Accessing items in an collections.OrderedDict by index
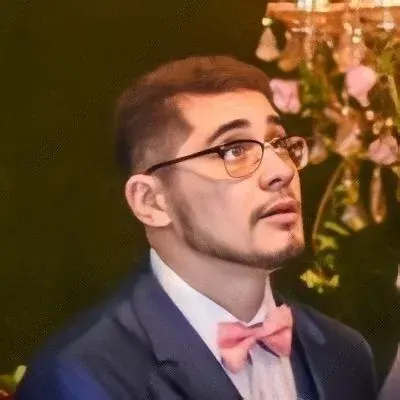
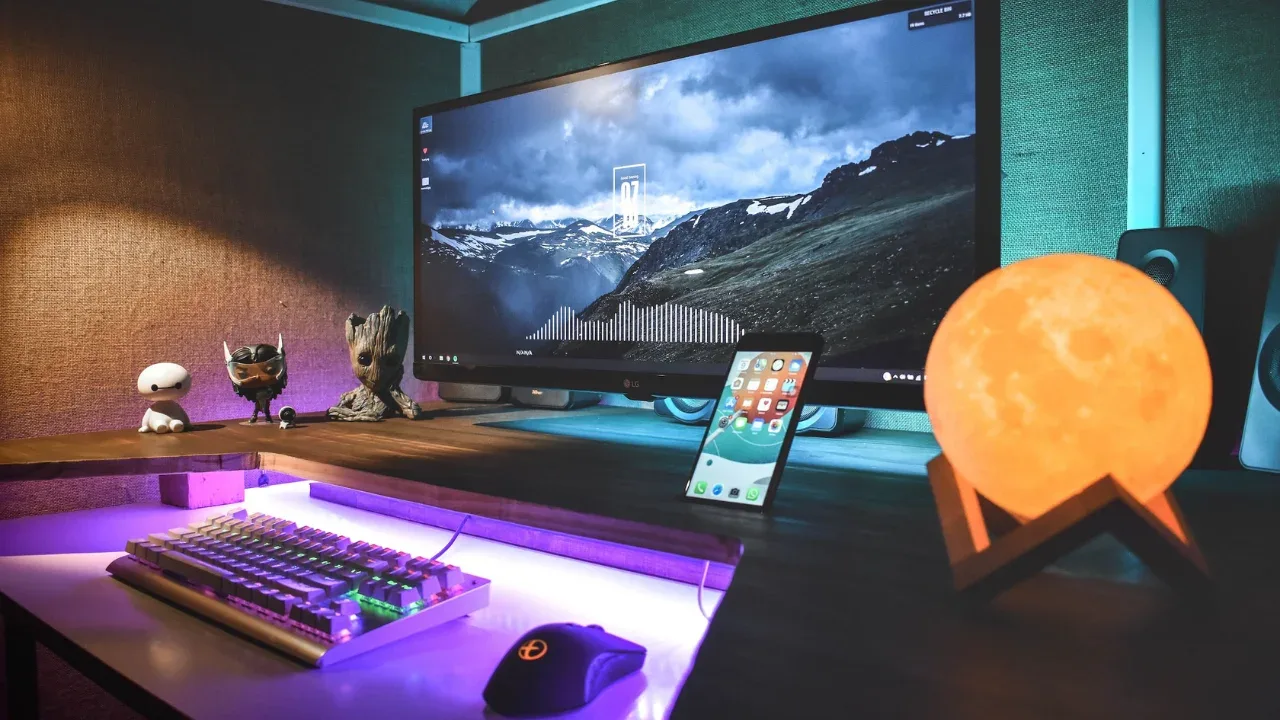
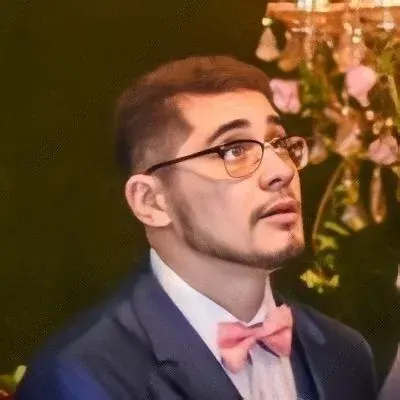
Accessing items in an collections.OrderedDict
by index
Are you struggling with accessing specific items from an OrderedDict
in Python? 🤔 No worries, I've got you covered! In this blog post, I'll walk you through the problem, provide easy solutions, and make sure you leave with a smile on your face. Let's dive right in! 💪
The Problem
Imagine you have the following code:
import collections
d = collections.OrderedDict()
d['foo'] = 'python'
d['bar'] = 'spam'
You might be wondering if there's a way to access the items in a numbered manner, like d(0)
for foo
and d(1)
for bar
. Unfortunately, OrderedDict
does not directly support indexing like a list or tuple. 😕
Solution 1: Convert the OrderedDict to a list
One way to tackle this problem is by converting your OrderedDict
into a list. Here's how you can do it:
import collections
d = collections.OrderedDict()
d['foo'] = 'python'
d['bar'] = 'spam'
# Convert OrderedDict to a list
items = list(d.items())
# Access items by index
print(items[0]) # ('foo', 'python')
print(items[1]) # ('bar', 'spam')
By using the items()
method of the OrderedDict
, we can easily convert it into a list of tuples. Then, we can access individual items using the index position of the list. 📜
Solution 2: Implement a helper function
If you find yourself frequently needing to access items by index in an OrderedDict
, you might prefer to create a helper function. Here's an example:
import collections
d = collections.OrderedDict()
d['foo'] = 'python'
d['bar'] = 'spam'
def get_item_by_index(od, index):
return list(od.items())[index]
# Access items using the helper function
print(get_item_by_index(d, 0)) # ('foo', 'python')
print(get_item_by_index(d, 1)) # ('bar', 'spam')
By encapsulating the conversion and index retrieval logic within a function, you can easily fetch items by their index without repeating the same code. 🧩
Feeling Adventurous? Try Solution 3 with a custom subclass!
For those who love exploring advanced Python concepts, you can even create a custom subclass of collections.OrderedDict
to directly support indexing. While this approach requires a bit more coding, it offers a convenient way to access items. Here's a sample implementation:
import collections
class MyOrderedDict(collections.OrderedDict):
def __call__(self, index):
return list(self.items())[index]
d = MyOrderedDict()
d['foo'] = 'python'
d['bar'] = 'spam'
print(d(0)) # ('foo', 'python')
print(d(1)) # ('bar', 'spam')
In this solution, we define a new method __call__()
in our custom MyOrderedDict
class. This method allows us to use d(index)
syntax to retrieve items by their index directly. 🎩
Conclusion
Today, we learned three different solutions to access items in an collections.OrderedDict
by index. We covered converting the OrderedDict
to a list, implementing a custom helper function, and creating a custom subclass.
Now it's your turn! 💡 Which solution caught your attention the most? Are you planning to give it a try? Let me know in the comments section below, and don't forget to share this post with your fellow Pythonistas. Happy coding! 🐍✨