Combine two columns of text in pandas dataframe
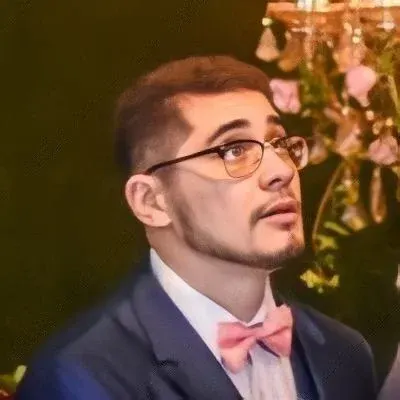
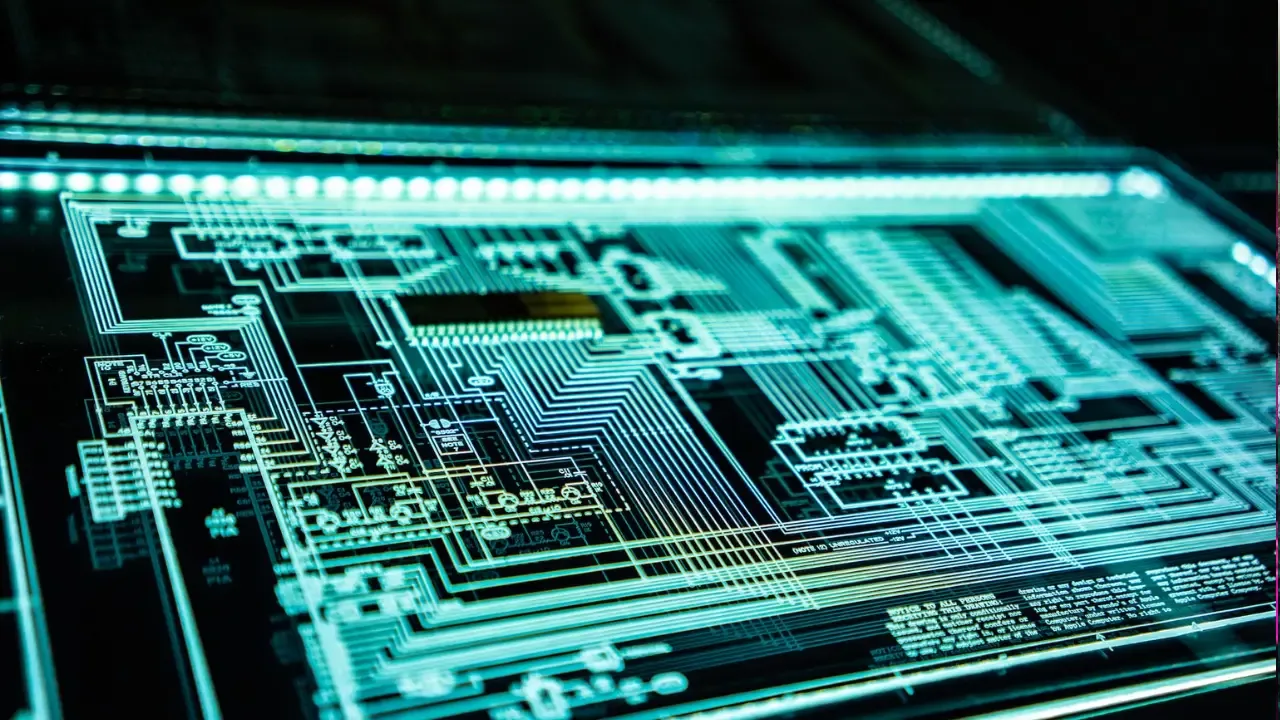
📝 Combine Two Columns of Text in Pandas Dataframe
Do you have a pandas dataframe with two separate columns of text and want to combine them into a single column? Well, you're in luck! In this blog post, we will address this common data manipulation issue and guide you through easy solutions to achieve your desired outcome. 💪
The Scenario
Let's start by setting the context. Imagine that you have a 20 x 4000 dataframe in Python, created using the pandas library. Among the columns, you have one named Year
and another named quarter
. Your goal is to create a new variable called period
that combines the values from these two columns in a specific format.
Here's an example of what you're aiming for: if Year
is equal to 2000
and quarter
is equal to q2
, you want the resulting period
value to be 2000q2
. Sounds simple enough, right? 🤔
Solution Steps
To achieve this, we can follow a straightforward process using pandas. Let's dive into the easy solutions:
First, make sure you have pandas installed in your Python environment. If you haven't done it already, you can install it by running the following command:
pip install pandas
Import the pandas library into your Python script or Jupyter Notebook:
import pandas as pd
Assuming you already have your dataframe loaded into a variable, let's call it
df
, you can add theperiod
column by concatenating theYear
andquarter
columns, with the desired separator in between. Here's how you can do it:df['period'] = df['Year'] + df['quarter']
As a result, the
period
column will be added to your dataframe, combining the values fromYear
andquarter
.
An Example for Clarity
To illustrate the solution, let's consider an example with the following dataframe:
Year quarter
0 2000 q2
1 2001 q3
2 2001 q4
3 2002 q1
After applying the solution, our dataframe will look like this:
Year quarter period
0 2000 q2 2000q2
1 2001 q3 2001q3
2 2001 q4 2001q4
3 2002 q1 2002q1
By leveraging pandas' ability to handle column operations, we successfully combined the Year
and quarter
columns into the newly created period
column in the desired format.
Take Action Now!
Now that you know how to effortlessly combine two columns of text using pandas, why not try it out yourself? 🚀 Update your code or start a new project, apply the outlined steps, and witness the power of pandas in action!
Feel free to share your experience in the comments section below. If you have any questions or face any challenges, our community is here to support you. Let's unleash the full potential of pandas together! 💡💬
Happy coding! 💻😃
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
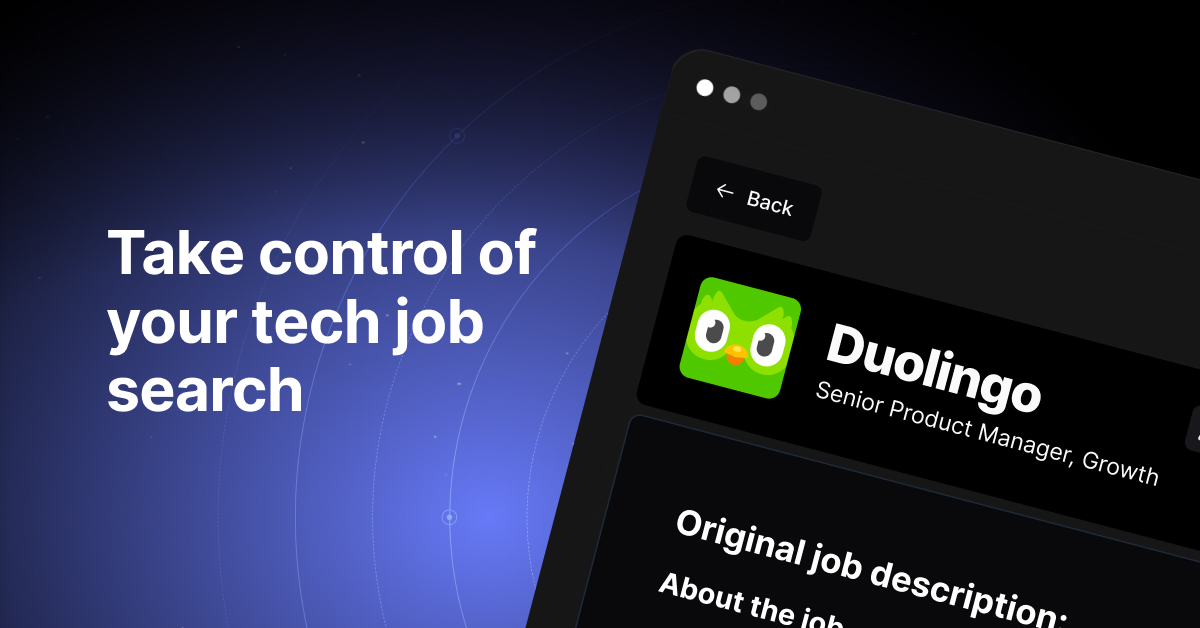