Can I get JSON to load into an OrderedDict?
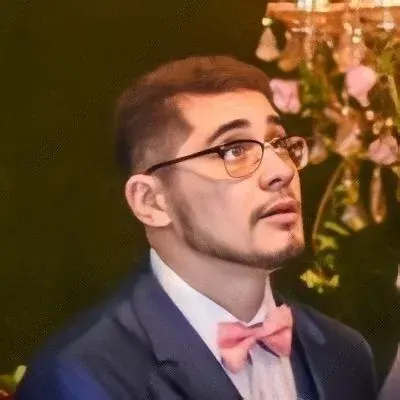
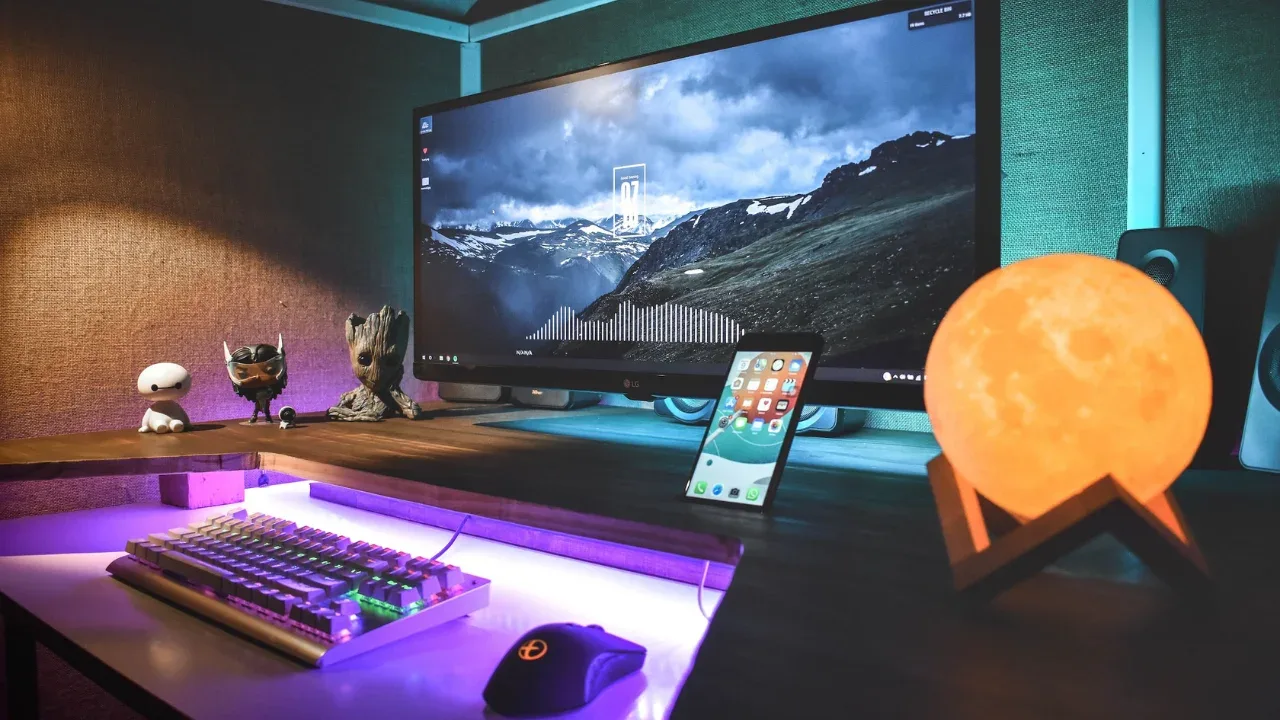
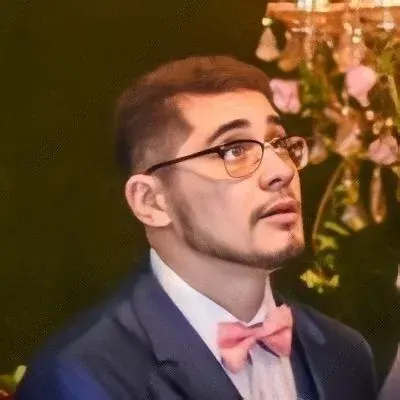
Title: JSON and OrderedDict: Maintaining Key Order Made Easy!
Introduction š Welcome to our tech blog, where we simplify complex problems and provide easy-to-follow solutions! Today, we'll tackle the question many developers have asked: "Can I get JSON to load into an OrderedDict?" We'll delve into common issues, provide simple solutions, and leave you with a compelling call-to-action that will have you engaging with our community.
Understanding the Problem š The original problem revolves around using an OrderedDict in JSON. While you can utilize an OrderedDict as an input to JSON, the question is whether it can be used as an output to maintain the order of keys in the file. So, let's explore this further.
Exploring Solutions
š ļø Using JSON's dump
method, we can indeed load an OrderedDict into JSON. However, the question remains as to how we can load JSON into an OrderedDict. Luckily, a simple workaround exists.
The Workaround: OrderedDict in JSON
First, we need to import the
json
andcollections
modules:import json import collections
Next, let's create a helper function called
json_load_ordered
, which loads JSON into an OrderedDict:def json_load_ordered(file_name): with open(file_name) as fp: # Pass the `object_pairs_hook` argument to `json.load` return json.load(fp, object_pairs_hook=collections.OrderedDict)
Finally, we can use the
json_load_ordered
function to load JSON into an OrderedDict while preserving the key order:json_data = json_load_ordered('your_file.json')
Explanation and Example
š To better understand the workaround, let's break it down. The json.load
method has an optional parameter called object_pairs_hook
. By passing object_pairs_hook=collections.OrderedDict
as an argument, we ensure that the loaded JSON is transformed into an OrderedDict instead of a regular Python dictionary.
š” Here's a quick example:
Consider a JSON file named your_file.json
with the following content:
{
"name": "John",
"age": 30,
"city": "New York"
}
Using the json_load_ordered
function, we can load this JSON into an OrderedDict:
json_data = json_load_ordered('your_file.json')
print(json_data)
Output:
OrderedDict([('name', 'John'), ('age', 30), ('city', 'New York')])
As you can see, the key order is preserved!
Conclusion and Call-to-Action š Congratulations! We've conquered the challenge of loading JSON into an OrderedDict. By following the simple workaround we provided, you can effortlessly maintain the order of keys in your JSON files.
š¢ We hope this guide was helpful to you! Don't forget to share it with other developers who might encounter similar issues. Feel free to leave a comment below sharing your experiences or any questions you may have. Join our vibrant tech community and stay tuned for more insightful blog posts on our platform!
Now, over to you! Have you encountered any other fascinating JSON-related puzzles? Share them with us and let's unravel them together! š