Catch multiple exceptions in one line (except block)
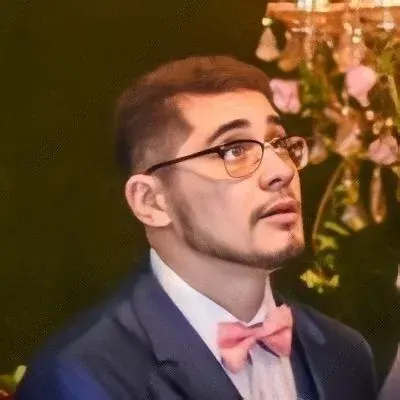
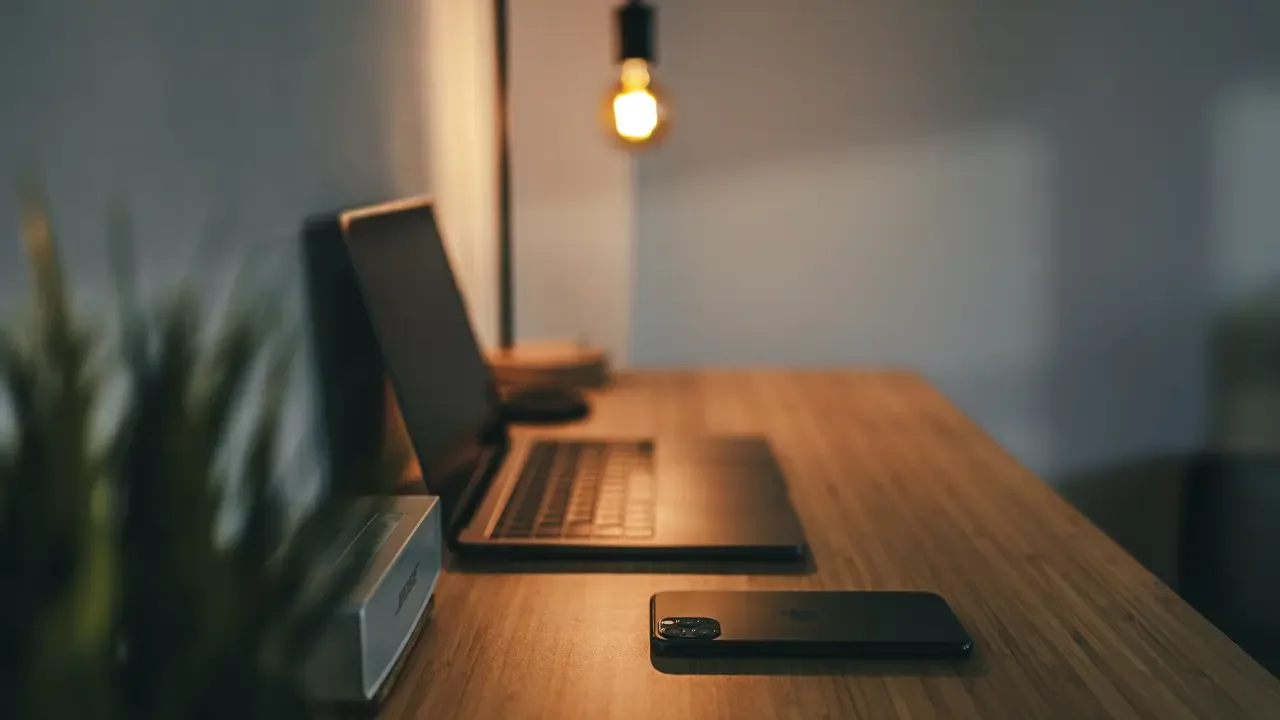
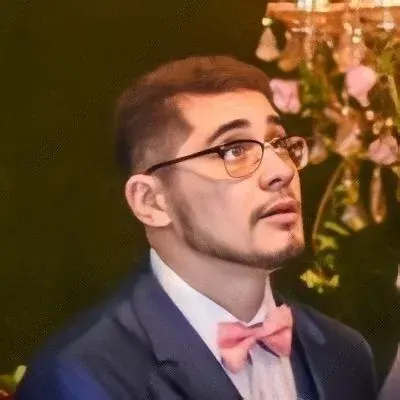
Catching Multiple Exceptions in One Line (Except Block) 😮💥
Have you ever encountered a situation where you need to handle different exceptions in the same way? This can often lead to repetitive code and make your code less maintainable. But fear not! In this guide, we'll explore how to catch multiple exceptions in one line using the except
block. 🙌
The Basics 😎
Let's start with the basics. You might already know that you can use a generic except
block to catch any exception that occurs in your code. For example:
try:
# do something that may fail
except:
# do this if ANYTHING goes wrong
This can be handy when you just want to handle any exception in the same way.
Catching Specific Exceptions 👀
But what if you want to handle different exceptions differently? Here's where things get interesting. You can use multiple except
blocks to catch specific exceptions and handle them individually. Check out this example:
try:
# do something that may fail
except IDontLikeYouException:
# say please
except YouAreTooShortException:
# stand on a ladder
In this case, if an IDontLikeYouException
is raised, you can handle it differently than a YouAreTooShortException
. This allows for more flexibility in your exception handling.
The Challenge 😫
Now, let's address the specific problem the asker posed. They want to perform the same action for two different exceptions. In the given example, they want to say please for both IDontLikeYouException
and YouAreBeingMeanException
.
The asker tried to do this but ended up with something like this:
try:
# do something that may fail
except IDontLikeYouException:
# say please
except YouAreBeingMeanException:
# say please
Going Beyond the Obvious 💡
The asker then wonders if there's a way to combine the two exceptions into a single except
block, similar to this:
try:
# do something that may fail
except IDontLikeYouException, YouAreBeingMeanException:
# say please
However, this syntax doesn't work as intended. It actually matches the syntax for catching any exception, such as:
try:
# do something that may fail
except Exception as e:
# say please
So, how can we achieve the desired result?
The Solution 🚀
To catch multiple exceptions in a single line, we can use parentheses to enclose the exceptions. Here's how it looks:
try:
# do something that may fail
except (IDontLikeYouException, YouAreBeingMeanException):
# say please
By grouping the exceptions within parentheses, we can catch both IDontLikeYouException
and YouAreBeingMeanException
and perform the desired action in a concise way.
Your Turn to Try 🤩
Now that you know the secret to catching multiple exceptions in one line, try implementing it in your own code. It will help you avoid duplicating code and make your exception handling more elegant.
Share your experience in the comments below! Have you encountered any other tricky exception handling scenarios? Let's discuss and find solutions together!
Happy coding! 👩💻👨💻