Alphabet range in Python
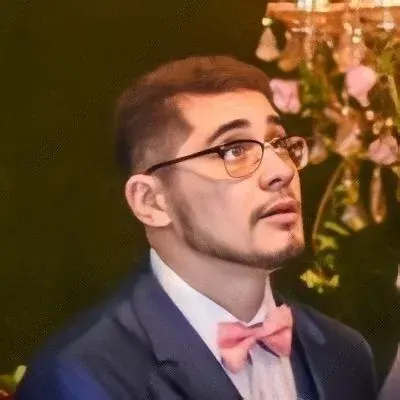
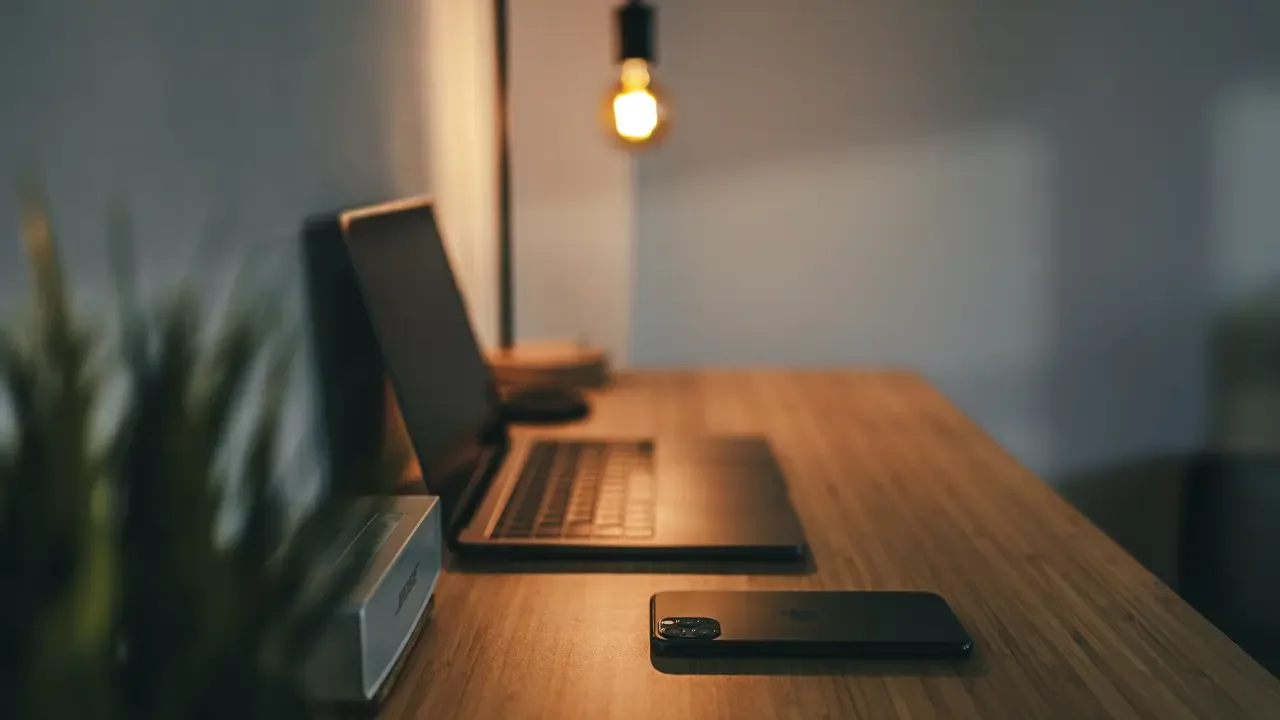
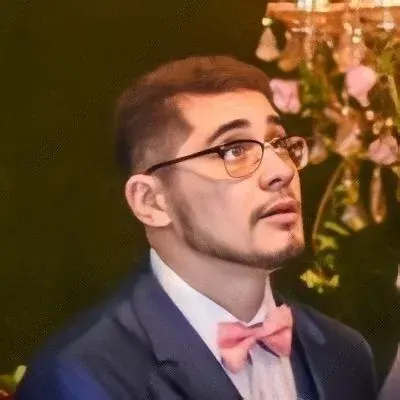
🌟 A Handy Guide to Creating an Alphabet Range in Python 📚
Are you tired of manually typing out each letter of the alphabet in Python? We've got you covered! In this post, we'll show you an easy and efficient way to create a list of alphabet characters without all the hassle. Let's dive in! 💪
The Problem 😫
So you want to create a list of alphabet characters, but doing it manually like this is just too time-consuming and tedious:
['a', 'b', 'c', 'd', ..., 'z']
Surely, there must be a better way, right? 😓
The Solution 💡
Fortunately, Python has a built-in module called string
that we can leverage to simplify the process. The string
module provides a constant called ascii_lowercase
which contains all the lowercase letters of the alphabet.
Here's how you can use it to create an alphabet range:
import string
alphabet = list(string.ascii_lowercase)
print(alphabet)
🎉 And just like that, you have a list alphabet
containing all 26 lowercase letters of the alphabet! So easy, right?
Handling Different Cases 🆒
But what if you need the list to include uppercase letters as well? No worries! We can leverage the string.ascii_uppercase
constant to achieve this. Here's how:
import string
alphabet = list(string.ascii_lowercase + string.ascii_uppercase)
print(alphabet)
Now, the alphabet
list includes both lowercase and uppercase letters. Perfect! 👌
Bonus Tip 🌟
What if you want to create an alphabet range with a custom starting point? Let's say you want to start from the letter 'c' instead of 'a'. No problemo! We can use slicing to achieve this. Here's an example:
import string
alphabet = list(string.ascii_lowercase[string.ascii_lowercase.index('c'):])
print(alphabet)
This returns a list starting from 'c': ['c', 'd', ..., 'z']
. Feel free to modify the starting point as needed!
Call-to-Action: Time to Get Creative! 🎨
Now that you know how to create an alphabet range in Python without all the manual typing, it's time to explore the possibilities. 🌈
Challenge yourself by:
Creating a function that accepts a starting and ending point and returns the corresponding alphabet range.
Implementing a program that encrypts a message using the alphabet range.
Designing an interactive game where players have to guess the next letter in the alphabet sequence.
The sky's the limit! Share your creations with the Python community and let's inspire each other. ✨
Remember, the power of code is in your hands! 🚀
Conclusion 📝
Creating an alphabet range in Python no longer needs to be a chore. By leveraging the string
module, we can effortlessly generate a list of alphabet characters, whether in lowercase, uppercase, or with a custom starting point.
Now, it's your turn to take what you've learned and let your imagination run wild! 💡
So go ahead, break free from the confines of manual typing and unlock the true potential of your Python code! Happy coding! 😄
Don't forget to share this post with your friends and fellow Python enthusiasts. Let's spread the word and make coding more accessible to everyone. 🙌✨
Got any questions or insights? Leave a comment below and let's start a conversation! 👇🗨️