Constructing pandas DataFrame from values in variables gives "ValueError: If using all scalar values, you must pass an index"
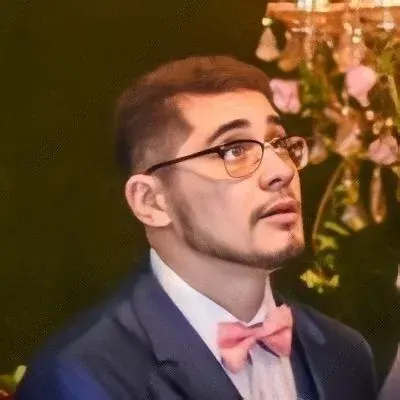
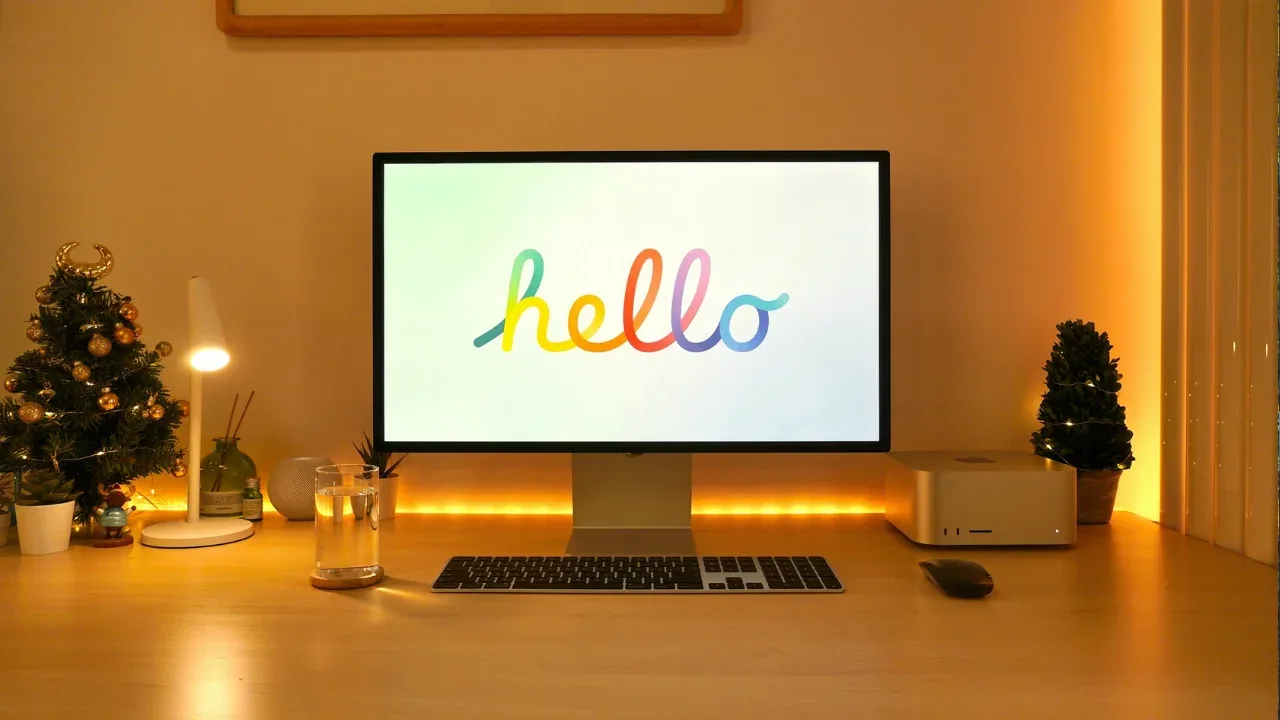
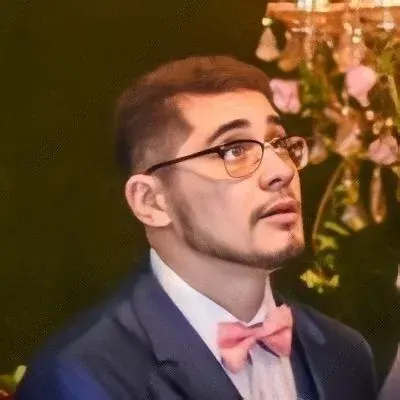
🎉 Title: The KeyError Mystery-Unveiling How to Construct a Pandas DataFrame from Variable Values 🎉
Hey there, fellow data enthusiasts! 😄
Are you struggling to construct a Pandas DataFrame using values stored in variables? Do you despair when you encounter a pesky "ValueError: If using all scalar values, you must pass an index" message? Fear not, for we have the answers you seek! 🙌
Understanding the Problem 🕵️♀️
Picture this: you have two variables, a
and b
, and you want to create a DataFrame from them. You march on, confidently typing:
df2 = pd.DataFrame({'A': a, 'B': b})
🚫 Bam! 🚫 You're greeted with a frustrating "ValueError: If using all scalar values, you must pass an index" message. What went wrong? Let's dive into the depths of this issue. 💡
Demystifying the Error Message 🕵️♂️
The error message you encountered arises when you attempt to create a DataFrame using only scalar values (single values, such as integers) without specifying an index. Pandas requires an index to be supplied alongside scalar values to construct a DataFrame correctly.
Simple Solutions to the Rescue 🦸♀️
Solution 1: Using Lists 📝
A quick and handy workaround is to create lists from your variables and pass them into the DataFrame constructor. Here's the code you should use:
df2 = pd.DataFrame({'A': [a], 'B': [b]})
Wait, what's this sorcery? By enclosing a
and b
in square brackets, we transform them from scalar values into lists. This simple trick ensures Pandas constructs the DataFrame without any complaints! 🪄
Solution 2: Using NumPy 🧮
If you prefer to channel your inner magician using NumPy, fret not! You can utilize NumPy's array
function to create a one-dimensional array, which in turn can be used to easily generate your DataFrame. Here's how:
import numpy as np
df2 = pd.DataFrame({'A': np.array(a), 'B': np.array(b)})
Voilà! You've successfully circumvented the notorious scalar values issue by effortlessly creating a DataFrame from your variables with NumPy's helping hand. 🎩
Unyielding Persistence, Perseverance, and... the Same Error!? 🚧
Some readers may have attempted another potential solution, seeking a workaround by resetting the index. Alas, despair not, for while this approach can be useful in different scenarios, it won't resolve this particular issue. The error message will remain intact.
df2 = (pd.DataFrame({'a':a,'b':b})).reset_index()
The Call-to-Action 🔔
We hope this blog post has provided you with clarity on constructing Pandas DataFrames from variables while avoiding that pesky "ValueError: If using all scalar values, you must pass an index" message.
Have you encountered any other DataFrame construction enigmas? Do you want to learn more about Pandas' hidden secrets? Share your thoughts, experiences, and questions in the comments below! Let's join forces and conquer the data realm together! 👊💥
Stay curious, keep coding, and until next time! 🚀📊