Combine several images horizontally with Python
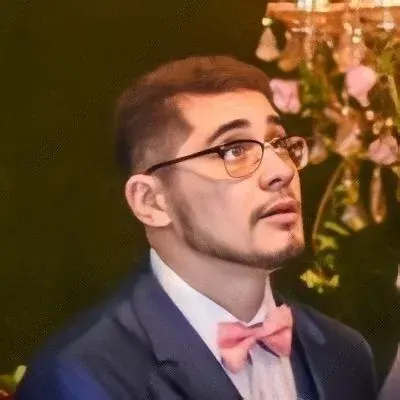
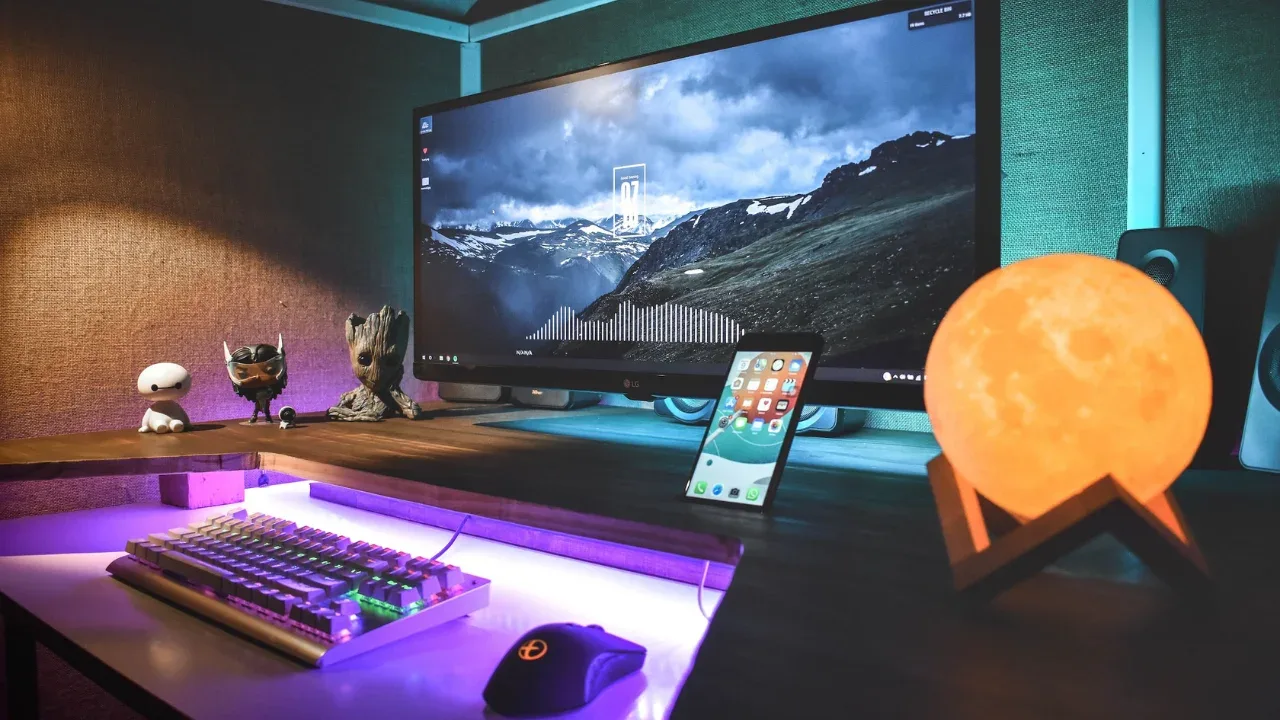
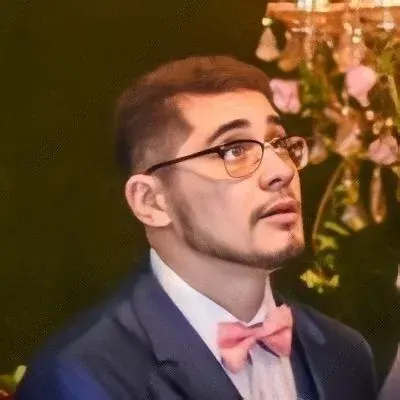
Combining Images Horizontally with Python 🖼️
Are you looking to horizontally combine multiple images in Python? Look no further! In this guide, we'll address the common issues surrounding this task and provide you with easy solutions to achieve the desired result. By the end of this post, you'll be able to concatenate as many images as you want, all while avoiding any extra partial images. Let's dive in! 🏊♂️
The Problem 🤔
The original poster has three images, each measuring 148 x 95 pixels. The goal is to horizontally join these images together. However, the initial attempt produced an output image that contained an unwanted extra partial image.
The Solution 💡
To achieve the desired outcome, we need to make a few adjustments to the provided code. Let's break it down step by step.
First, make sure to import the necessary modules:
import sys
from PIL import Image
Next, define the list of image filenames that you want to concatenate horizontally:
list_im = ['Test1.jpg','Test2.jpg','Test3.jpg']
To create an empty image that will serve as the canvas for concatenation, we need to specify the dimensions. In this case, we can utilize the dimensions of the individual images and calculate the total width:
total_width = sum([Image.open(filename).width for filename in list_im])
height = Image.open(list_im[0]).height
Now, we can create the blank canvas:
new_im = Image.new('RGB', (total_width, height))
Let's modify the loop to paste the images correctly, ensuring that there are no partial images:
current_width = 0
for filename in list_im:
im = Image.open(filename)
new_im.paste(im, (current_width, 0))
current_width += im.width
Finally, save the concatenated image with the desired filename:
new_im.save('concatenated_image.jpg')
With these changes in place, you should now be able to horizontally concatenate your images without any extra partial images!
Additional Tips and Tricks 🌟
If you want to make the code more flexible and avoid hard-coding the image dimensions, you can further enhance it. Here's how:
Define a variable for the desired height and width:
desired_height = 95
desired_width = 444
Modify the code block that calculates the total width:
total_width = len(list_im) * desired_width
Adjust the code for creating the blank canvas:
new_im = Image.new('RGB', (total_width, desired_height))
By making these changes, you can easily specify the desired dimensions in one line, offering more flexibility for future modifications. 📏
Share Your Concatenated Creations! 📤
Now that you have the power to horizontally combine multiple images with Python, why not give it a try? Feel free to experiment and share your fantastic creations! Whether it's creating a collage or merging screenshots, the possibilities are endless. 🌈
If you have any questions or other cool solutions to achieve the same result, please share them in the comments section below. Let's learn and grow together! 😄
Happy coding! 💻✨