Apply multiple functions to multiple groupby columns
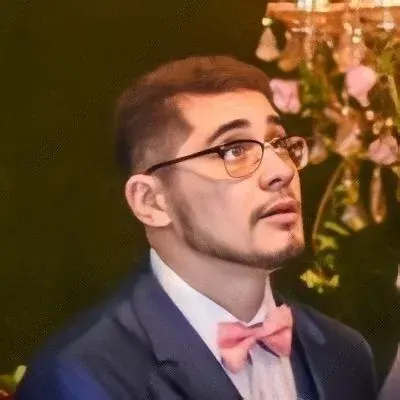
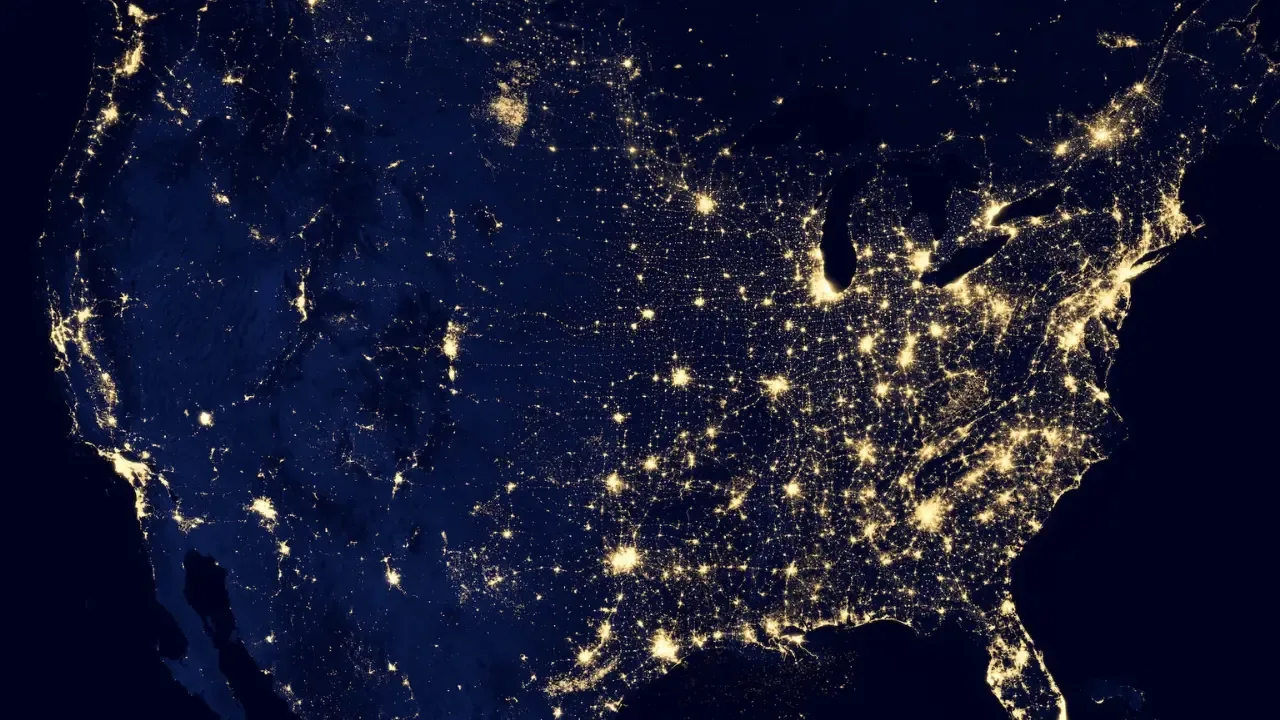
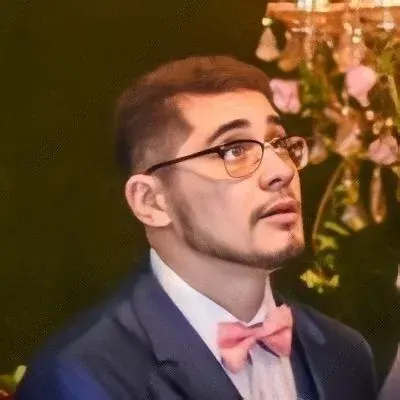
Applying Multiple Functions to Multiple Groupby Columns: A Comprehensive Guide
So, you're trying to apply multiple functions to multiple groupby columns in pandas and you're facing some challenges. Don't worry, you're not alone! In this blog post, we'll explore common issues around this topic, provide easy solutions, and give you a compelling call-to-action to engage with us. Let's dive in! 💪🐼
Understanding the Problem
To set the context, let's take a look at an example from the pandas documentation. The docs demonstrate how to apply multiple functions to a groupby object using a dictionary with the desired output column names as keys. Here's an example:
grouped['D'].agg({'result1': np.sum, 'result2': np.mean})
This works perfectly fine when we have a Series groupby object. However, when we try to apply the same approach to a DataFrame groupby object, things get a bit tricky. The dictionary keys are expected to be column names that the functions will be applied to. This limitation can lead to frustration if we want to perform multiple operations on multiple columns, including operations that depend on other columns within the groupby object.
Easy Solutions
Fear not! We have some easy solutions to address these common issues. Let's explore them one by one:
Solution 1: Iterating through Columns - The Traditional Way
One way to tackle this problem is to go column by column and apply the desired functions. Here's an example:
grouped.agg({'C_sum': lambda x: x['C'].sum(),
'C_std': lambda x: x['C'].std(),
'D_sum': lambda x: x['D'].sum(),
'D_sumifC3': lambda x: x['D'][x['C'] == 3].sum()})
While this approach may work, it can be time-consuming as we iterate through the groupby object multiple times. 😴
Solution 2: Expanding on Solution 1 - Leveraging Other Columns
To overcome the limitations of Solution 1, we can use lambdas and include functions that depend on other columns within the groupby object. Here's an example:
grouped.agg({'C_sum': lambda x: x['C'].sum(),
'C_std': lambda x: x['C'].std(),
'D_sum': lambda x: x['D'].sum(),
'D_sumifC3': lambda x: x['D'][x['C'] == 3].sum(),
...
})
However, keep in mind that this approach will lead to a KeyError since the keys must be columns when using agg()
on a DataFrame.
Solution 3: A Cleaner Approach with Transform
Now, let's introduce you to a built-in pandas function that can handle your requirements in a cleaner way - transform()
. This function can perform group-wise operations and maintain the shape of the original DataFrame. Here's an example:
grouped[['C', 'D']].transform(lambda x: x.sum())
In the above example, we are applying the sum()
function to both the 'C' and 'D' columns within the groupby object. You can replace sum()
with any other function as per your requirements.
By leveraging transform()
, you can apply multiple functions to multiple columns in a single run without the need for iterative operations. 🚀
Engage with Us!
We hope these solutions have helped you overcome the challenges of applying multiple functions to multiple groupby columns. Now, it's your turn to engage with us!
📢 Share your thoughts: Have you faced similar issues in your pandas projects? How did you solve them? Share your experiences and insights in the comments section below.
💌 Subscribe to our newsletter: Never miss an update on the latest pandas tips, tricks, and best practices. Subscribe to our newsletter to stay ahead of the curve.
🚀 Join our community: Connect with like-minded data enthusiasts in our vibrant and supportive community. Participate in discussions, ask questions, and share your knowledge to help others grow.
That's it for now! We hope you found this guide helpful and engaging. Remember, pandas is a powerful tool, and with a little creativity, you can overcome any hurdle. Happy coding! 😊🐼