Alternatives for returning multiple values from a Python function
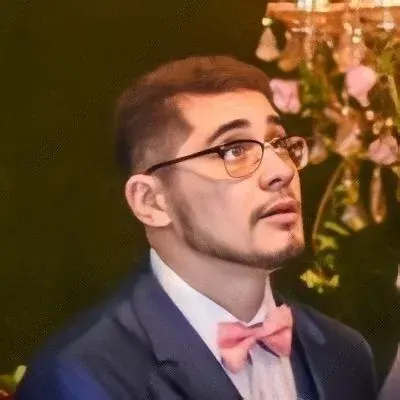
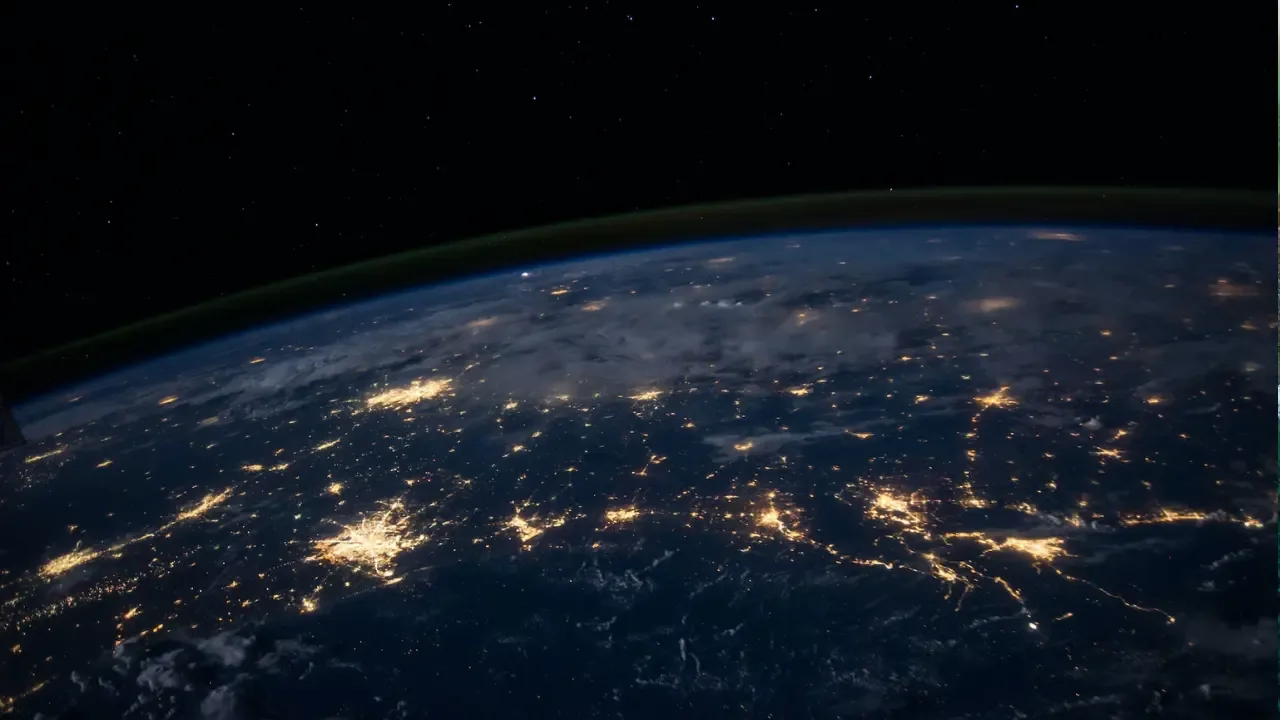
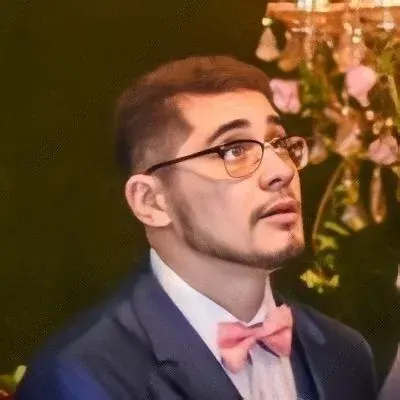
Alternatives for Returning Multiple Values from a Python Function
Have you ever encountered a situation where you needed to return multiple values from a Python function? 🤔 It can be a bit trickier than expected, but fear not! In this blog post, we'll explore different options and discuss their pros and cons. By the end, you'll have a clear understanding of the alternatives available and be able to choose the best approach for your specific use case. Let's dive in! 💪
Option 1: Using a Tuple
The most common way to return multiple values in Python is by using a tuple. 👥 Consider the following example:
def f(x):
y0 = x + 1
y1 = x * 3
y2 = y0 ** y3
return (y0, y1, y2)
This approach works well when you have a small number of values to return. However, things can quickly get messy when the number of values increases. Remembering the order in which the values were packed and unpacking them can become challenging. 😫
Option 2: Using a Dictionary
To overcome the limitations of using a tuple, you can use a dictionary to return multiple values. 📚 Consider the following example:
def g(x):
y0 = x + 1
y1 = x * 3
y2 = y0 ** y3
return {'y0': y0, 'y1': y1 ,'y2': y2}
Using a dictionary allows you to access specific values by their keys, making the code more readable and easier to understand. For example, result['y0']
gives you the value of y0
.
Option 3: Using a Class
Another alternative is to return a specialized structure, such as a class. 🏗️ This approach provides more structure and flexibility. Here's an example:
class ReturnValue:
def __init__(self, y0, y1, y2):
self.y0 = y0
self.y1 = y1
self.y2 = y2
def g(x):
y0 = x + 1
y1 = x * 3
y2 = y0 ** y3
return ReturnValue(y0, y1, y2)
In Python, using a class is similar to using a dictionary. However, it provides additional features, such as defining methods and utilizing the __slots__
attribute for memory optimization.
Option 4: Using a Dataclass (Python 3.7+)
If you're using Python 3.7 or newer, you can take advantage of the dataclass
feature to simplify the process even further. 🎉 Here's an example:
@dataclass
class Returnvalue:
y0: int
y1: float
y3: int
def total_cost(x):
y0 = x + 1
y1 = x * 3
y2 = y0 ** y3
return ReturnValue(y0, y1, y2)
dataclass
automatically generates special methods, adds typing annotations, and provides other useful tools, saving you time and effort.
Option 5: Using a List
Although not commonly recommended, you can also use a list to return multiple values from a function. 📜 Here's an example:
def h(x):
result = [x + 1]
result.append(x * 3)
result.append(y0 ** y3)
return result
Using a list in this way doesn't offer many advantages over using a tuple. It's best to follow the convention of using lists for multiple elements of the same type and tuples for a fixed number of elements with predetermined types – a convention borrowed from functional programming. 🌟
💡 The Best Method?
After discussing various alternatives, you might be wondering which method is the best. 🤔 The answer depends on your specific requirements and preferences. Each option has its advantages, so consider factors like code readability, ease of use, and performance when making your decision.
Now It's Your Turn!
Have you faced the challenge of returning multiple values from a Python function? Which method did you find most effective? 🚀 Share your thoughts and experiences in the comments below!
Remember, the ultimate goal is to choose an approach that best suits your needs. Whether it's using a tuple, a dictionary, a class, a dataclass, or a list, make sure your code is clean, maintainable, and easy to understand.
Thanks for reading! If you found this blog post helpful, don't forget to share it with your fellow Pythonistas. Together, let's make Python programming a breeze! ✨🐍💻