Can "list_display" in a Django ModelAdmin display attributes of ForeignKey fields?
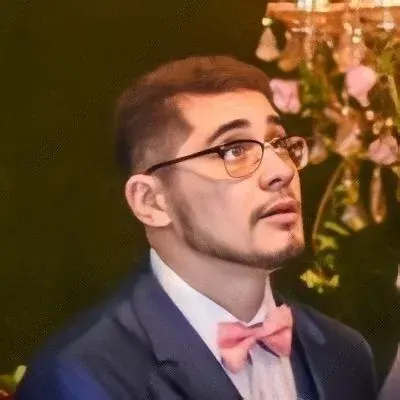
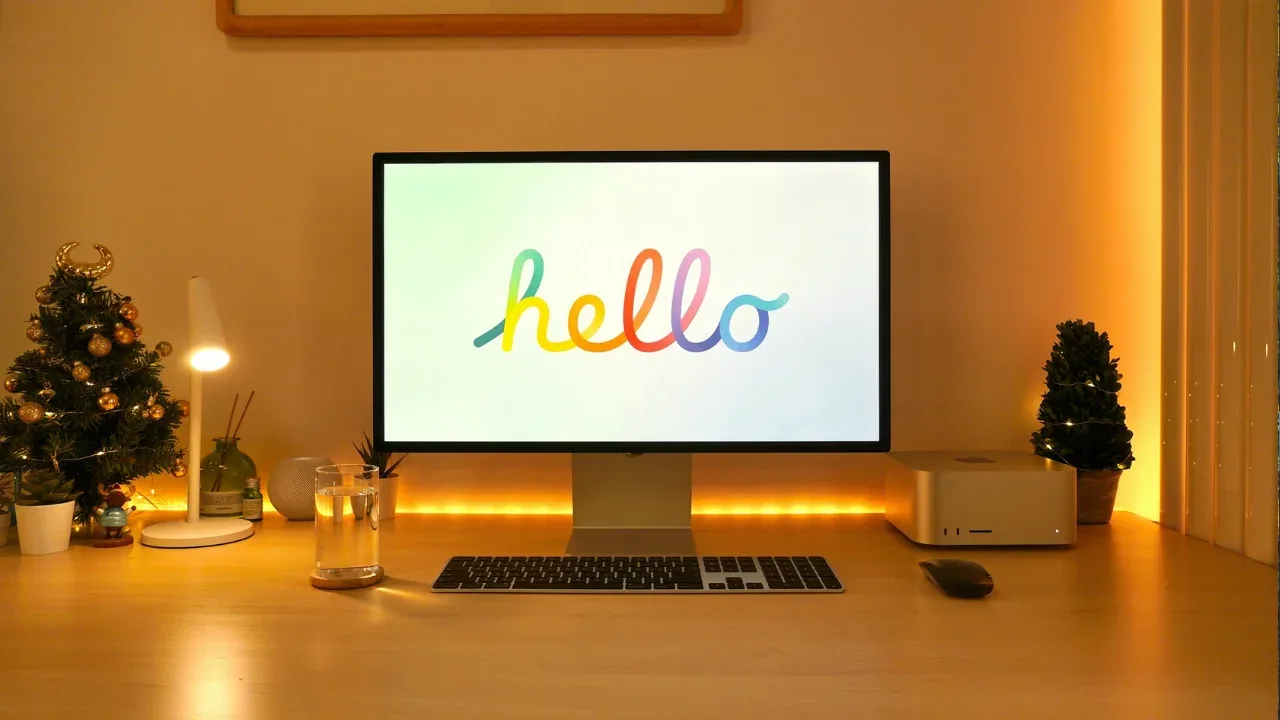
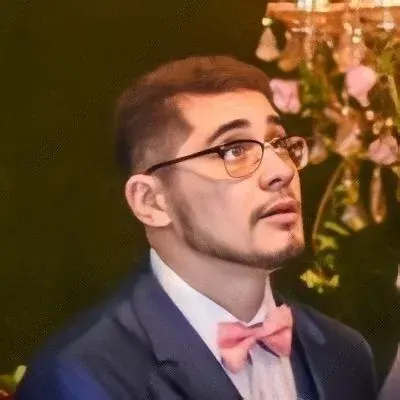
📝💡🤔 How to Display Attributes of ForeignKey Fields in Django ModelAdmin's list_display?
If you're facing the same question, no worries! Many Django developers have wondered whether the list_display
attribute in ModelAdmin
can display attributes of a ForeignKey
field. In this blog post, we'll explore this common issue, provide easy solutions, and end with a compelling call-to-action to encourage reader engagement.
Let's dive in!
🔍 Understanding the Problem
In the given context, there's a Person
model with a ForeignKey
relationship to Book
. You want to display the author
attribute from the Book
model using list_display
in PersonAdmin
. However, the traditional methods you tried didn't yield the desired results.
🛠️ Easy Solution
To display attributes of ForeignKey
fields in list_display
, you can define a custom method in the PersonAdmin
class:
class PersonAdmin(admin.ModelAdmin):
list_display = ('display_author',)
def display_author(self, obj):
return obj.book.author
display_author.short_description = 'Author'
In this example, we created a new method called display_author
that accepts obj
as a parameter. Within this method, we access the author
attribute using obj.book.author
and return its value. By adding display_author
to list_display
, we can now see the author
attribute in the admin list view.
🌟 Optimizing the Solution
If you have multiple attributes from ForeignKey
fields that you want to display, you can create additional custom methods in PersonAdmin
and add them to list_display
. For example, if you also wanted to display the title
attribute from Book
, you could modify PersonAdmin
as follows:
class PersonAdmin(admin.ModelAdmin):
list_display = ('display_author', 'display_title',)
def display_author(self, obj):
return obj.book.author
display_author.short_description = 'Author'
def display_title(self, obj):
return obj.book.title
display_title.short_description = 'Title'
By expanding the number of custom methods and updating list_display
accordingly, you can effectively display attributes of ForeignKey
fields in Django's admin interface.
📣 Call-to-Action
Now that you have a clear understanding of how to display attributes of ForeignKey
fields in list_display
, why not give it a try in your Django project? Share your experience in the comments below and let us know if you encountered any challenges or found an even better solution. Happy Django coding! 😄💻
Share this blog post with fellow Django developers who might find it helpful!