Accessing dict_keys element by index in Python3
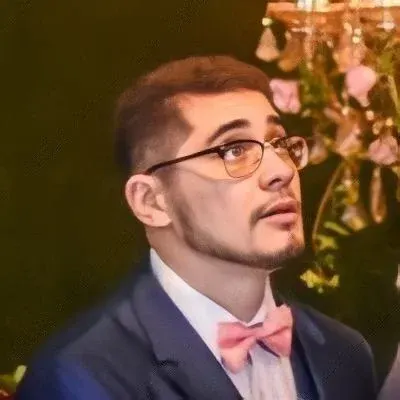

Accessing dict_keys element by index in Python3: Easy Solutions 🐍
Have you ever found yourself in a situation where you need to access a specific element from a dictionary using its index? You're not alone! 🤔
In Python, dictionaries are incredibly powerful data structures that allow you to map keys to values. However, one limitation of dictionaries is that they don't support indexing directly like lists or tuples do. But fear not! We have got you covered with some easy solutions to help you access elements from dict_keys objects by index. 😎
Understanding the Problem
Before diving into the solutions, let's understand why you encountered the errors:
test = {'foo': 'bar', 'hello': 'world'}
keys = test.keys() # dict_keys object
keys.index(0)
AttributeError: 'dict_keys' object has no attribute 'index'
keys[0]
TypeError: 'dict_keys' object does not support indexing
In the above code snippet, test.keys()
returns a dict_keys object, which represents a dynamic view of the dictionary's keys. Since dict_keys objects aren't sequences, you cannot directly access elements by index or use the index()
method. 😕
Solution 1: Convert dict_keys to a List 📜
A simple solution to this problem is to convert the dict_keys object into a list. Python provides the list()
function that can convert any iterable, including dict_keys, to a list.
test = {'foo': 'bar', 'hello': 'world'}
keys = list(test.keys()) # Convert dict_keys to a list
keys[0] # 'foo'
By using the list()
function to convert dict_keys
to a list, you can access elements using the index without any hassle. 👍
Solution 2: Using the itertools Module 🌀
If you'd rather avoid creating a new list, you can use the islice()
function from the itertools
module to access elements from dict_keys by index. The islice()
function allows you to slice an iterator without the need to convert it into a list or any other sequence type.
Here's how you can use islice()
to access elements from dict_keys:
from itertools import islice
test = {'foo': 'bar', 'hello': 'world'}
keys = test.keys() # dict_keys object
next(islice(keys, 0, None)) # 'foo'
In the code above, islice()
allows you to slice the dict_keys
object and the next()
function returns the first element from the sliced iterator.
Conclusion and Call-to-Action
Although dictionaries don't natively support indexing, you now have two easy solutions at your fingertips! Whether you choose to convert the dict_keys object into a list or utilize the itertools module, accessing elements by index is now a breeze. 🎉
Next time you encounter this issue, try one of these solutions and let us know how it worked for you! Feel free to share your thoughts, questions, or any other Python-related topic in the comments section below. Happy coding! 💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
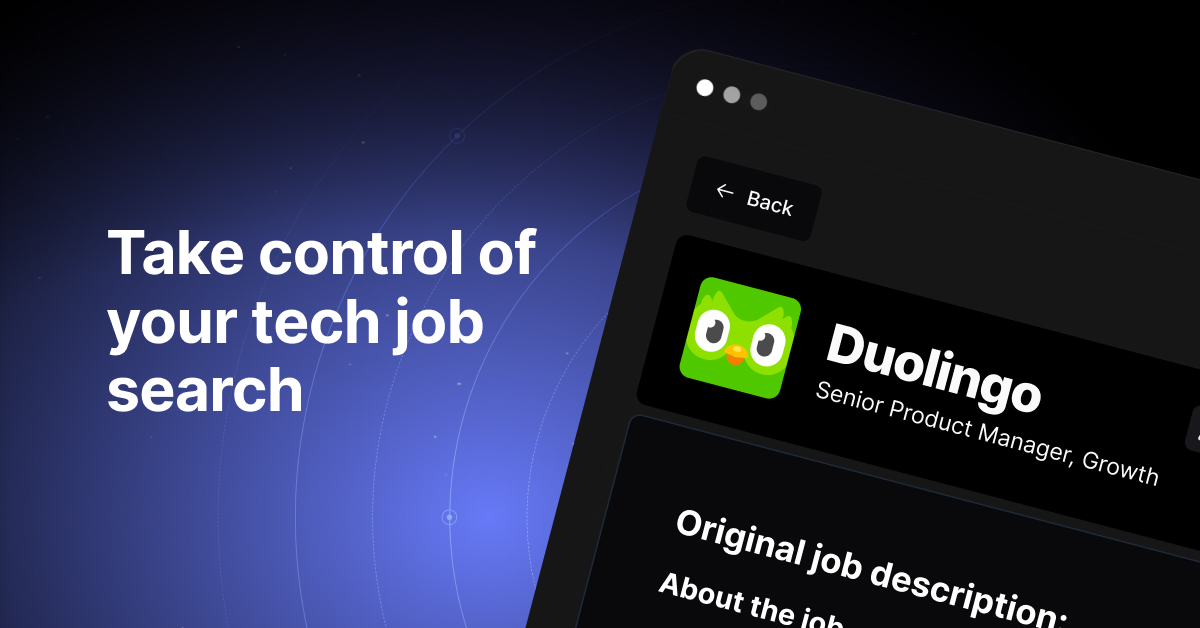