Applying function with multiple arguments to create a new pandas column
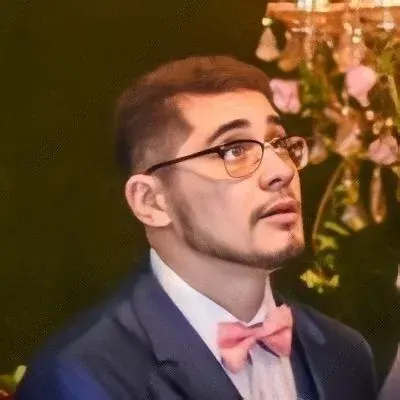
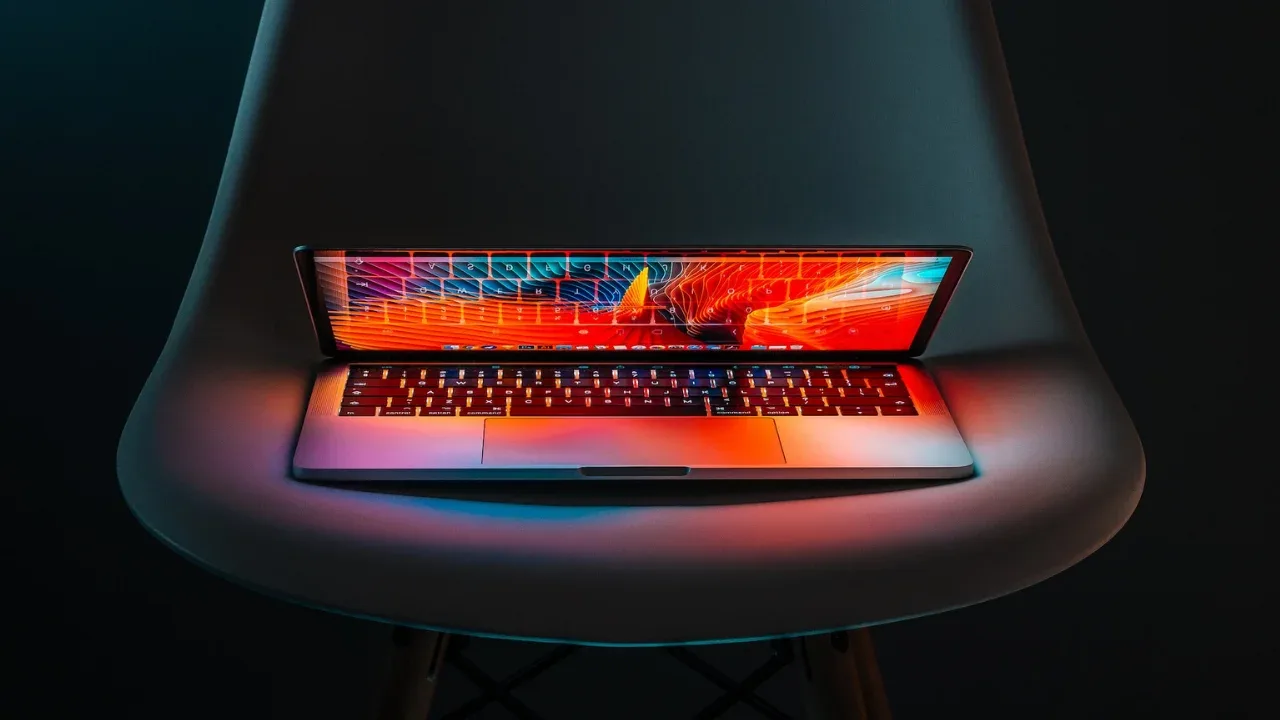
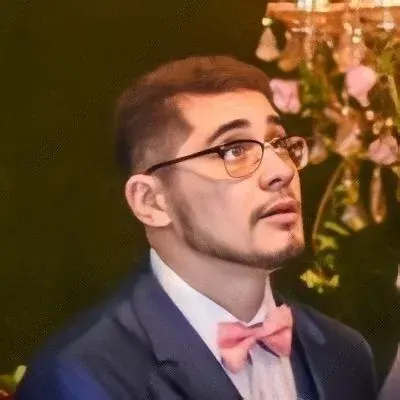
πΌπ Applying a Function with Multiple Arguments to Create a New Pandas Column
Are you struggling to create a new column in a pandas dataframe by applying a function that requires multiple arguments? Don't worry, you're not alone! This is a common challenge faced by many pandas users. In this blog post, we will explore easy solutions to overcome this problem and help you unlock the full potential of pandas. Let's dive in! π
The Challenge: Applying a Function with Multiple Arguments
Let's start by understanding the challenge at hand. In the provided code example, you were able to create a new column based on a function that only required one argument. However, things get a bit tricky when the function requires multiple arguments, like the fxy
function in the code snippet you shared.
def fxy(x, y):
return x * y
The question that arises is: how do we create a new column by passing both column A
and column B
as arguments to this function?
Solution 1: apply
with lambda
function
One way to tackle this challenge is by using the apply
function along with a lambda
function. The lambda
function allows us to create an anonymous function with multiple arguments on-the-fly. Here's how you can apply this solution to your pandas dataframe:
df['newcolumn'] = df.apply(lambda row: fxy(row['A'], row['B']), axis=1)
In the above code, we are using apply
on the dataframe df
along the horizontal axis (axis=1
) to iterate over each row. The lambda
function takes each row as input and applies the fxy
function by passing row['A']
and row['B']
as arguments. The result is stored in the new column named 'newcolumn'
. πͺ
Solution 2: Utilizing zip
and map
Another neat solution involves leveraging the power of zip
and map
functions. We can make use of the zip
function to pair corresponding elements from column A
and column B
and then apply the fxy
function using map
. Let's take a look at the code:
df['newcolumn'] = list(map(fxy, df['A'], df['B']))
In this code, the map
function applies the fxy
function to each pair of elements from column A
and column B
using zip
. The result is returned as a map object, which is then converted to a list and assigned to 'newcolumn'
in the dataframe. π
Engage with Us!
Now that you have learned two easy solutions to create a new column in pandas by applying a function with multiple arguments, it's time to put your knowledge into practice! Head over to your Jupyter Notebook or Python script and give it a try. π»
If you have any questions or need further assistance, feel free to reach out to us in the comments section below. We would love to hear about your experiences and help you out. Happy coding! π
Note: Make sure to import the required libraries (pandas
in this case) before executing the code snippets.
Conclusion
In this blog post, we explored the challenge of applying a function with multiple arguments to create a new pandas column. We provided two easy-to-implement solutions using apply
with lambda
functions and zip
with map
. By applying these techniques, you can now effortlessly create new columns based on your custom functions.
Don't let this challenge slow you down! Embrace these solutions and unlock the full potential of pandas in your data analysis projects. If you found this blog post helpful, share it with your fellow pandas enthusiasts and spread the knowledge. Happy coding! ππΌ