Change column type in pandas
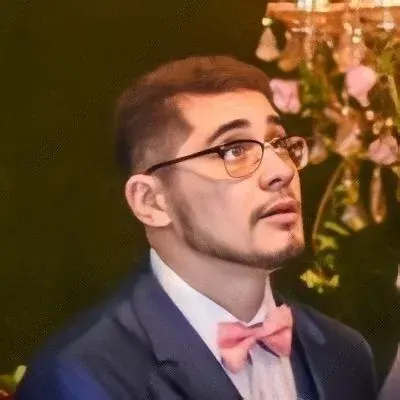
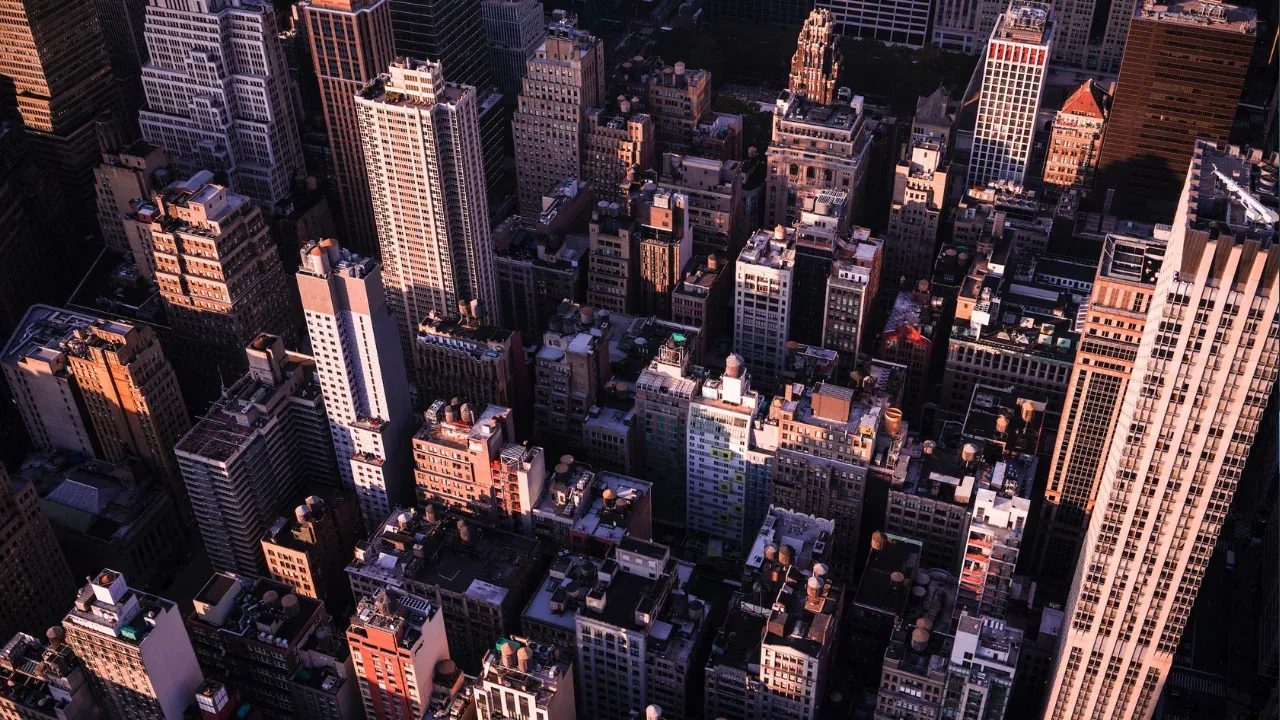
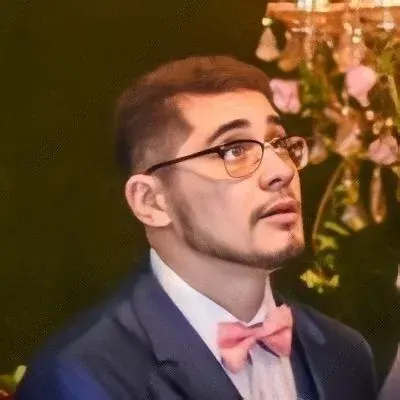
💻 Blog Post: How to Change Column Types in Pandas
If you've ever found yourself wanting to convert column types in Pandas, you're not alone. It's a common problem that can cause headaches if not approached correctly. 🤯
In this blog post, we'll explore a specific problem and provide easy solutions to convert column types in Pandas. Whether you're a beginner or an experienced Pandas user, we've got you covered! 😎
The Problem: Converting Columns to Specific Types
Let's start by discussing the specific problem at hand. Suppose you have created a DataFrame from a list of lists:
table = [
['a', '1.2', '4.2' ],
['b', '70', '0.03'],
['x', '5', '0' ],
]
df = pd.DataFrame(table)
Here's the question: How do you convert columns 2 and 3 into floats? 🤔
Solution 1: Specifying Types During DataFrame Creation
One approach is to specify the types while converting the list to DataFrame. Pandas provides the astype
function, which allows you to define the data type of each column.
df = pd.DataFrame(table, columns=['col1', 'col2', 'col3']).astype({'col2': float, 'col3': float})
By explicitly defining the column names and their corresponding types, you can create the DataFrame with the desired column types right away. 🚀
Solution 2: Change Column Types After DataFrame Creation
If it's not feasible to specify column types during DataFrame creation, don't worry! Pandas offers a flexible way to change column types after the DataFrame has been created.
Here's a handy method: astype
.
df['col2'] = df['col2'].astype(float)
df['col3'] = df['col3'].astype(float)
By applying the astype
method to the specific columns you want to modify, you can change their types to floats. It's as simple as that! 🔢
Solution 3: Dynamically Change Column Types
In some scenarios, you may have hundreds of columns and don't want to specify the type for each one individually. Don't worry, we've got you covered! 😄
Here's a dynamic approach to changing column types that can save you time and effort:
for column in df.columns:
df[column] = df[column].astype(float)
By looping through each column in the DataFrame, you can apply the astype
method to each column and convert them all to floats. 🔄
Conclusion
Congratulations! You now have multiple ways to change column types in Pandas. Whether you choose to specify the types during DataFrame creation, change the types after creation, or dynamically convert all columns, it's up to you!
Next time you encounter a similar problem, remember these solutions and save yourself from unnecessary headaches! 😌
So go ahead, give it a try, and let us know how it goes! Do you have any other Pandas questions or challenges? Share them in the comments below. We're here to help! 👇
Happy coding! 💪
DISCLAIMER: This blog post assumes you have a basic understanding of Pandas and Python. If you're new to Pandas, we recommend checking out some beginner-friendly resources before diving into this topic.
<!-- Call-to-Action -->
If you found this blog post helpful, please consider sharing it with your friends and colleagues. Let's spread the knowledge and make data manipulation in Pandas easier for everyone! 🙌
Tags: #Pandas #Python #DataFrames #DataManipulation