Accessing class variables from a list comprehension in the class definition
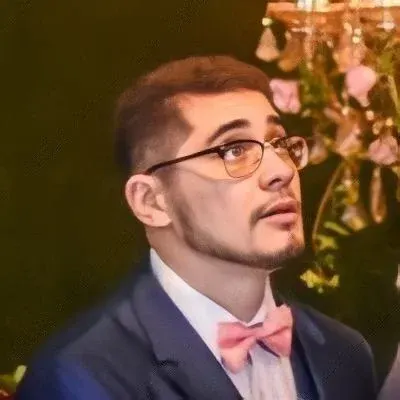
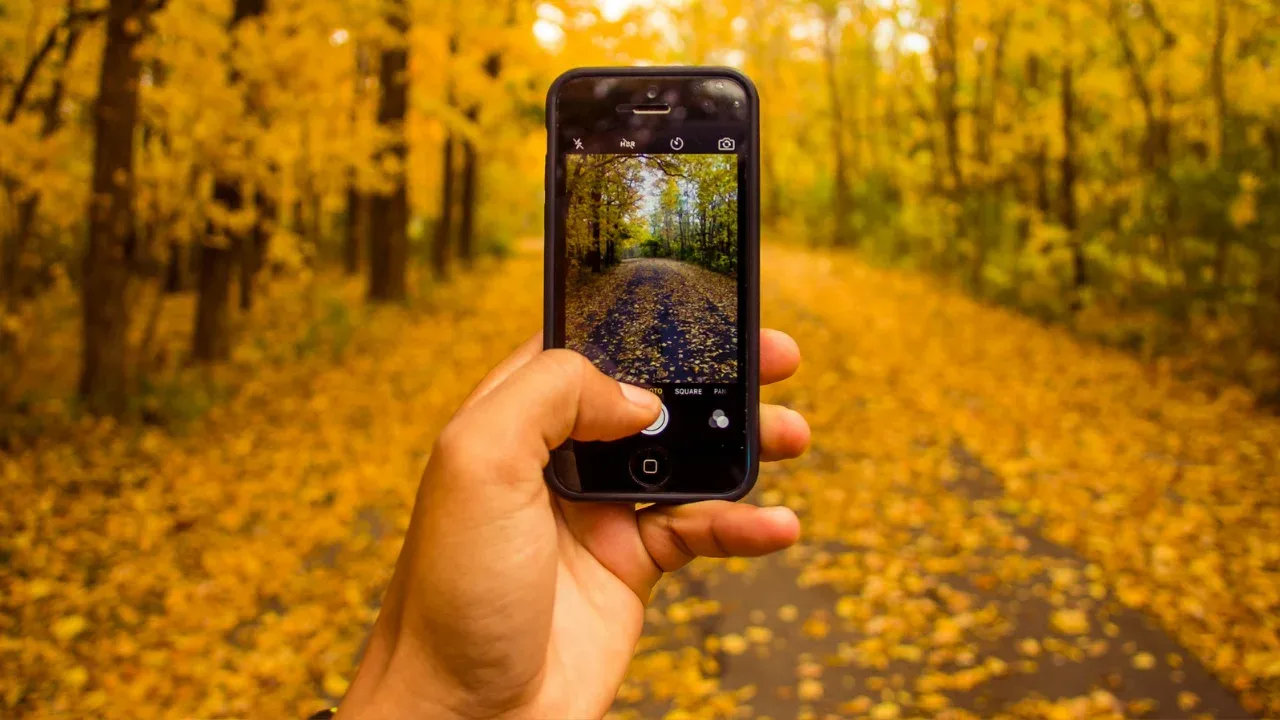
š Title: Accessing Class Variables from a List Comprehension: Python 3 Solution
š Hey there, Pythonistas! Facing trouble accessing class variables from a list comprehension within the class definition in Python 3? You're not alone! š Fear not, for we have the solution you've been looking for. Let's dive right in!
Understanding the Problem
In Python 2, accessing class variables from a list comprehension within the class definition worked seamlessly. However, Python 3 changed the scoping rules, leading to the "NameError: global name 'x' is not defined" error.
The Python 3 Solution
To overcome this hurdle, we can use the __init_subclass__
method, which was introduced in Python 3.6. This special method allows us to access the class attributes before the class initialization.
Here's an updated version of the first example using the __init_subclass__
method:
class Foo:
x = 5
def __init_subclass__(cls):
cls.y = [cls.x for _ in range(1)]
By overriding the __init_subclass__
method, we gain access to the class attributes inside the list comprehension. Voila! Problem solved! š
Solving a More Complicated Example
Now let's tackle the trickier example involving a namedtuple and a list comprehension. As the apply()
workaround isn't available in Python 3, we need a different approach to initialize the database.
from collections import namedtuple
class StateDatabase:
State = namedtuple('State', ['name', 'capital'])
def __init_subclass__(cls):
cls.db = [cls.State(*args) for args in [
['Alabama', 'Montgomery'],
['Alaska', 'Juneau'],
# ...
]]
In this solution, we make use of the __init_subclass__
method once again. Now, when you subclass StateDatabase
, the db
list will be automatically initialized with the required values.
Engage with Us!
š If you found this solution helpful, give it a try in your own code and see the magic happen!
ā Do you have any other Python 3-related questions or interesting scenarios you want us to cover? Drop them in the comments section below, and we'll be happy to help you out!
š” Stay tuned for more amazing content and Pythonic solutions. Don't forget to share this post with your Python enthusiast friends and spread the joy of code!
That's it for today, folks! Keep coding and stay Pythonic! š»šāØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
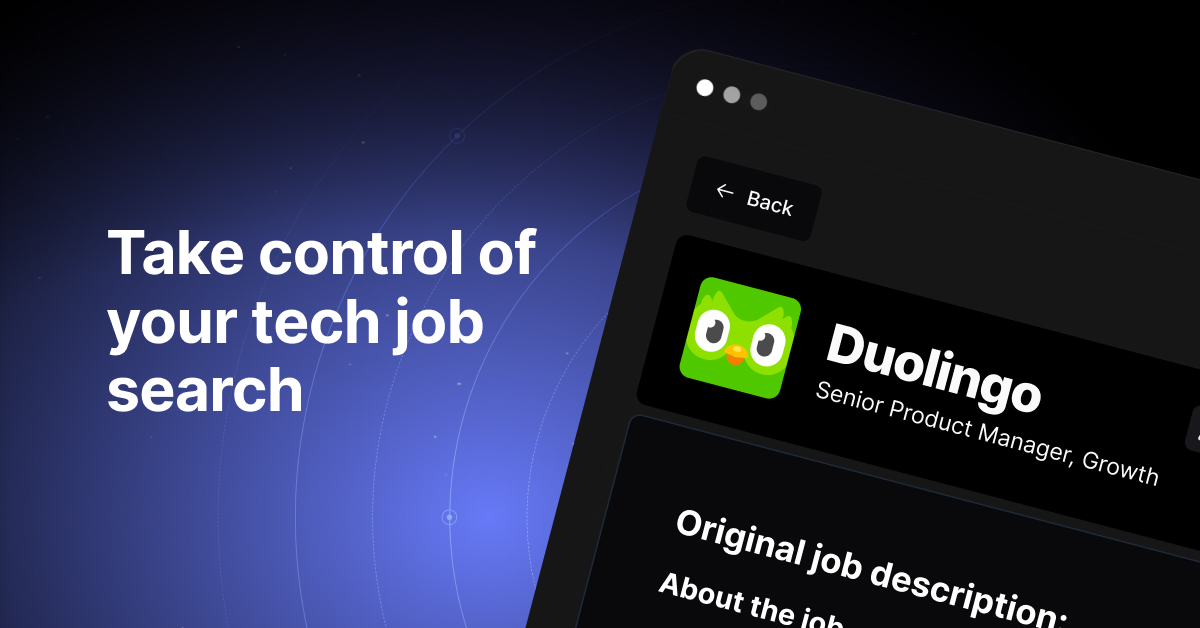