Case insensitive regular expression without re.compile?
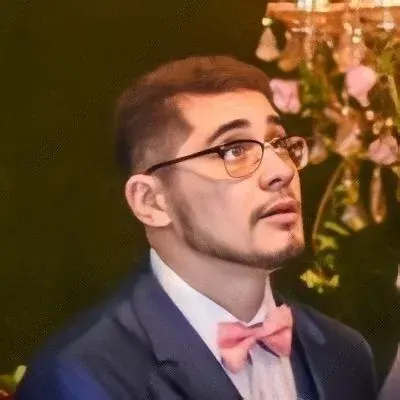
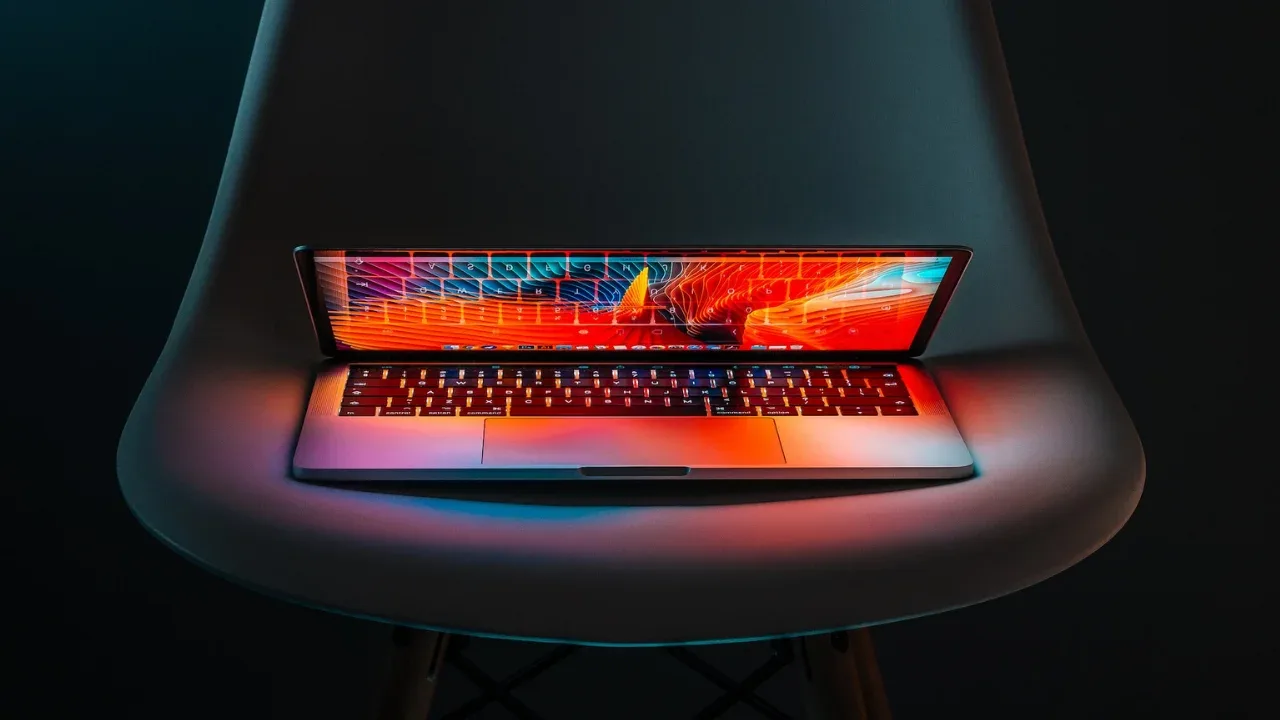
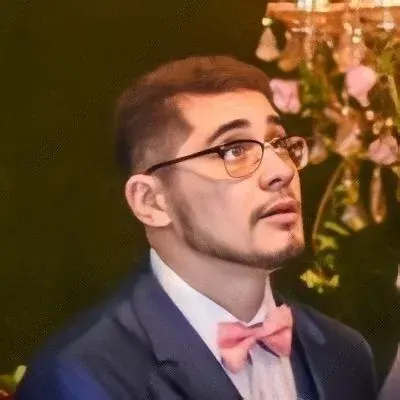
📝👉🔎 Case Insensitive Regular Expression in Python: No re.compile
?
Are you struggling to find an easy way to write a case-insensitive regular expression in Python without using re.compile
? 😕 Look no further, my friend! In this blog post, I'll show you a simple and hassle-free solution to your problem. 🎉
Let's start by understanding the problem. In Python, you can use re.compile
to create regular expression objects with various options. One of those options is re.IGNORECASE
, which makes the regular expression case-insensitive. However, what if you can't or don't want to use re.compile
for some reason? 🤔 That's where things get interesting.
Many developers wonder if Python has a built-in syntax or flag, similar to Perl's i
suffix (e.g., m/test/i
), to achieve case insensitivity without using re.compile
. Unfortunately, the documentation doesn't explicitly mention such a feature. But fear not, because I've got a nifty workaround for you! 😎
Here's how you can write a case-insensitive regular expression in Python without using re.compile
:
import re
s = 'TeSt'
pattern = '(?i)test'
if re.match(pattern, s):
print("Match found!")
else:
print("No match.")
In this code snippet, we utilize a technique called "inline flags." 👌 By adding the (?i)
flag at the beginning of the regular expression pattern, we indicate that the matching process should be case-insensitive.
When you run this code, you'll see the output:
Match found!
Voilà! 🎩✨ We achieved the desired case insensitivity without explicitly using re.compile
. The (?i)
flag does the trick for us.
You might be wondering, "How does this work?" Well, the (?i)
flag is a way to set the case-insensitive option for the specific regular expression pattern it precedes. It acts similarly to the re.IGNORECASE
flag when used with re.compile
. Pretty neat, isn't it? 😄
Now that we've cracked the case of case insensitivity, you can save yourself some lines of code by using this inline flag technique. Plus, it's a cool trick to impress your fellow developers at the next coding meetup! 🤓
Remember, when using inline flags, make sure you only apply them to the specific regular expression pattern where you need case insensitivity. Otherwise, it might produce unintended results.
So, the next time you encounter a situation where using re.compile
is not feasible or desired, remember this handy inline flag technique to create case-insensitive regular expressions in Python. 💪
Have any other cool Python tricks up your sleeve? Share them with us in the comments below and let's geek out together! 🚀💬
📢 Call-to-Action: Join the Conversation!
Found this blog post helpful? 😉 Engage with us and share your thoughts in the comments section. Let us know if you have any questions, additional tips, or even funny anecdotes related to case-insensitive regular expressions in Python.
Connect with our vibrant community of Python enthusiasts and stay up to date with the latest tech trends by subscribing to our newsletter. 💌👩💻
Happy coding! 🎉🐍