Apply pandas function to column to create multiple new columns?
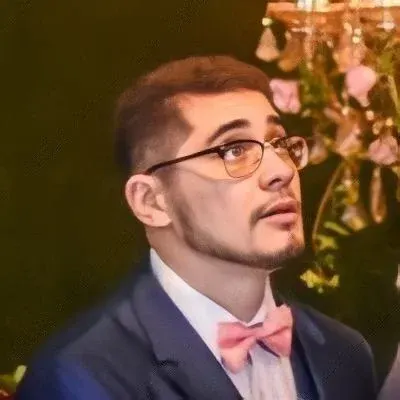
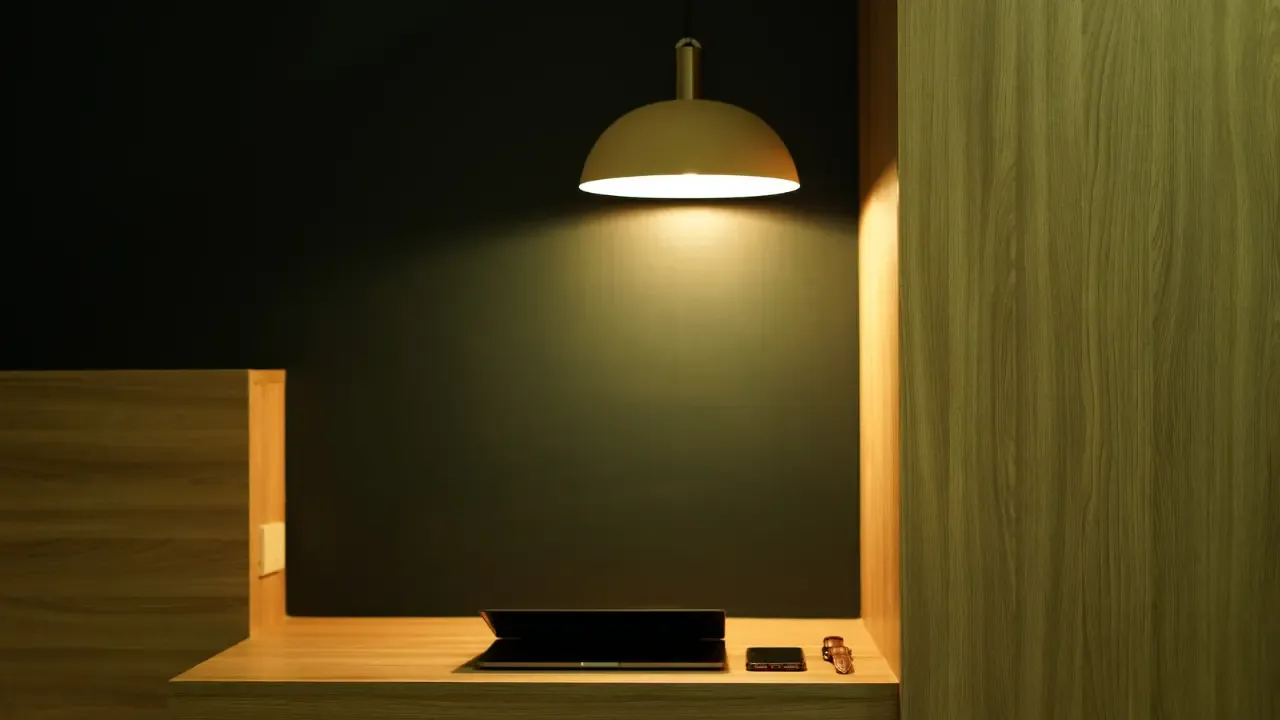
Apply pandas function to column to create multiple new columns? 😕🐼
So you're trying to apply a function to a column in pandas and create multiple new columns as a result? 😮
You're not alone! Many pandas users have faced this common issue, but fret not, my friend! I'm here to guide you through the process and provide you with easy solutions. Let's dive in! 💪💻
The Dilemma 😓
You have a function called extract_text_features
that takes a single text column as input and returns a whopping 6 values as output. You want to apply this function to your pandas DataFrame and assign the output to new columns in the same DataFrame. Seems straightforward, right? Well, not quite! 🤔
The Struggle 😩
You've tried using the map
function like this: df.ix[:,10:16] = df.textcol.map(extract_text_features)
, but something's not right. There's no proper return type that allows you to assign the output correctly. You're left scratching your head, wondering if you should resort to iterating through the DataFrame using df.iterrows()
. But hold your horses! There's a better way! 🙌
The Solution 🎉
Instead of getting tangled in the loops of df.iterrows()
, you can leverage the power of pandas to simplify your task. Here are two simple solutions for you:
Solution 1: Using pd.DataFrame.assign()
Starting from pandas version 0.16.0, the assign
function was introduced. It allows you to easily create new columns and assign them to your DataFrame. Here's how you can use it to solve your problem:
df = df.assign(new_cols=df.textcol.apply(extract_text_features).tolist())
df[['new_col1', 'new_col2', 'new_col3', 'new_col4', 'new_col5', 'new_col6']] = pd.DataFrame(df.new_cols.tolist(), index=df.index)
df.drop('new_cols', axis=1, inplace=True)
First, we create a temporary column called new_cols
, which contains the output of the extract_text_features
function applied to each row.
Next, we create new columns new_col1
, new_col2
, ..., new_col6
by unpacking the temporary column using pd.DataFrame()
and assign it to the corresponding DataFrame columns.
Finally, we drop the temporary column to clean up our DataFrame.
Solution 2: Using df.apply()
with result_type='expand'
Starting from pandas version 1.0.0, the apply
function gained a new result_type
parameter. By setting result_type='expand'
, we can directly expand the output of our function into multiple columns. Here's how you can do it:
df[['new_col1', 'new_col2', 'new_col3', 'new_col4', 'new_col5', 'new_col6']] = df.textcol.apply(lambda x: pd.Series(extract_text_features(x)))
We use the apply
function on the textcol
column and apply a lambda function that converts the output of extract_text_features
into a pandas Series
. This automatically expands the output into separate columns, which we assign to the DataFrame.
Bottom Line 😎
You no longer have to
confuse yourself with df.iterrows()
waste time and energy on splitting functions into separate map(lambda ...)
calls.
With the power of df.assign()
or df.apply()
with result_type='expand'
, you can effortlessly apply a function to a column and create multiple new columns in your pandas DataFrame. 🚀
So go ahead, give these solutions a try, and let us know how they work for you! And remember, if you have any further questions or ideas, feel free to leave a comment below. Happy coding! 😊✨
P.S.
This question was asked in the past, and the answers might not be relevant for the latest pandas versions. Make sure to check the pandas documentation and the latest releases for any improvements or additions to the df.apply()
or df.assign()
functions.📚🔖
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
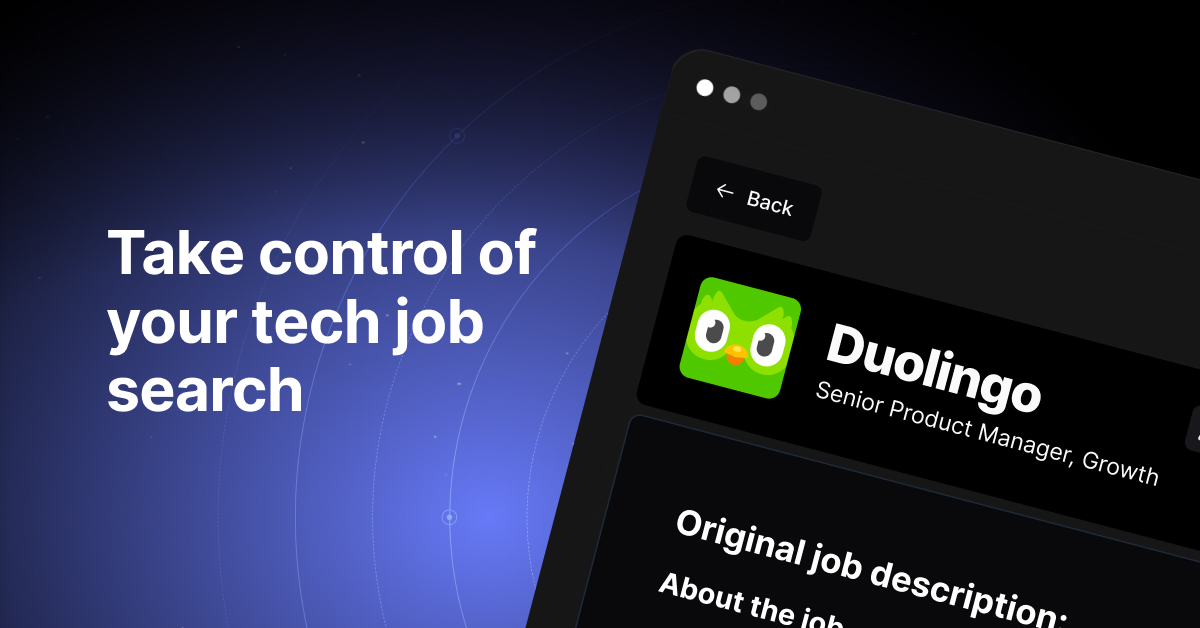