">", "<", ">=" and "<=" don"t work with "filter()" in Django
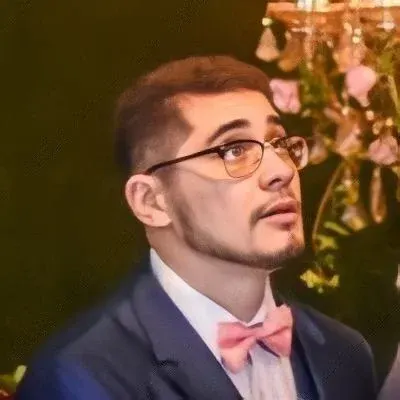
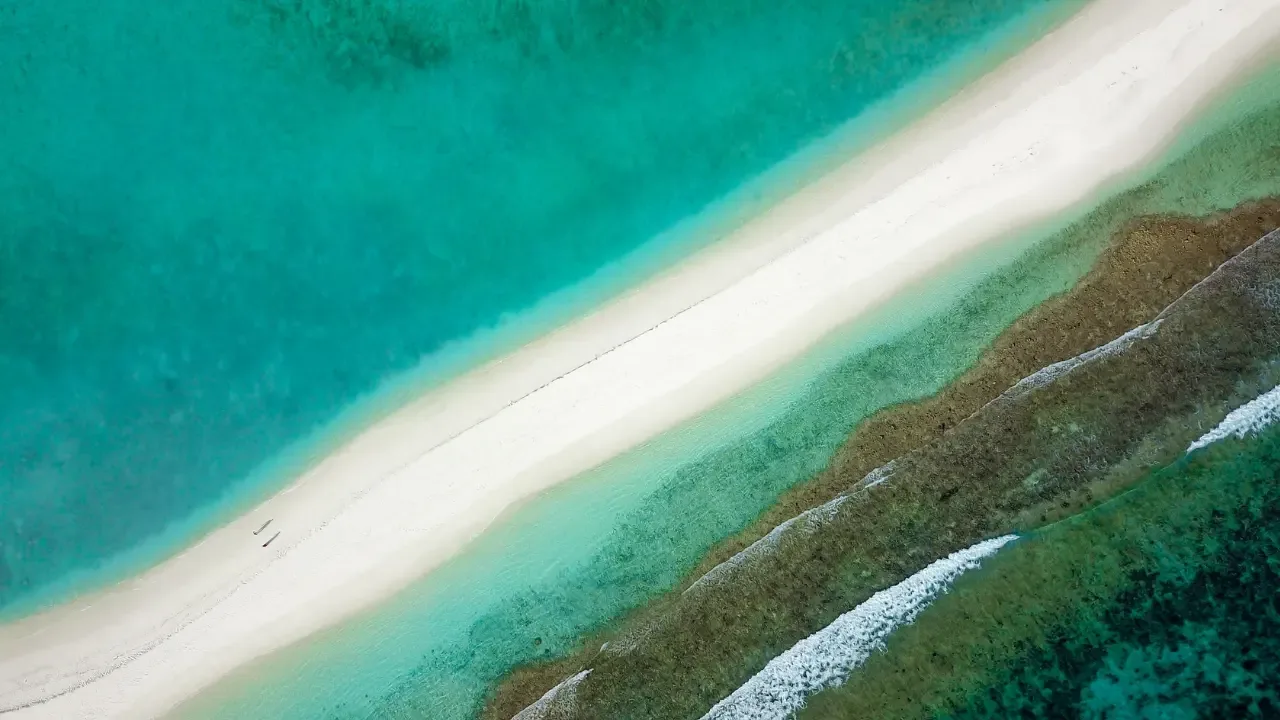
The Django "filter()" Dilemma: Greater Than, Lesser Than, and Equality
š Hey there, tech enthusiasts! Welcome to another exciting blog post where we're going to tackle a common issue in Django's filter()
method. Are you ready to dive into the world of filtering objects based on greater than, lesser than, and equality conditions? Let's go! š
The Challenge: Filtering with ">", "<", ">=", and "<=" in Django
So, you are working on your Django project, and you need to filter objects based on specific conditions using the filter()
method. Filtering by equality? Easy peasy! Just use the equals sign (=
) like this:
qs = Person.objects.filter(age=20)
ā
Great! Filtering by age equals 20 works like a charm! But what about greater than (>
), lesser than (<
), greater than or equal to (>=
), and lesser than or equal to (<=
)? š
qs = Person.objects.filter(age > 20)
# ā Here
qs = Person.objects.filter(age < 20)
# ā Here
qs = Person.objects.filter(age >= 20)
# āā Here
qs = Person.objects.filter(age <= 20)
# āā Here
ā Uh-oh! Suddenly, mystery strikes and you encounter the dreaded "NameError: name 'age' is not defined" error.
The Explanation: Understanding the Problem
The reason behind the error is subtle but crucial to grasp. The issue lies in using comparison operators (>
, <
, >=
, and <=
) directly within the filter()
method. Django's ORM (Object-Relational Mapping) requires a slightly different syntax for expressing these conditions.
The Solution: Django's "Q" Object to the Rescue
Fear not, my friend! Django has a powerful tool called the "Q" object, which allows us to construct complex queries using logical operators. Let's see how we can solve the filtering conundrum! š
Import the Q object:
from django.db.models import Q
Use the Q object with relevant syntax to create the desired conditions:
Filtering by greater than (
>
):qs = Person.objects.filter(age__gt=20) # ā Here
Filtering by lesser than (
<
):qs = Person.objects.filter(age__lt=20) # ā Here
Filtering by greater than or equal to (
>=
):qs = Person.objects.filter(age__gte=20) # āā Here
Filtering by lesser than or equal to (
<=
):qs = Person.objects.filter(age__lte=20) # āā Here
Voila! Your queries are now filtering based on the desired conditions! š
Taking It a Step Further: Combining Conditions
But wait, there's more! The beauty of the "Q" object is its incredible versatility. You can also combine multiple conditions in complex ways using logical operators like AND
, OR
, and NOT
.
Combining conditions:
qs = Person.objects.filter(Q(age__gt=20) & Q(name__icontains='John')) # āāā Here āāāāā Here
Here, we're filtering for persons with an age greater than 20 AND a name containing "John" (case-insensitive).
The Call-to-Action: Share Your Input!
Now that you've learned how to use the "Q" object for filtering in Django, it's time to put your new skills to the test! Give it a go in your projects and share your experiences with us. Have you encountered any other Django filtering challenges? Let us know in the comments below! Let's engage in a tech chat! š¬š„
Thanks for joining us today! Stay tuned for more amazing tech insights, tips, and tutorials. Until next time, happy coding! š»āØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
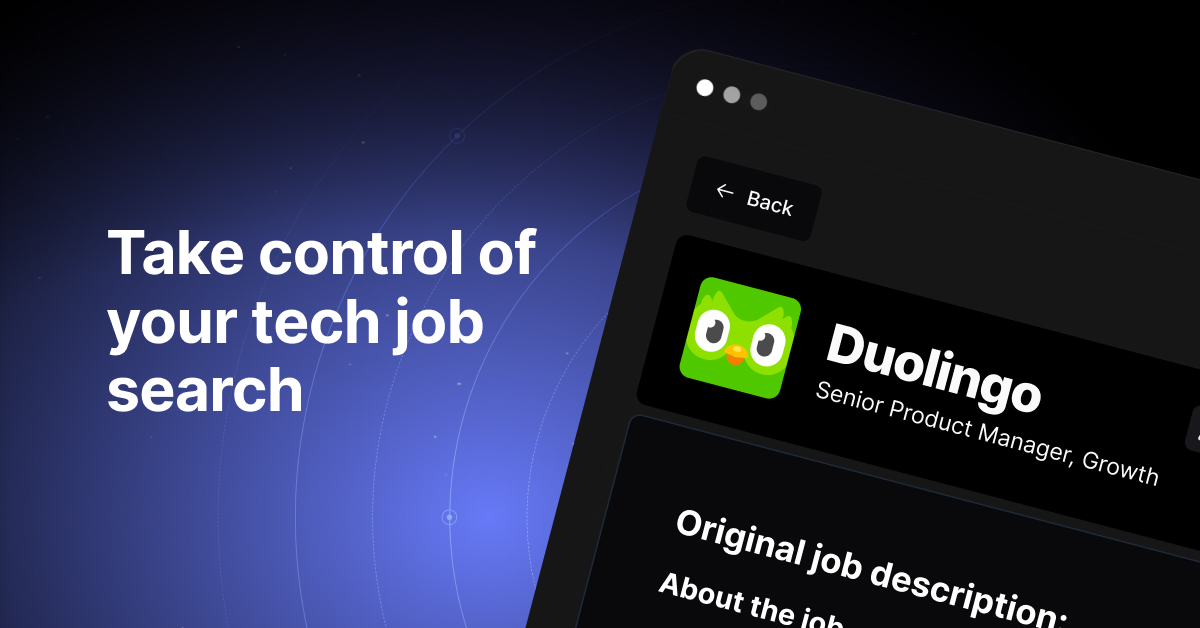