check if a file is open in Python
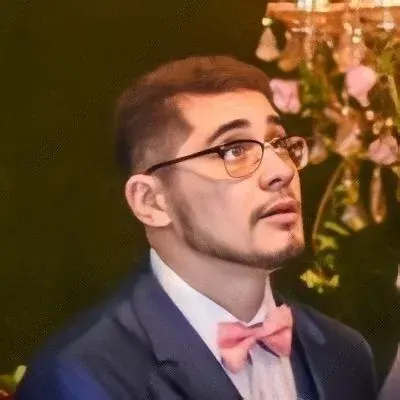
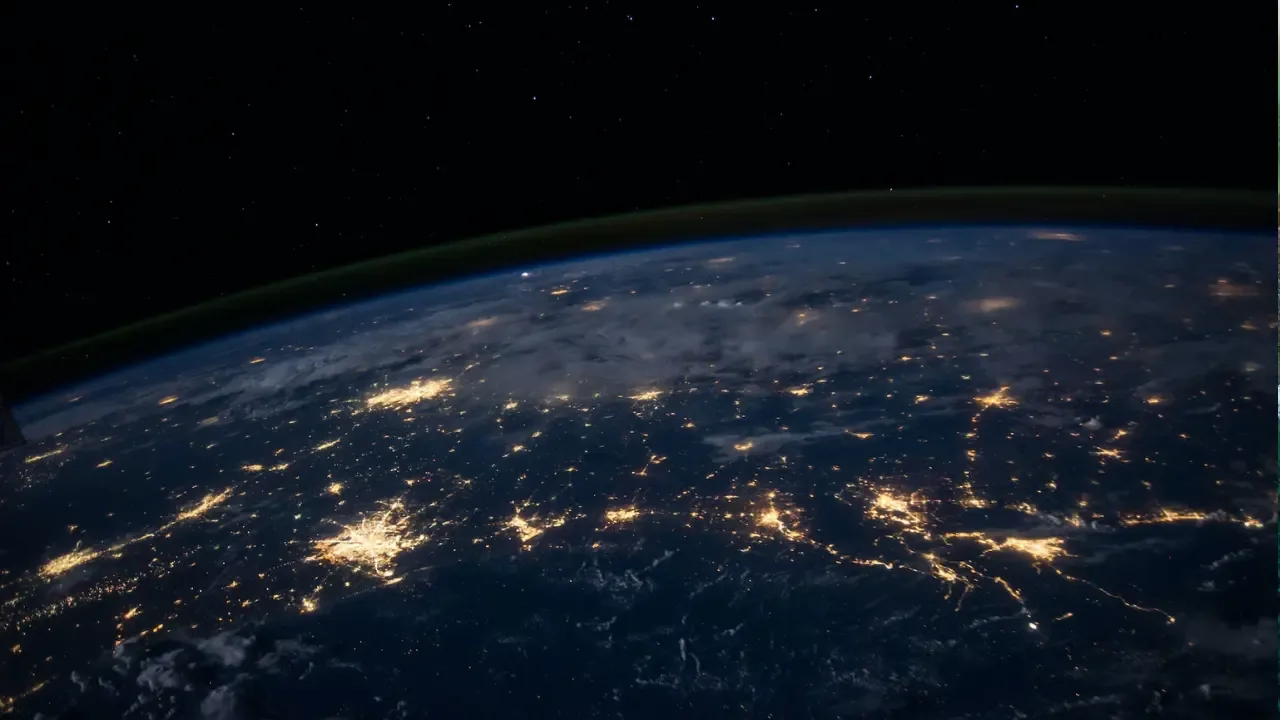
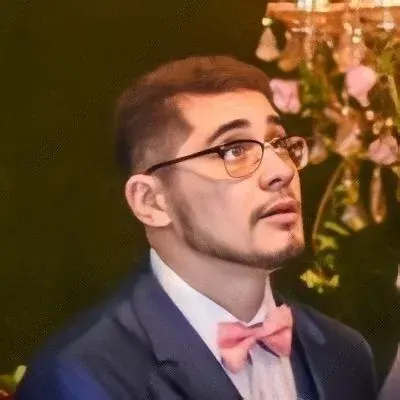
Title: "Is the File Open? The Pythonic Way to Check and Avoid Writing Disasters"
Introduction: Hey Techies! 👋 Are you tired of writing scripts that cause unexpected errors because a file is left open? 📂 Don't worry, we've got your back! In this blog post, we'll discuss the common issue of checking if a file is open in Python 🐍 and provide you with easy solutions to handle this problem like a pro! Let's dive in and never experience writing disasters again! 😎
Understanding the Problem:
So, you're building an app, and you write data to an Excel file. But here's the catch: sometimes users forget to close the file before any further writing! 😱 This can lead to corrupted data and errors in your application. To prevent such catastrophes, you need a way to check if the file is open.
The Pythonic Solution:
To check if a file is open, we can use the psutil
library in Python, which provides cross-platform support for retrieving information about running processes. Here are the steps to follow:
Step 1: Install psutil
library:
pip install psutil
Step 2: Import the necessary modules:
import psutil
import os
Step 3: Write a function to check if the file is open:
def is_file_open(file_path):
for proc in psutil.process_iter():
try:
files = proc.open_files()
for file in files:
if file_path == file.path:
return True
except (psutil.NoSuchProcess, psutil.AccessDenied, psutil.ZombieProcess):
pass
return False
Step 4: Call the function and handle the response:
file_path = "path/to/your/file.xlsx"
if is_file_open(file_path):
# File is open! Take necessary actions.
print("⚠️ Warning: The file is already open! Please close it before continuing.")
else:
# File is not open. Carry on.
print("✅ File is not open. Safe to proceed writing.")
Additional Tips:
It's always a good practice to display a warning message or inform the user before performing any critical operations on an open file.
Make sure to handle potential exceptions that may occur while using
psutil
, such aspsutil.NoSuchProcess
,psutil.AccessDenied
, orpsutil.ZombieProcess
.Remember to replace
"path/to/your/file.xlsx"
with the actual path to your file.
Conclusion:
By implementing this Python code snippet and checking if a file is open before writing, you can avoid those nasty writing disasters and keep your app running smoothly. 🌟 Say goodbye to errors caused by open files and say hello to hassle-free coding! 💪
Feel free to try out this solution and let us know in the comments how it worked for you. 👇 Have any additional tips or tricks to share? We'd love to hear about them too! Let's keep the conversation going! 💬
Now, go forth and write confidently! 🚀