Can"t subtract offset-naive and offset-aware datetimes
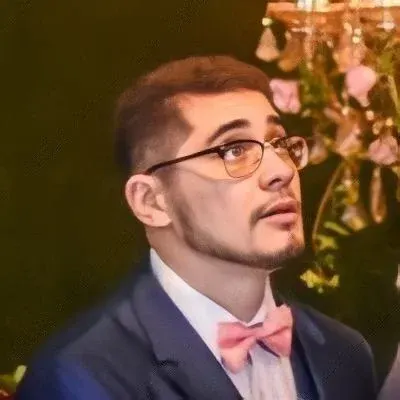
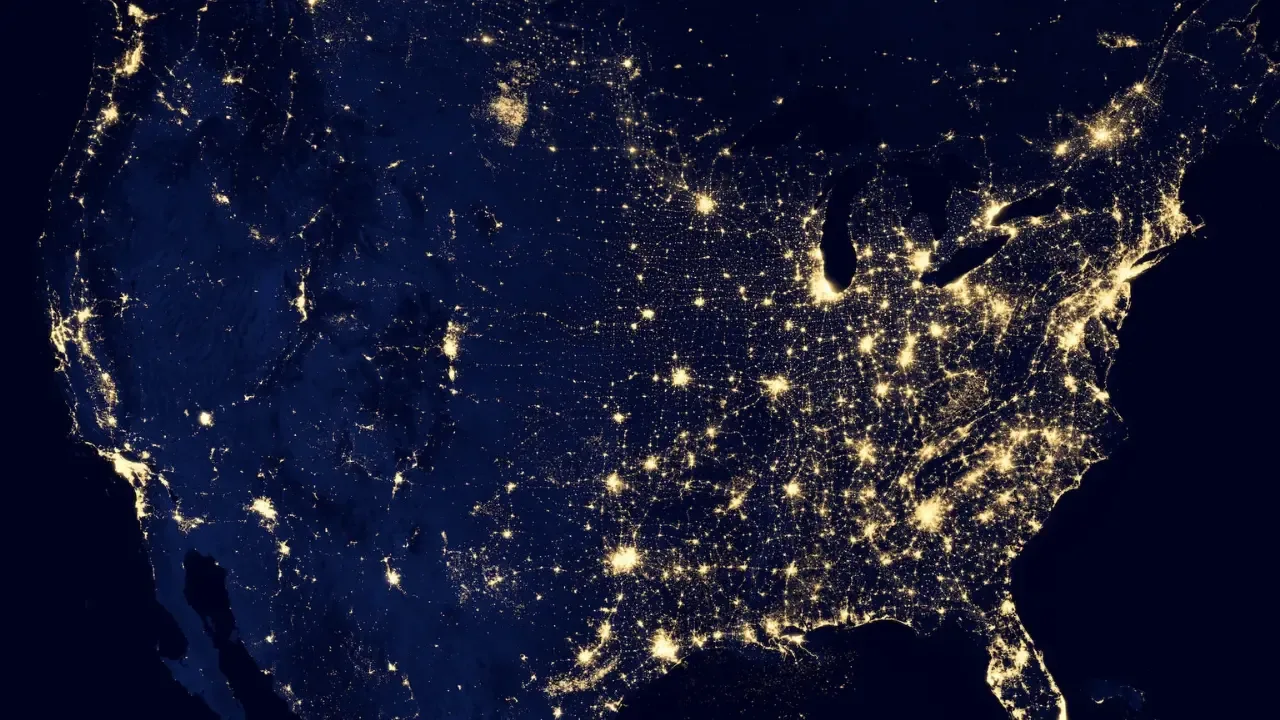
🕒 Can't subtract offset-naive and offset-aware datetimes
So you're trying to calculate the age of a timestamp in PostgreSQL, but running into an error: TypeError: can't subtract offset-naive and offset-aware datetimes
. Sounds frustrating, right? But don't worry, I'm here to help you tackle this issue head-on!
⚠️ The Problem
The root of the problem lies in the mismatch between the timezone awareness of your datetime objects. You have a timezone-aware timestamptz
field in PostgreSQL, but when you retrieve the data and try to subtract the current time using datetime.datetime.now()
or datetime.datetime.utcnow()
, you're left with a timezone-unaware datetime object.
⛑️ The Solution
Luckily, there are a couple of easy solutions to get past this roadblock. Let's explore them:
🌍 Solution 1: Adjusting the Timezone
One way to handle this is by adjusting the timezone of the current time to match the timezone of your timestamptz
field. Here's how you can do it:
import pytz
import datetime
current_time = datetime.datetime.now(pytz.timezone('Your_Timezone'))
Make sure to replace 'Your_Timezone'
with the specific timezone you're working with.
🔄 Solution 2: Converting to UTC
Another approach is to convert your timestamptz
field to UTC before performing the subtraction. This ensures both timestamps are in the same timezone. Here's an example:
import datetime
# datetime object of the current time in UTC
current_time = datetime.datetime.utcnow()
# datetime object retrieved from the PostgreSQL query
db_time = get_db_time()
age = current_time - db_time
By using datetime.datetime.utcnow()
, you obtain a timezone-aware datetime object in UTC. Just replace get_db_time()
with the appropriate code to retrieve the database timestamp.
⭐ Solution 3: Always Insert using UTC
If none of the above options suit you, an alternative would be to consistently insert timestamps into the PostgreSQL table as UTC. This way, you can avoid the hassle of dealing with conversions and timezones during retrieval. You can achieve this by using the AT TIME ZONE 'UTC'
clause in your queries like this:
INSERT INTO your_table (timestamp_column)
VALUES (NOW() AT TIME ZONE 'UTC');
This ensures all the timestamps stored in your table are in UTC by default.
🙌 Conclusion
And there you have it! Now you know how to tackle the "can't subtract offset-naive and offset-aware datetimes" error when working with timezone-aware and timezone-unaware datetimes in PostgreSQL. Remember, you have multiple solutions to choose from, depending on your specific requirements.
If you found this guide helpful, feel free to share it with your fellow developers who might be facing a similar issue. And don't hesitate to leave your thoughts or questions in the comments section below. Happy coding!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
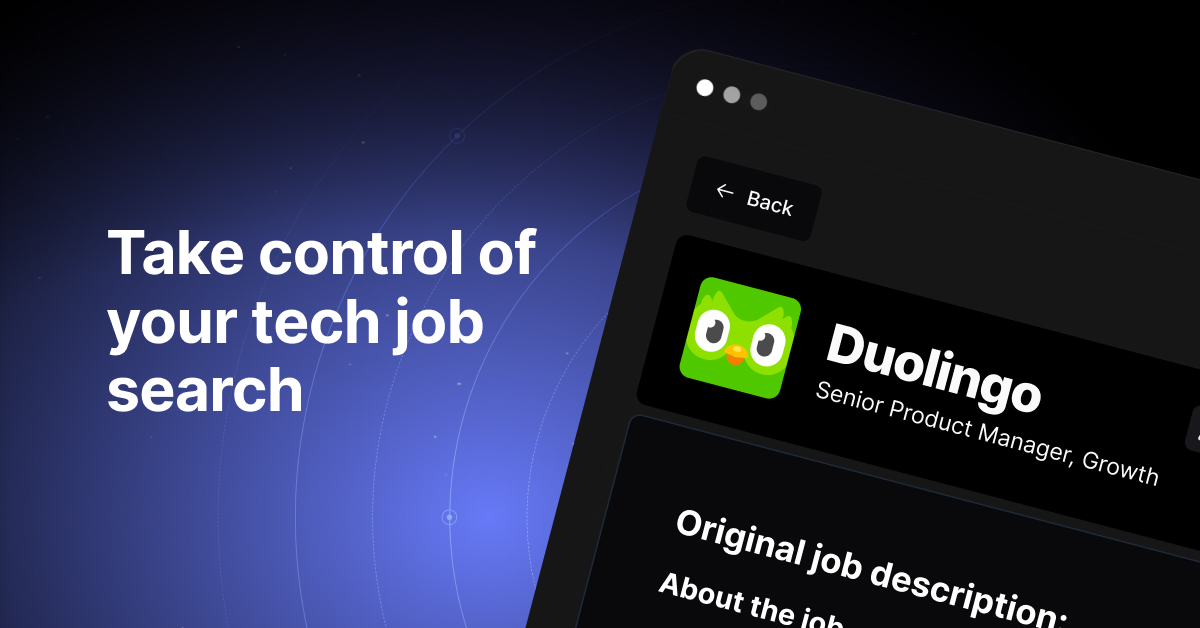