Add x and y labels to a pandas plot
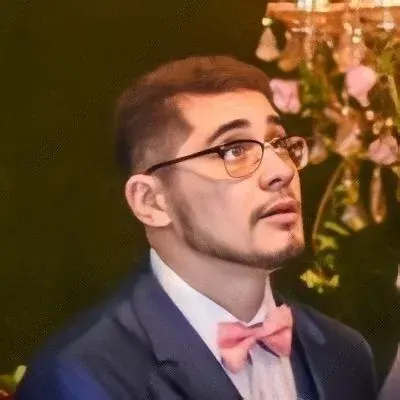
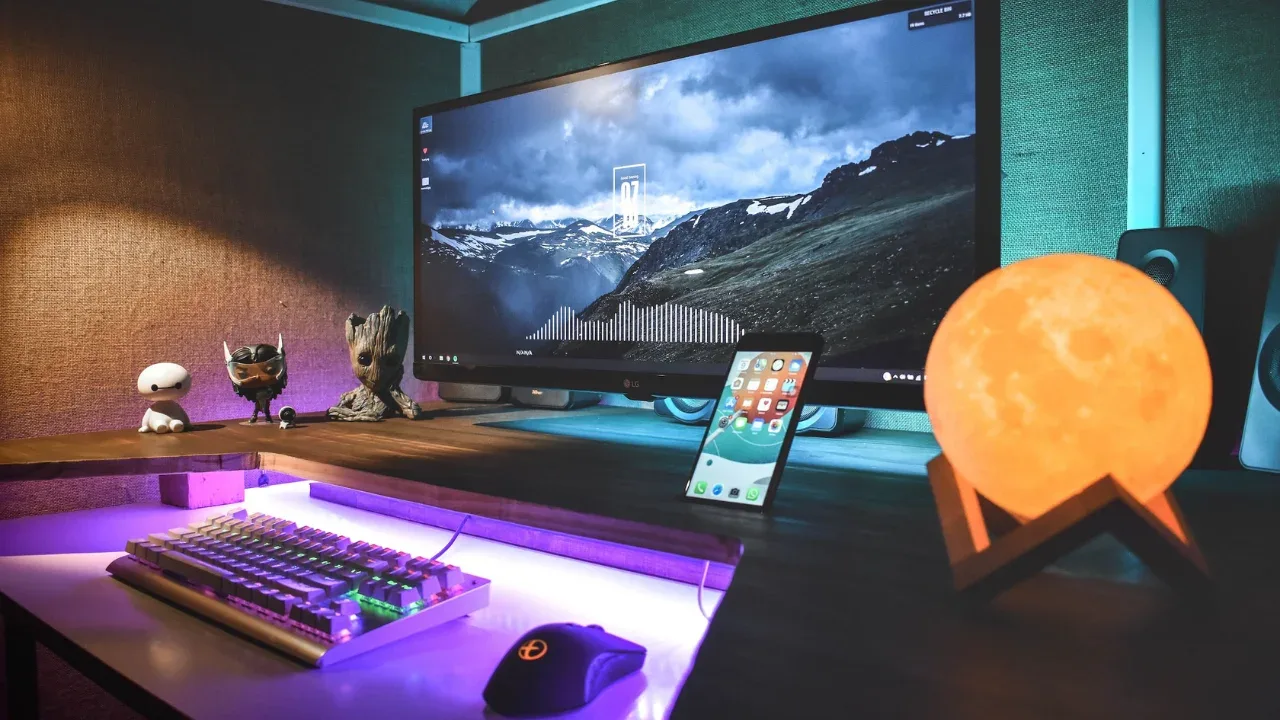
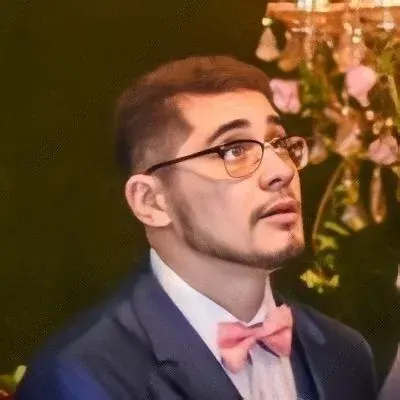
How to Add x and y Labels to a Pandas Plot
So you've created a simple plot using the plot()
function in pandas, but you realized that you need to add x and y labels to make it more informative. You also want to preserve the specific colormaps you're using. Don't worry, I've got you covered! 📊📝
The Problem
When using the plot()
function in pandas to create a plot, you may notice that there are no specific parameters for adding x and y labels. This can be frustrating when you want to provide context to your plot. But fear not, there are easy solutions available!
Solution 1: Use Matplotlib
One way to add x and y labels to your pandas plot is to make use of the underlying Matplotlib library. Here's how you can do it:
import pandas as pd
import matplotlib.pyplot as plt
# Your dataframe and plot code
values = [[1, 2], [2, 5]]
df2 = pd.DataFrame(values, columns=['Type A', 'Type B'],
index=['Index 1', 'Index 2'])
# Plotting using pandas
df2.plot(lw=2, colormap='jet', marker='.', markersize=10,
title='Video streaming dropout by category')
# Adding x and y labels using Matplotlib
plt.xlabel('X Label')
plt.ylabel('Y Label')
# Display the plot
plt.show()
By importing matplotlib.pyplot
as plt
, you gain access to additional functionality to customize your plot. Here, we use the xlabel()
and ylabel()
functions to add the desired labels to our plot. Finally, we call plt.show()
to display the plot with the added labels.
Solution 2: Use Pandas Styling
Another way to add x and y labels without the need for external libraries is to use pandas styling capabilities. Here's how you can do it:
import pandas as pd
# Your dataframe and plot code
values = [[1, 2], [2, 5]]
df2 = pd.DataFrame(values, columns=['Type A', 'Type B'],
index=['Index 1', 'Index 2'])
# Plotting using pandas
ax = df2.plot(lw=2, colormap='jet', marker='.', markersize=10,
title='Video streaming dropout by category')
# Adding x and y labels using pandas styling
ax.set_xlabel('X Label')
ax.set_ylabel('Y Label')
# Display the plot
plt.show()
Here, we assign the plot object returned by df2.plot()
to ax
. Then, we use set_xlabel()
and set_ylabel()
methods on ax
to add x and y labels respectively. Finally, we call plt.show()
to display the plot with the added labels.
Conclusion
Adding x and y labels to a pandas plot is essential for providing context and making your plot more informative. You can achieve this by using Matplotlib or pandas styling capabilities. So go ahead, add those labels and make your plots shine! 🌟
How do you usually add x and y labels to your pandas plots? Did you find these solutions helpful? Let us know in the comments below! And don't forget to share this post if you found it useful. Happy plotting! 💪💻