Accessing the index in "for" loops
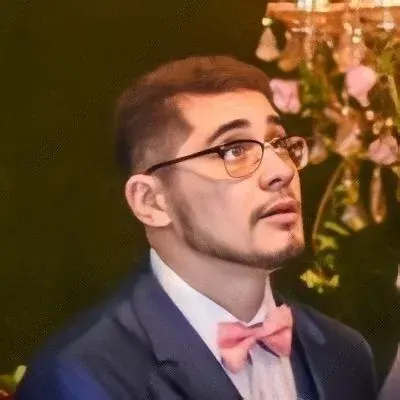
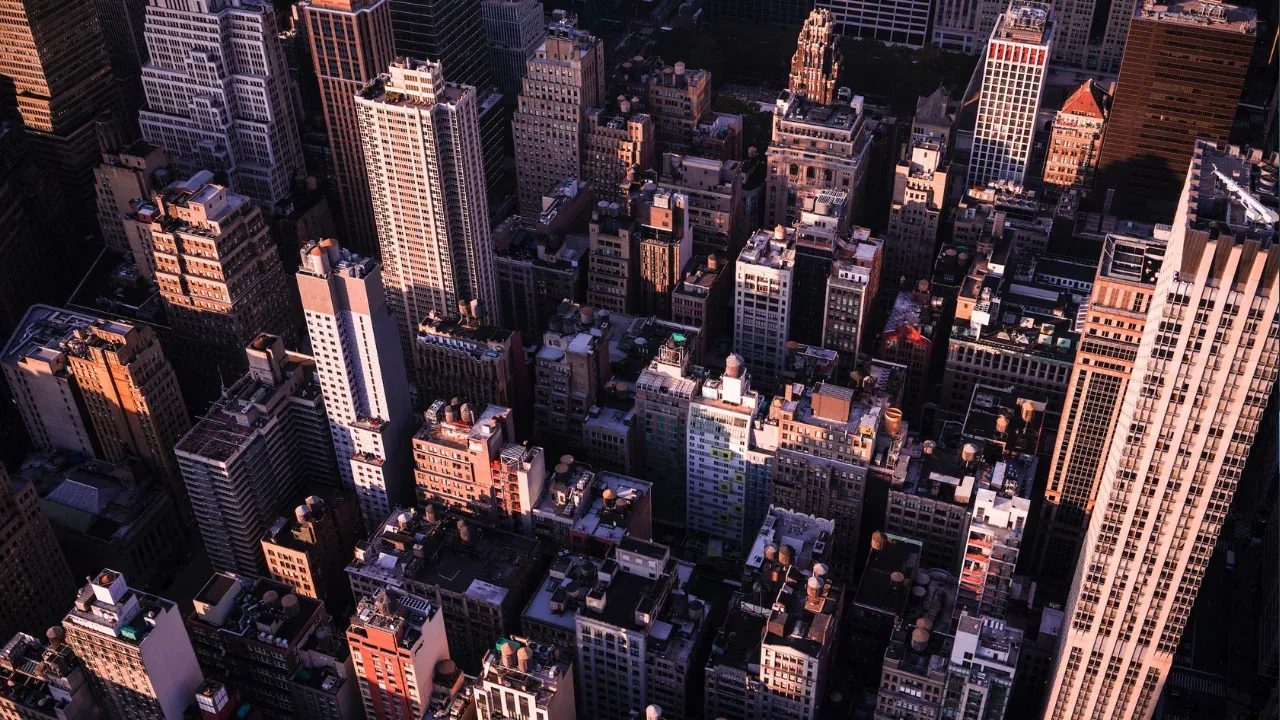
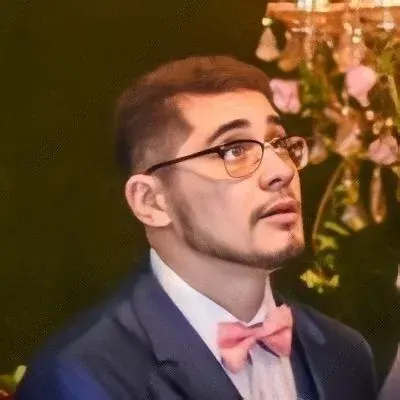
Blog Title: "Unleash the Power of the Index: Accessing the Index in 'for' Loops"
š Accessing the index while iterating over a sequence in a for loop is a common challenge for many programmers. Whether you want to print a message with the index or perform some specific operations based on the index, knowing how to access it is crucial. In this blog post, we will explore the best practices for accessing the index in for loops and provide simple solutions to help you conquer this hurdle. Let's dive in! šŖ
The Challenge
š§ Imagine you have a sequence, let's say a list of numbers just like in the example:
xs = [8, 23, 45]
for x in xs:
print("item #{} = {}".format(index, x))
šÆ The desired output is:
item #1 = 8
item #2 = 23
item #3 = 45
š Notice how we want to include the index in the printed message. However, running this code as is will only print the values without the corresponding indices. So, how can we access the index to achieve the desired result? Let's find out!
The Solution
⨠The key to accessing the index in a for loop is by using the built-in enumerate()
function. The enumerate()
function returns an iterator that provides both the index and the value of each item in the sequence. Here's how we can modify our code to achieve the desired output:
xs = [8, 23, 45]
for index, x in enumerate(xs, start=1):
print("item #{} = {}".format(index, x))
š By using the enumerate()
function, we can assign the index to the index
variable and the value to the x
variable in each iteration. The start
parameter allows us to specify the starting index value (in our case, 1) instead of the default 0.
Additional Tips and Tricks
š Here are some additional tips and tricks to make your index-accessing journey even smoother:
1. Reversing the Order of Iteration
š If you need to iterate over the sequence in reverse order while still accessing the index, you can use the enumerate()
function in conjunction with the reversed()
function. Here's an example:
xs = [8, 23, 45]
for index, x in reversed(list(enumerate(xs, start=1))):
print("item #{} = {}".format(index, x))
š By wrapping enumerate()
with reversed()
and converting it to a list, we can iterate over the sequence in reverse order while preserving the index.
2. Skipping Some Elements
āļø If you want to skip some elements while iterating over the sequence, you can use the continue
statement. Here's an example:
xs = [8, 23, 45]
for index, x in enumerate(xs, start=1):
if index == 2:
continue
print("item #{} = {}".format(index, x))
š In this example, the second element with the index 2 will be skipped because of the continue
statement. You can customize this condition to skip any specific elements based on your requirements.
Wrap Up
š Accessing the index in a for loop doesn't have to be a daunting task anymore. By utilizing the enumerate()
function, you can effortlessly access both the index and value of each item in the sequence. Remember to use the start
parameter to control the starting index value.
š” Whether you want to print formatted messages, perform calculations, or implement specific logic based on the index, mastering this technique will enhance your programming skills.
š Now it's your turn! Try applying the enumerate()
function in your own code and see the magical results. Share your experience and any questions or doubts in the comments section below. Let's continue this coding journey together! š