Check if a given key already exists in a dictionary
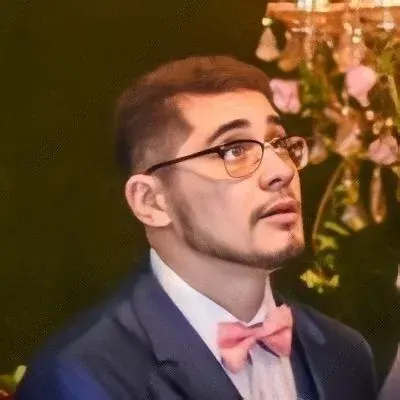
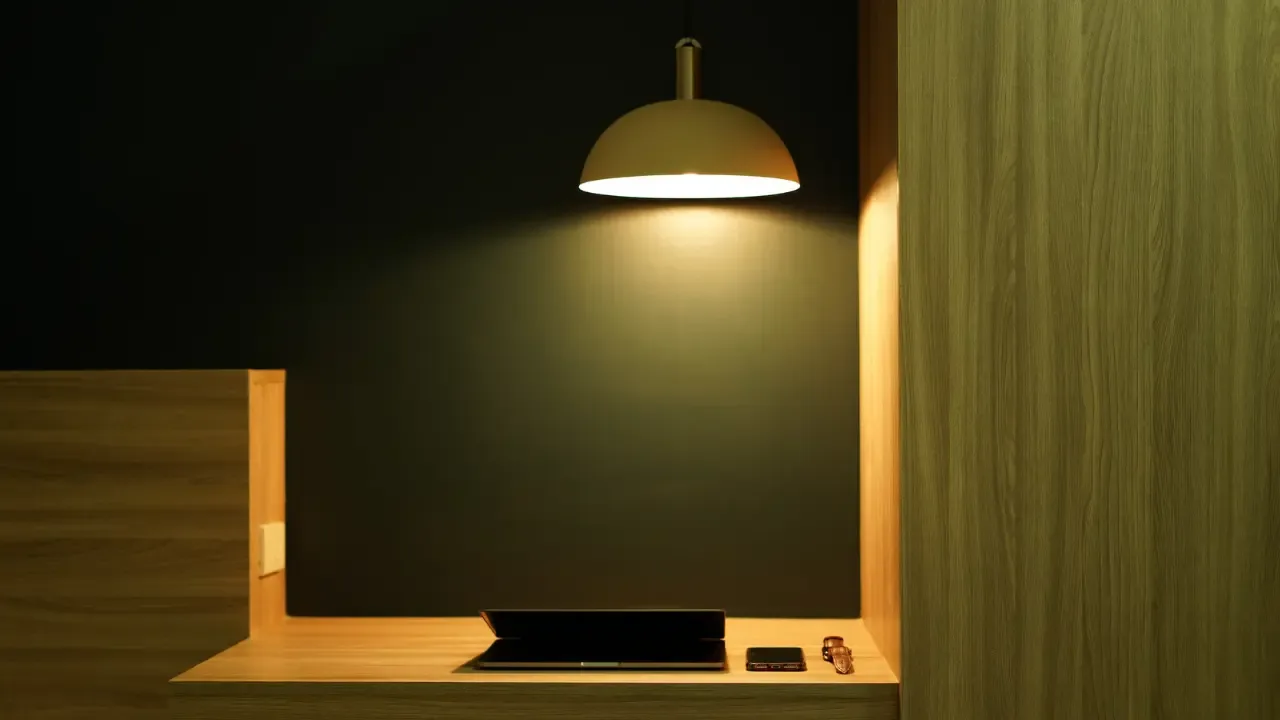
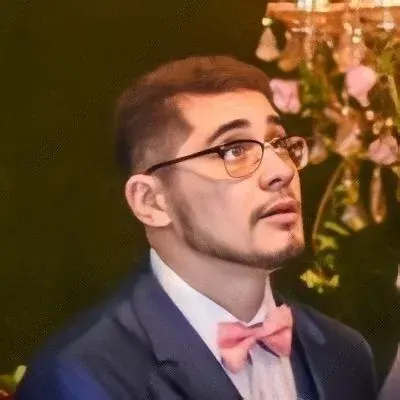
🗝️ How to Check if a Key Exists in a Dictionary?
Are you tired of feeling unsure if a key exists in a dictionary? Well, worry no more! 🙌 In this blog post, we'll address this common issue and provide you with easy solutions to nail the task. 💪
🤔 The Problem:
Let's start with the context. You have a dictionary, and you want to test if a certain key exists before updating its value. You already tried the following code:
if 'key1' in dict.keys():
print("blah")
else:
print("boo")
But you feel like there must be a better way to accomplish this task. And you're right! 🎉
🚀 The Solution:
Python provides a built-in function called dict.get()
that you can use to directly test if a key exists in a dictionary. Here's the improved code:
if dict.get('key1') is not None:
print("blah")
else:
print("boo")
By using dict.get('key1')
, you can check if the value associated with 'key1'
is None
. If it is, the key doesn't exist in the dictionary. If it's not None
, then congrats, you found the key! 🎉
✨ A Simpler Approach:
But wait, there's an even simpler way to achieve the same result! You can directly use the in
operator on the dictionary itself. Check out this elegant code snippet:
if 'key1' in my_dictionary:
print("blah")
else:
print("boo")
By using the in
operator, you can directly check if 'key1'
exists within the dictionary my_dictionary
. No need for any additional functions or complexities. It's straightforward and efficient! 😎
👉 Your Turn:
Now that you have learned these cool techniques to check for the existence of a key in a dictionary, it's time to put your knowledge into action! Take your code to the next level and try it out. Experiment with different dictionaries and see how it plays out. 🔍
And don't forget to share your experiences in the comments below. Did you find this post helpful? Did you encounter any challenges? We'd love to hear from you! 📝
So go ahead, write some code, and let us know how you're doing. Let's dive into the world of Python dictionaries together! 🐍💼
Keep coding, keep exploring! 🚀✨