Convert a python dict to a string and back
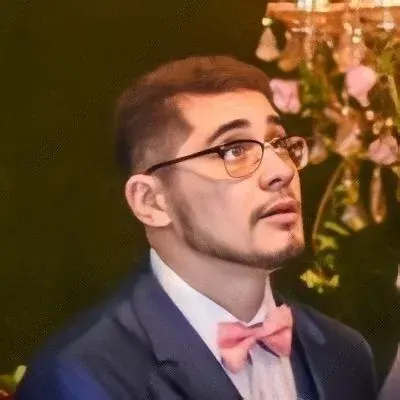
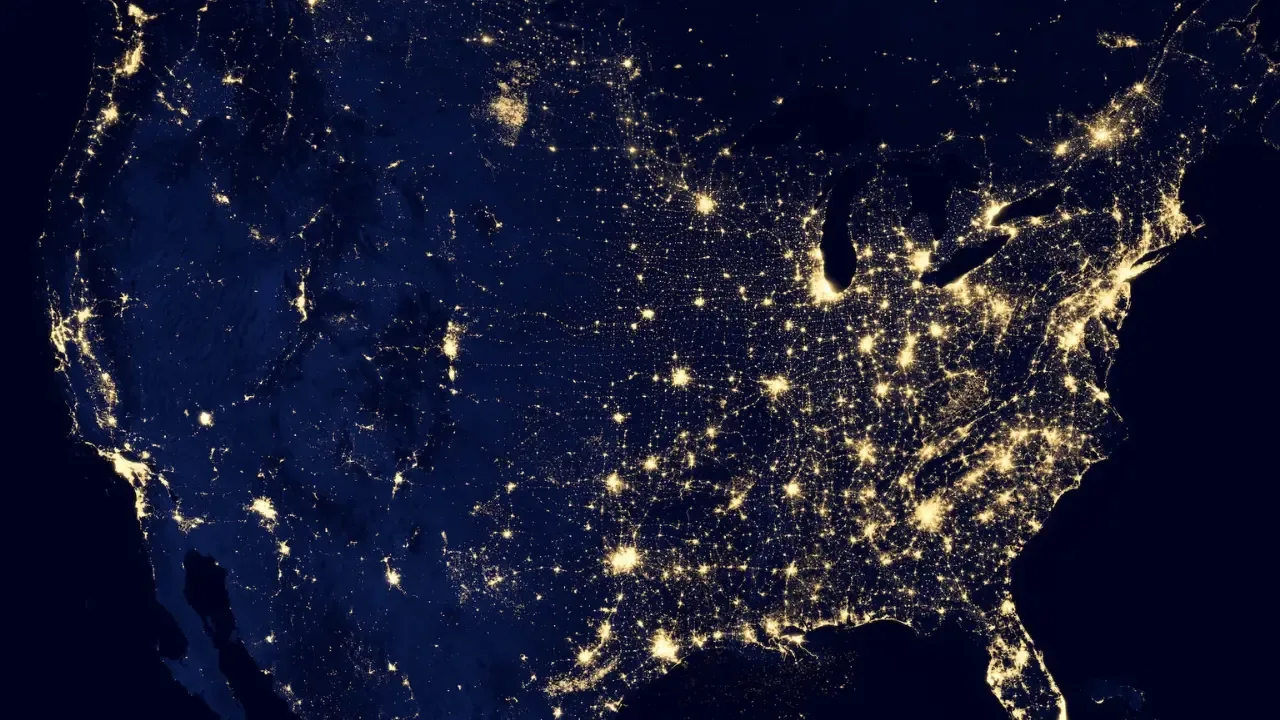
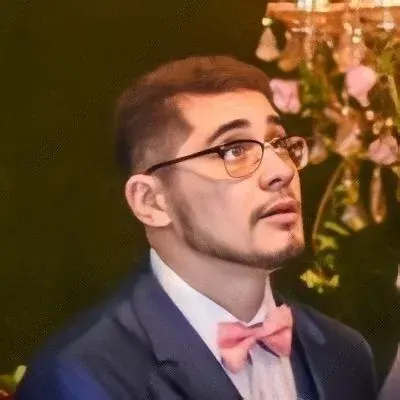
Converting a Python Dict to a String and Back: The Ultimate Guide 😎🔥
Are you working on a program that needs to store data in a dictionary object and then load it back into the dictionary when the program is run again? Look no further! In this guide, we will explore how to convert a Python dict to a string and back, including support for dictionaries containing other dictionaries. Let's dive right into it! 💪
The Problem: Storing and Loading Dicts 🧐
Imagine you have a program that uses a dictionary object to store important data. But, at some point, you need to save this data to a file so it can be retrieved later. How can you convert the dictionary to a string representation that can be written to a file and loaded back into the program as a dictionary object? This is the challenge we are about to solve! 😉
Solution 1: JSON Serialization 🌐
One popular and straightforward solution is to use JSON serialization. The json
module in Python provides functions to convert Python objects to JSON strings and vice versa.
Here's how you can implement it:
import json
# Convert dict to JSON string
dict_data = {'key': 'value'}
json_string = json.dumps(dict_data)
# Convert JSON string back to dict
dict_back = json.loads(json_string)
By using json.dumps()
function, you can convert the dictionary to a JSON string. And json.loads()
allows you to load the JSON string as a dictionary object again. Easy peasy! 😄
Solution 2: Pickle Serialization 🥒🧅
If you want more flexibility and support for complex objects, you may consider using the pickle
module. Pickle serialization allows you to handle more than just JSON-serializable objects.
Let's see an example:
import pickle
# Convert dict to string using pickle
dict_data = {'key': 'value'}
pickle_string = pickle.dumps(dict_data)
# Convert pickle string back to dict
dict_back = pickle.loads(pickle_string)
Using pickle.dumps()
, you can convert the dictionary to a string representation. Then, pickle.loads()
enables you to load the string back into a dictionary object.
Bonus Tip: Handling Dictionaries Containing Dictionaries 📦
What if your dictionary contains other nested dictionaries? How can you handle this scenario?
Both JSON and Pickle serialization support dictionaries containing other dictionaries. All you need to do is ensure that the nested dictionaries are JSON-serializable or compatible with Pickle serialization.
Conclusion and Call-to-Action 🏁📣
In this guide, we explored two popular solutions for converting a Python dict to a string and back: JSON and Pickle serialization. Depending on your needs, you can choose either one. Just ensure that the objects in your dictionary are JSON-serializable or compatible with Pickle.
Now, it's your turn to give it a try! Experiment with different dictionaries, nested dictionaries, and complex objects. Which solution works best for your program? Share your experience and any further questions or challenges in the comments below! Let's convert those dicts! 🚀🔢
Remember: Always analyze the security implications of loading and executing strings as code. Be cautious when loading untrusted data into your program.
Happy coding! 😊👩💻👨💻