Combining two Series into a DataFrame in pandas
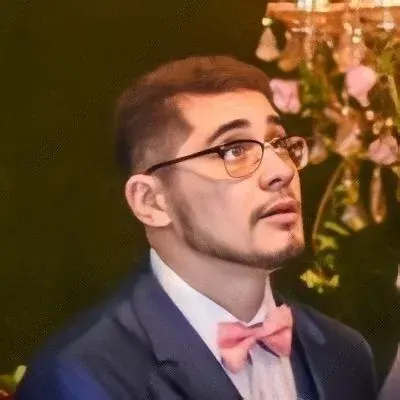
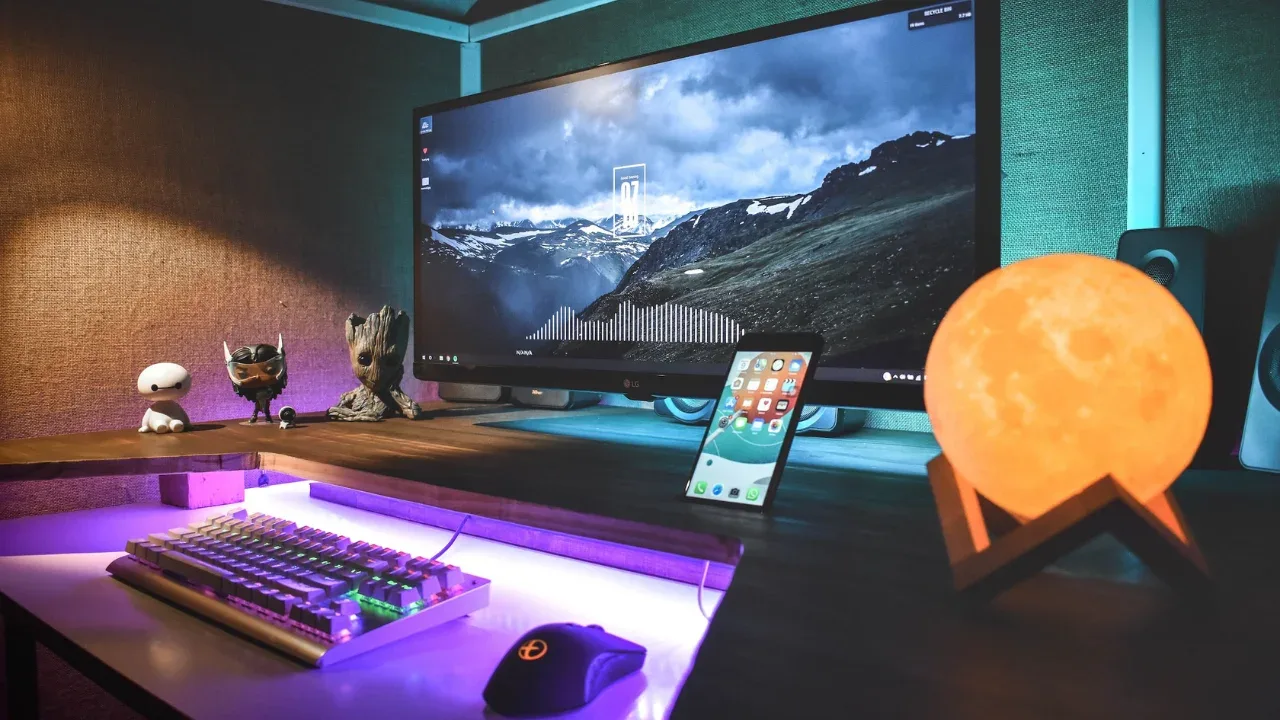
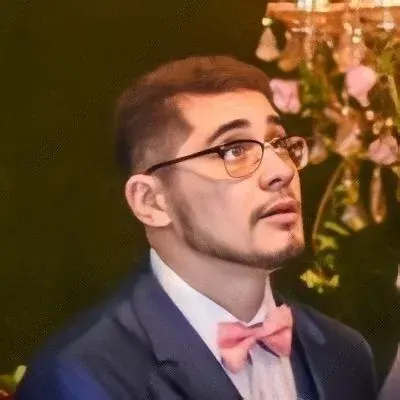
Combining two Series into a DataFrame in pandas: A Guide πΌ
Have you ever found yourself in a situation where you need to combine two Series in pandas into a DataFrame? It's a common need, especially when working with data analysis and manipulation. In this guide, we'll explore the common issues that arise when combining two Series and provide easy solutions to help you tackle this problem like a pro, all while keeping one of the indices as a third column. Let's jump right in!
Understanding the Problem
The first step in solving any problem is understanding it. So, what exactly are we trying to achieve here? We have two Series, s1
and s2
, with non-consecutive indices, and we want to combine them into a DataFrame while preserving one of the indices as a third column. Sounds challenging, right? Fear not! pandas makes it easy for us.
The Solution: pd.concat()
to the Rescue!
To combine two Series into a DataFrame, we can make use of the pd.concat()
function provided by pandas. This function allows us to concatenate objects along a particular axis.
Let's take a look at how we can use pd.concat()
to solve our problem:
import pandas as pd
# Assume we have two Series, s1 and s2, with non-consecutive indices
s1 = pd.Series([10, 20, 30], index=[1, 2, 3])
s2 = pd.Series([40, 50, 60], index=[2, 3, 4])
# Combine s1 and s2 into a DataFrame, preserving one index as a third column
df = pd.concat([s1, s2], axis=1, keys=['s1', 's2'])
# Print the resulting DataFrame
print(df)
π Ta-da! By using pd.concat([s1, s2], axis=1, keys=['s1', 's2'])
, we have successfully combined s1
and s2
into a DataFrame with two columns, where the column names are 's1' and 's2'.
Example: Putting the Solution into Action
To further solidify our understanding, let's walk through an example using actual data. Consider the following scenario:
import pandas as pd
# Sales data for two products: 'Product A' and 'Product B'
sales_product_a = pd.Series([100, 200, 150], index=[1, 2, 3])
sales_product_b = pd.Series([50, 75, 100], index=[2, 3, 4])
# Combining sales data into a DataFrame, preserving one index as a third column
df_sales = pd.concat([sales_product_a, sales_product_b], axis=1, keys=['Product A', 'Product B'])
# Print the resulting DataFrame
print(df_sales)
In this example, we have two Series representing the sales data for 'Product A' and 'Product B'. By using pd.concat()
with the appropriate arguments, we concatenate these Series into a DataFrame called df_sales
. The resulting DataFrame displays the sales data for both products, with the non-consecutive indices '1', '2', '3', and '4', and columns labeled 'Product A' and 'Product B'.
Engage with the Community π
We hope this guide has helped you understand how to combine two Series into a DataFrame in pandas while keeping one of the indices as a third column. Now, it's your turn to put your newly acquired knowledge into action!
βοΈ Share your thoughts and experiences in the comments section below. Have you encountered any issues while combining Series in pandas? How did you solve them? Let's learn from each other and grow together as a community!
π’ If you found this guide helpful, don't forget to share it with your fellow pandas enthusiasts. Spread the knowledge and help others overcome this common challenge!
Happy coding! πΌπ»