Are lists thread-safe?
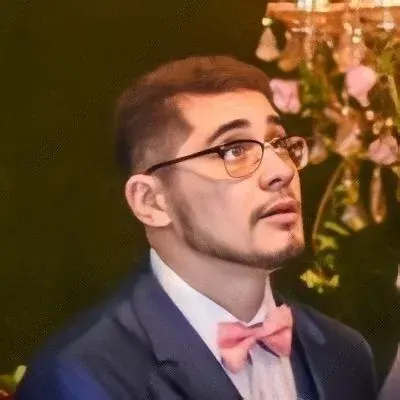
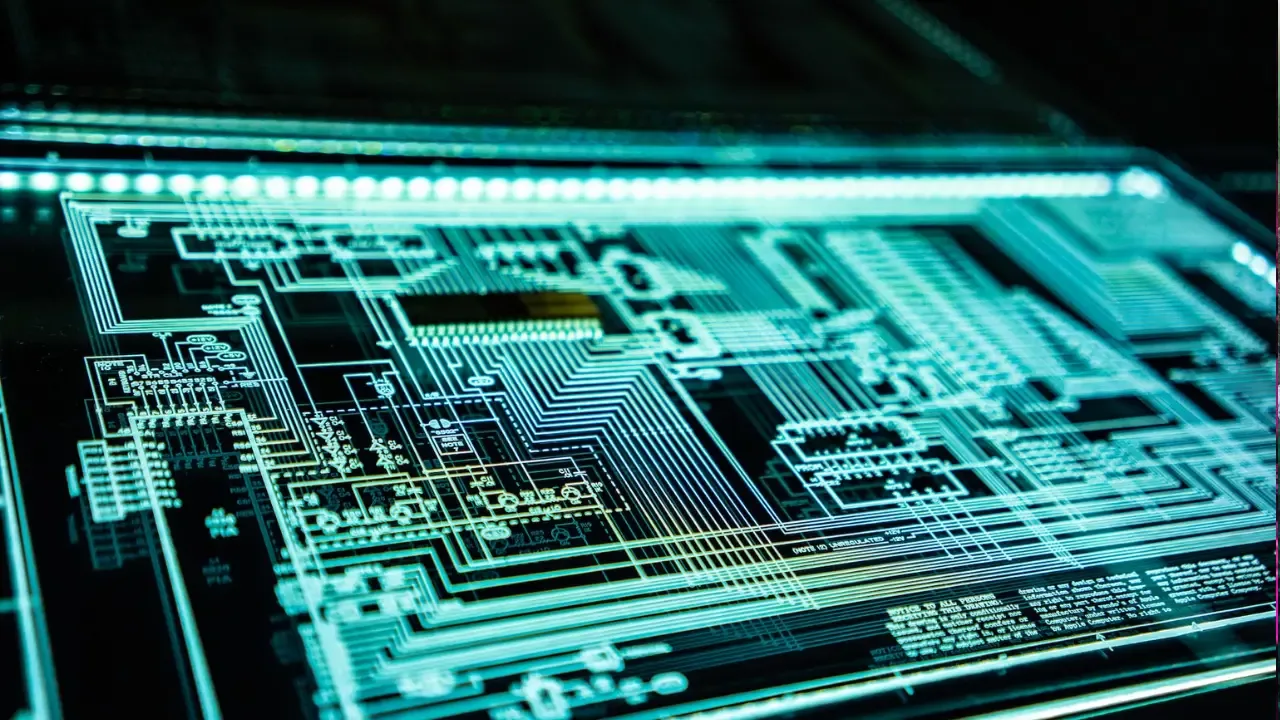
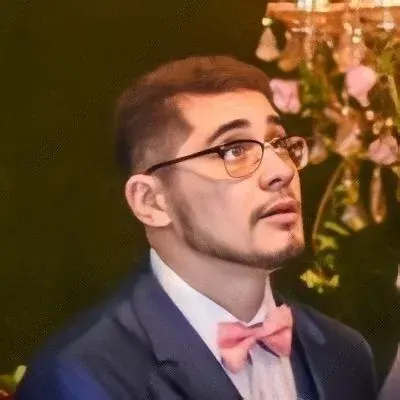
Are lists thread-safe? ๐งต๐ค
If you've ever worked with multiple threads in Python, you may have come across suggestions to use queues instead of lists with the .pop()
method. But why is this recommended? Are lists not thread-safe? ๐ค Let's dive deeper into this subject to unravel the mystery and find easy solutions to common issues! ๐ก
Understanding Thread-Safety ๐
In multi-threaded programming, thread-safety is a crucial concept. It refers to the ability of a program or data structure to handle concurrent access from multiple threads without causing unexpected results or crashing.
The Problem with Lists and .pop()
๐ฑ
By default, lists in Python are not thread-safe. This means that if multiple threads attempt to modify the same list concurrently, it can lead to race conditions or data corruption. ๐ฑ One particular issue arises when using the .pop()
method to remove an item from a list.
Imagine this scenario: Thread A and Thread B both want to pop an item from the same list simultaneously. If there are no synchronization mechanisms in place, both threads could end up popping the same item, leading to erroneous behavior. ๐ซ
Solutions for Thread-Safe Operations ๐ง
1. Use a Lock (Explicit Synchronization) ๐
One way to ensure thread-safety when working with lists is by using a lock. A lock is a synchronization primitive that allows multiple threads to take turns accessing a shared resource.
import threading
my_lock = threading.Lock()
my_list = []
# Thread A
with my_lock:
my_list.pop()
# Thread B
with my_lock:
my_list.pop()
By acquiring the lock before performing the .pop()
operation, we guarantee that only one thread can access the list at a time. This eliminates the risk of race conditions and ensures the integrity of the data.
2. Use a Queue (Implicit Synchronization) ๐
As mentioned in the question, using queues for multi-threaded operations is often recommended alternative to lists. Queues are inherently thread-safe and provide built-in synchronization mechanisms.
import queue
my_queue = queue.Queue()
# Thread A
my_queue.get()
# Thread B
my_queue.get()
With queues, you don't need to worry about explicit synchronization or locks. The underlying queue implementation takes care of ensuring thread-safety for you. You can safely retrieve items without the risk of data corruption or race conditions.
Engage and Share Your Thoughts! ๐ฌ๐
Now that you understand the thread-safety concerns with lists, it's time to take action! Are you facing any issues with lists and threads in your own projects? Or maybe you have some cool tips to share with the community? Let's start a conversation and learn from each other! ๐ช๐ก
๐ฃ Have you ever encountered thread-safety issues with lists in Python? How did you solve them? Share your experiences by leaving a comment below! Let's help each other write better, more efficient code! ๐ฅ๐ค
Remember, sharing is caring! If you found this article useful, don't forget to share it with your fellow developers who might be working with threads and lists. Let's spread knowledge and make code safer together! ๐๐งโ๐ป
Stay tuned for more exciting blog posts in the future! Happy coding! ๐๐