Check if string matches pattern
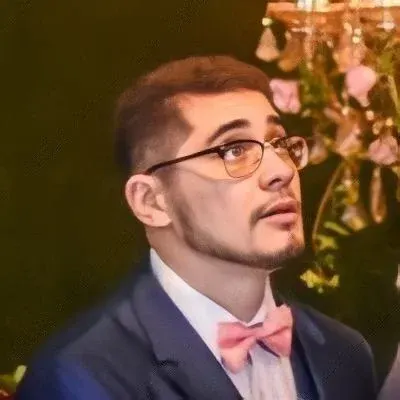
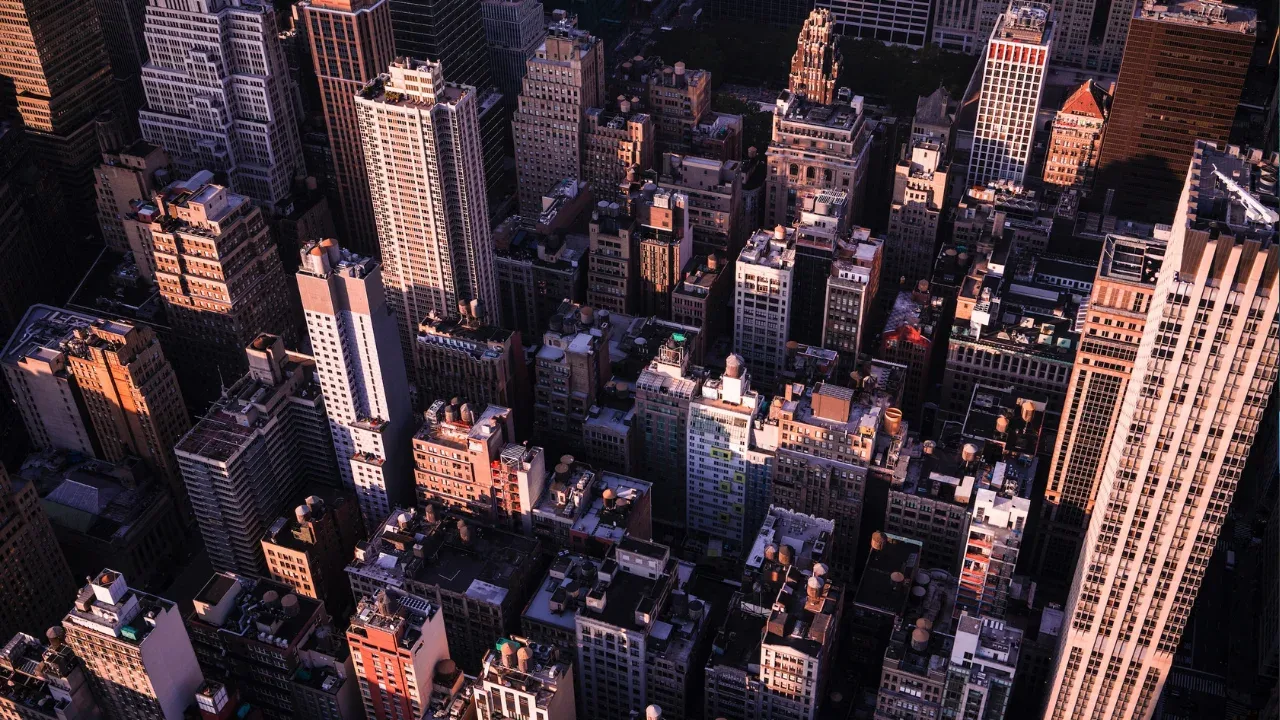
How to Check If a String Matches a Pattern
Are you struggling to determine if a string matches a specific pattern? π€ Don't worry - we've got you covered! In this blog post, we'll address the common issue of checking if a string matches a certain pattern and provide easy solutions to help you achieve your desired outcome. πͺ
The Problem: Matching a Specific Pattern
First, let's understand the problem at hand. You want to check if a string adheres to a specific pattern that consists of an uppercase letter, followed by one or more numbers, and then another uppercase letter, followed by more numbers, and so on. π
Examples of Matching and Non-Matching Strings
To make things clearer, let's take a look at some examples. The following strings would match the pattern:
A1B2
B10L1
C1N200J1
On the other hand, these strings wouldn't match the pattern:
a1B2
The first letter is lowercase, which doesn't match the required uppercase letter.
A10B
The second letter is lowercase, which doesn't match the required uppercase letter.
AB400
There are no numbers between the uppercase letters, which doesn't match the required pattern.
We hope these examples help you understand the problem and the expected outcomes better. Now, let's move on to the solutions! π
Solution: Regular Expressions to the Rescue!
Regular expressions are a powerful tool for pattern matching in strings. They allow you to define complex patterns and check if a string matches them. In our case, we can utilize a regular expression to validate whether a string matches the specified pattern.
Here's an example of a regular expression that matches our pattern:
^[A-Z][0-9]+[A-Z][0-9]+$
Let's break it down:
^
asserts the start of the string.[A-Z]
matches any uppercase letter.[0-9]+
matches one or more numbers.[A-Z]
matches another uppercase letter.[0-9]+
matches more numbers.$
asserts the end of the string.
This regular expression ensures that the entire string consists of alternating uppercase letters and numbers. Nice, isn't it? π
Applying the Solution
Now that we have our regular expression, let's apply it in your preferred programming language. Here's an example in JavaScript:
const pattern = /^[A-Z][0-9]+[A-Z][0-9]+$/;
function isValidString(input) {
return pattern.test(input);
}
// Usage:
console.log(isValidString("A1B2")); // true
console.log(isValidString("a1B2")); // false
console.log(isValidString("AB400")); // false
In this example, we define a function isValidString
that takes an input string and uses the test
method of the regular expression pattern to check for a match. The function returns true
if the string matches the pattern and false
otherwise.
Feel free to adapt this code to your preferred programming language or framework. The core concept remains the same - utilize a regular expression to check if the string matches the desired pattern.
You're Ready to Go!
Congratulations! You now know how to check if a string matches a specific pattern using regular expressions. π
Next time you encounter a similar problem, don't fret. Just apply the knowledge you've gained from this blog post and find the perfect pattern match with ease. Remember, regular expressions are your trusty sidekick in the world of string matching. π¦ΈββοΈ
If you have any questions or want to share your own experiences with pattern matching, feel free to leave a comment below. We'd love to hear from you! Let's keep the conversation going. π¬β¨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
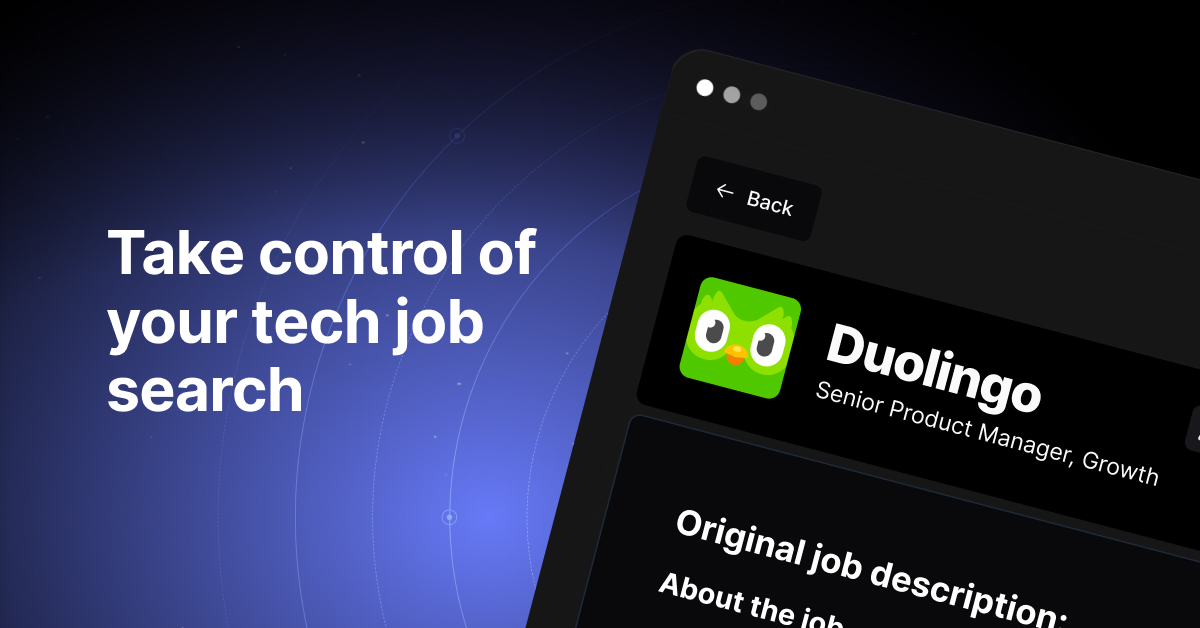