdout)
encoding = result['encoding']
decoded_str = stdout.decode(encoding)
🔔 Call to Action
Now that you know how to convert bytes
to a string in Python 3, go give it a try! Experiment with different encodings and make your code even more robust. Also, be sure to check out our other blog posts and stay tuned for more exciting Python tips and tricks. Happy coding! 🚀
🔗 References
Note: The information and examples presented in this blog post are for educational purposes only. Please refer to the official Python documentation and other reliable sources for detailed information and guidance.