Class inheritance in Python 3.7 dataclasses
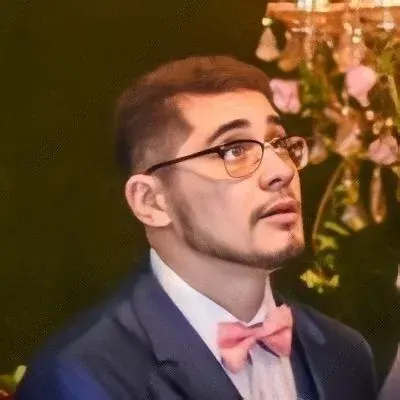
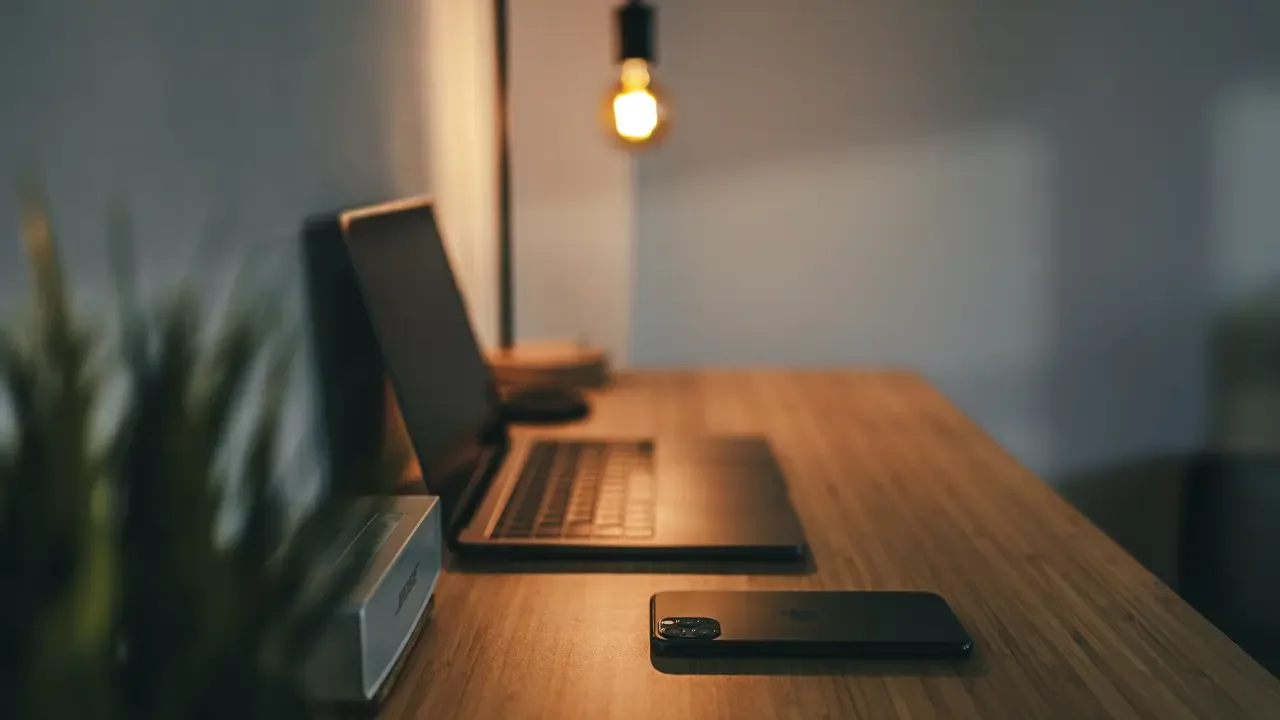
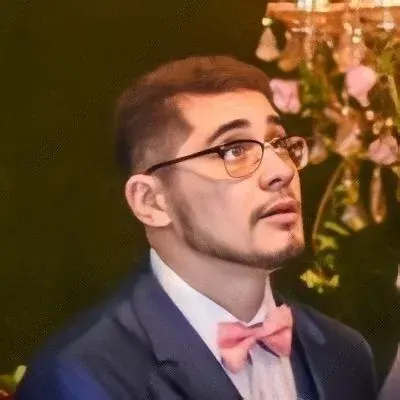
Class Inheritance in Python 3.7 Dataclasses: Handling Argument Order
If you're currently exploring the new dataclass constructions introduced in Python 3.7, you may come across situations where you need to handle class inheritance. This blog post will address a common issue related to argument order in class inheritance with dataclasses, provide an easy solution, and empower you to engage with the Python community.
The Problem: Argument Order and Type Error
Let's take a look at the provided code snippet:
from dataclasses import dataclass
@dataclass
class Parent:
name: str
age: int
ugly: bool = False
def print_name(self):
print(self.name)
def print_age(self):
print(self.age)
def print_id(self):
print(f"The Name is {self.name} and {self.name} is {self.age} years old")
@dataclass
class Child(Parent):
school: str
ugly: bool = True
jack = Parent('jack snr', 32, ugly=True)
jack_son = Child('jack jnr', 12, school='Harvard', ugly=True)
jack.print_id()
jack_son.print_id()
When running this code, you encounter a TypeError
with the following message:
TypeError: non-default argument 'school' follows default argument
The Solution: Reordering Arguments
The problem arises from the order of arguments in the child class, where the school
argument is placed after the ugly
argument, which has a default value. To fix this, we need to reorder the arguments.
from dataclasses import dataclass
@dataclass
class Parent:
name: str
age: int
ugly: bool = False
def print_name(self):
print(self.name)
def print_age(self):
print(self.age)
def print_id(self):
print(f"The Name is {self.name} and {self.name} is {self.age} years old")
@dataclass
class Child(Parent):
ugly: bool = True
school: str # Reordered argument
jack = Parent('jack snr', 32, ugly=True)
jack_son = Child('jack jnr', 12, ugly=True, school='Harvard') # Reordered argument
jack.print_id()
jack_son.print_id()
By placing the ugly
argument before the school
argument in the child class, we prevent the TypeError
from occurring.
Engage and Learn
Understanding class inheritance and argument order in dataclasses is crucial for writing clean and maintainable code. If you found this post helpful, let us know by leaving a comment or sharing it with fellow Python enthusiasts.
Have you encountered any other issues or challenges related to dataclasses in Python 3.7? Share your experiences and questions in the comments section below. Let's create a vibrant and supportive community that helps each other grow as Python developers!
Keep exploring, keep coding, and stay passionate about Python! 🚀