Convert a Pandas DataFrame to a dictionary
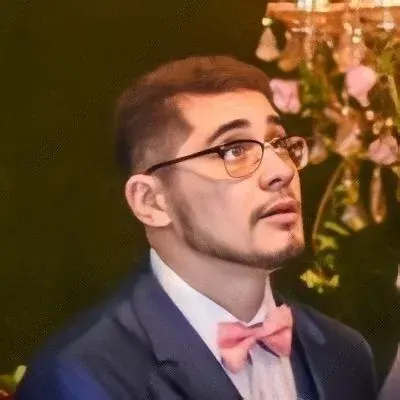
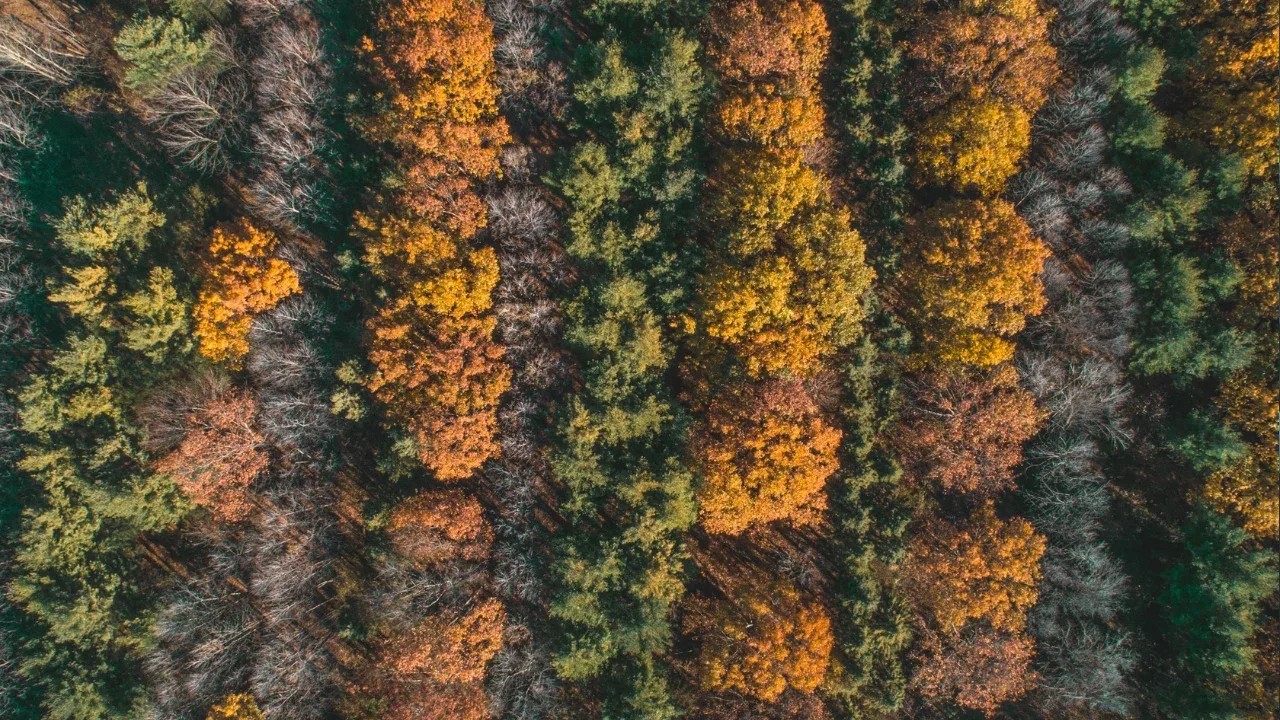
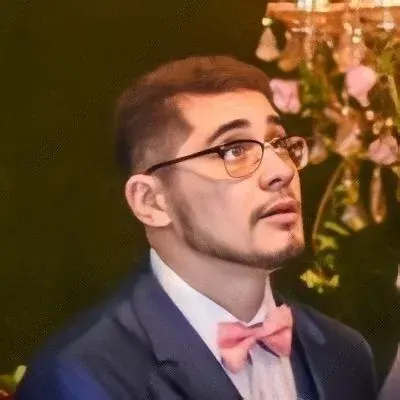
Converting a Pandas DataFrame to a Dictionary πΌπβ‘οΈπ
Have you ever found yourself needing to convert a Pandas DataFrame to a dictionary in Python? It's a common task that can come up in various data analysis and manipulation scenarios. In this blog post, we'll address how to convert a DataFrame to a dictionary, specifically in a way that the elements of the first column become the keys, and the elements of the other columns in the same row become the corresponding values.
The Challenge π€
Let's start by taking a look at the example DataFrame we want to convert:
ID A B C
0 p 1 3 2
1 q 4 3 2
2 r 4 0 9
As mentioned, we want to convert this DataFrame into a dictionary where the keys are the elements of the 'ID' column, and the values are lists containing the elements from columns 'A', 'B', and 'C' corresponding to the same row.
The Solution π‘
The good news is that Pandas provides an easy way to accomplish this using the to_dict
method. Here's an overview of the steps involved:
Set the 'ID' column as the index column (optional but recommended for simplicity)
Use the
to_dict
method with theorient
parameter set to'list'
Let's see how this solution looks in code:
import pandas as pd
# Create the DataFrame
df = pd.DataFrame({
'ID': ['p', 'q', 'r'],
'A': [1, 4, 4],
'B': [3, 3, 0],
'C': [2, 2, 9]
})
# Set 'ID' as the index column
df.set_index('ID', inplace=True)
# Convert the DataFrame to a dictionary
dictionary = df.to_dict(orient='list')
print(dictionary)
Running this code will produce the following output:
{'p': [1,3,2], 'q': [4,3,2], 'r': [4,0,9]}
As you can see, we've successfully converted the DataFrame into the desired dictionary format.
Your Turn! πͺ
Now it's your turn to give it a try! If you have a DataFrame that needs to be converted into a dictionary, I encourage you to follow the steps outlined above. Remember to set the appropriate column as the index before using the to_dict
method.
Feel free to use the example DataFrame provided or create your own. Experiment with different scenarios and see how the solution performs with larger datasets or different column types.
Conclusion π
Converting a Pandas DataFrame to a dictionary in Python doesn't have to be a daunting task. With the to_dict
method and a few simple steps, you can achieve the desired result effortlessly.
Remember, setting the index column can help simplify the process, and the orient
parameter in the to_dict
method allows you to control the structure of the resulting dictionary.
I hope you found this guide helpful and that it saved you some valuable time in your data analysis journey! If you have any questions or other interesting use cases for converting DataFrames to dictionaries, feel free to share them in the comments below.
Happy coding! ππβ¨