Convert a String representation of a Dictionary to a dictionary
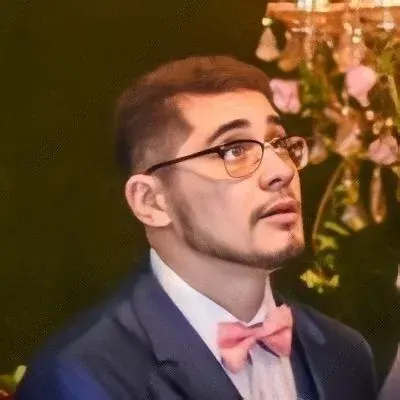
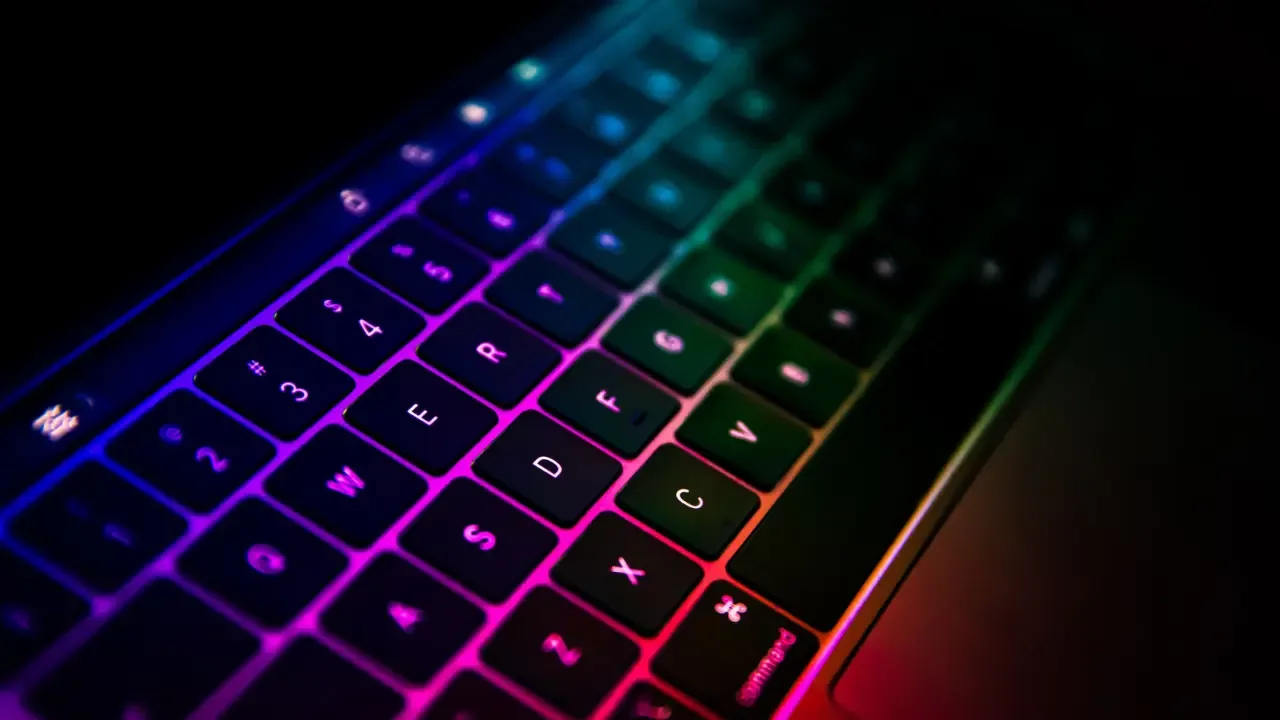
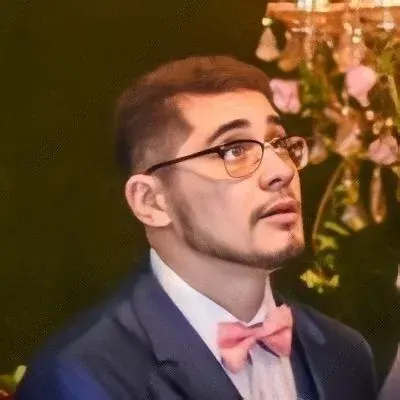
Title: Converting a String Representation of a Dictionary into a dict: No Eval Needed!
Intro
š Hey there tech enthusiasts! Welcome to another exciting blog post where we explore some awesome tech hacks. Today, we'll dive into the world of converting a string representation of a dictionary into an actual dictionary. šš
The Problem
š¤ So, you've come across a string that looks like a dictionary but is not quite a dictionary. Maybe it came from an API response, a text file, or even a co-worker's crafted string. You might be tempted to use the eval
function to convert it, but don't fret! We have an alternative solution that doesn't involve modifying anyone's code. š
āāļø
The string you're dealing with looks like this:
s = "{'muffin' : 'lolz', 'foo' : 'kitty'}"
The Solution
š Let's jump straight into the solution, shall we? We'll be using the ast.literal_eval
function, which safely evaluates a string containing a Python literal. It's perfect for this scenario!
Here's the code snippet you can use to convert the string into a dictionary:
import ast
def str_to_dict(s):
return ast.literal_eval(s)
# Example usage
s = "{'muffin' : 'lolz', 'foo' : 'kitty'}"
result = str_to_dict(s)
print(result)
That's it! With just a few lines of code, you'll have your desired dictionary without using eval
. šŖ
But, How Does It Work?
š Let's peek behind the curtain and understand how ast.literal_eval
works. It safely evaluates a single expression, rather than an entire program like eval
, making it less risky.
It utilizes the ast
(Abstract Syntax Trees) module to ensure that only literals, such as strings, numbers, tuples, lists, booleans, and dictionaries, can be evaluated. In the case of our string representation of a dictionary, it safely converts it to an actual dictionary.
Time to Take Control!
š§ Imagine the possibilities now that you know how to convert a string representation of a dictionary to a real dictionary. You're ready to tackle those messy input sources without modifying other code. Take control of your coding journey! šŖ
Conclusion
š There you have it! You've learned the alternative solution for converting a string representation of a dictionary into a dictionary in Python. Say goodbye to risky eval
functions and confidently parse those strings with ast.literal_eval
. It's safe, reliable, and efficient. š
š” So, what are you waiting for? Give it a try and let us know your thoughts in the comments section below. Have you come across any other cool alternatives? Share them with the community! Let's learn and grow together. Happy coding! ššØāš»š©āš»