Catch and print full Python exception traceback without halting/exiting the program
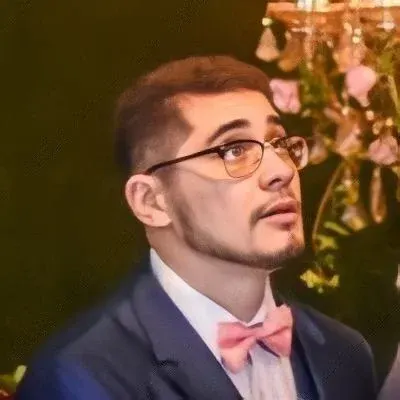
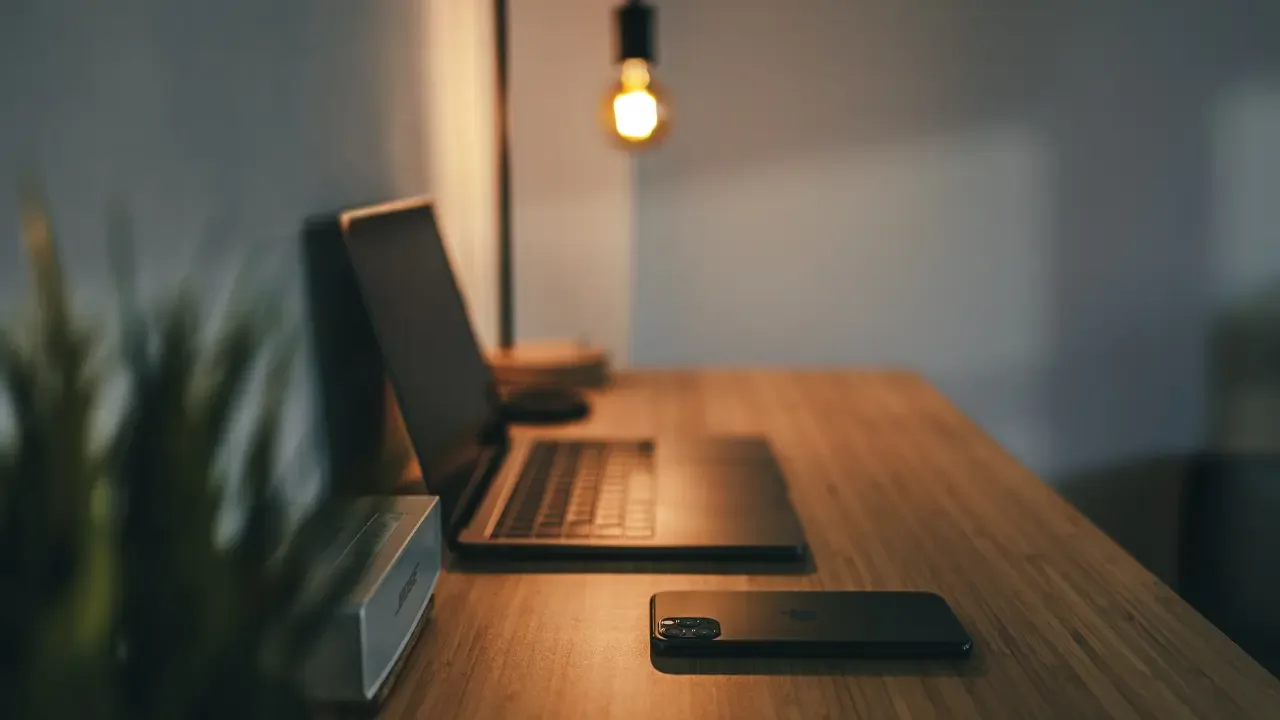
📝🔥🔍 How to Print Full Python Exception Traceback Without Halting/Exiting the Program
Hey there fellow Pythonista! 😄 Are you looking for a way to catch and log exceptions without exiting your program? Well, you've come to the right place! In this blog post, we'll dive into one common issue that many Python developers face and provide easy solutions to help you print the full traceback without interrupting your program. Let's get started! 🚀
The Problem: Catching Exceptions and Printing Full Tracebacks
Imagine this scenario: you have a block of code wrapped in a try-except
statement to handle any potential exceptions that may occur. Inside the except
block, you want to print not only the exception name and details but also the complete traceback that you would see if the exception was raised without interception. How can you achieve this?
Here's an example of code that reflects this problem:
try:
do_stuff()
except Exception as err:
print(Exception, err)
# I want to print the entire traceback here,
# not just the exception name and details
Your goal is to modify this code snippet to print the exact same output that you would see if the exception was raised without the try-except
block, without halting or exiting your program. Let's explore some solutions together! 💡
Solution 1: Using the traceback
Module
Python provides the traceback
module, which allows you to extract and format the full traceback of an exception. You can achieve this by importing the traceback
module and using the print_exc()
or format_exc()
methods.
Here's an updated version of our code using traceback
:
import traceback
try:
do_stuff()
except Exception as err:
print(Exception, err)
traceback.print_exc() # or use traceback.format_exc() to capture the traceback as a string
By adding the traceback.print_exc()
method call, you can now print the full traceback in addition to the exception name and details. Remember, don't worry about the traceback
module printing the traceback twice. It's just displaying the traceback twice to ensure it is shown on the console.
Solution 2: Using the sys
Module
Another alternative is to use the sys
module, which provides access to some variables used or maintained by the interpreter, including the last exception raised. You can access the last exception using sys.exc_info()
, which returns a tuple containing the exception type, the exception instance, and the traceback object.
Let's incorporate this solution into our code:
import sys
try:
do_stuff()
except Exception as err:
print(Exception, err)
exc_type, exc_obj, exc_tb = sys.exc_info()
print(exc_type, exc_obj)
traceback.print_tb(exc_tb)
By using sys.exc_info()
and accessing the traceback object in the returned tuple, you can print the full traceback. Using this method, you have more flexibility to further process and handle the exception and traceback as needed.
Stay Curious and Keep Exploring!
Now that you know how to catch and print full Python exception tracebacks without halting or exiting your program, you can enhance your debugging and error-handling capabilities. Remember, exceptions are your allies in finding and fixing bugs! 🐜🔨
Feel free to experiment with these solutions and adapt them to your specific use cases. If you found this post helpful, don't forget to share it with your fellow Python enthusiasts! 👍
Do you have any other Python questions or topics you'd like us to cover? We love hearing from our readers! Leave a comment below and let's keep the conversation going. Happy coding! 😊💻
[Call-to-action] Don't forget to subscribe to our newsletter for more helpful Python tips, tutorials, and tricks. Join our Python community today! 💌🐍
Now go forth and print those tracebacks! 🔍🐞
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
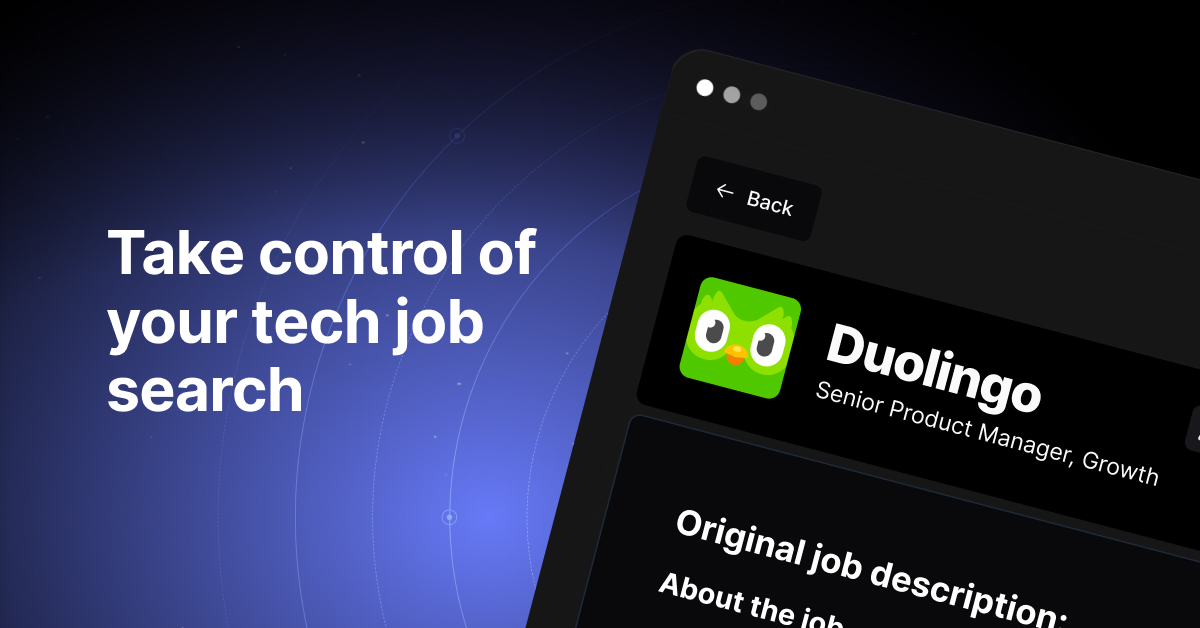