Error in Swift class: Property not initialized at super.init call
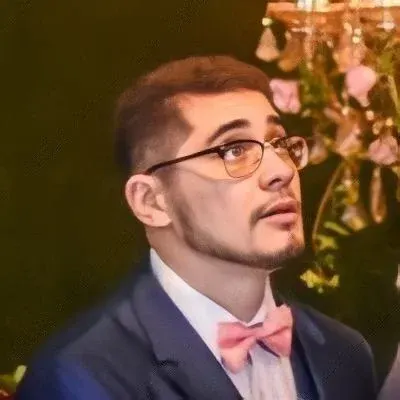
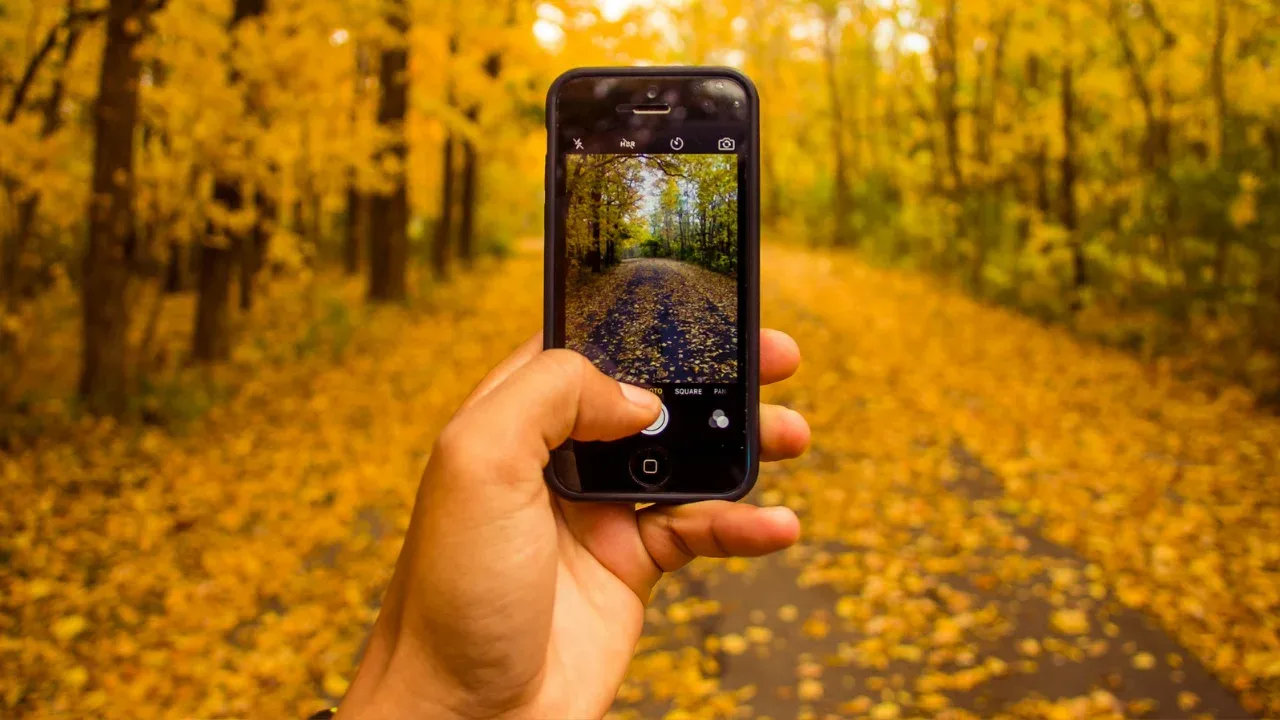
š„š Blog Post: Fixing the Error in Swift Class
š Hey there tech enthusiasts! Today, we're diving into an error that many Swift developers come across when working with classes ā the dreaded "property not initialized at super.init call" error. But fear not, we've got you covered with easy solutions to get your code up and running smoothly šāāļø
The Setup šļø
In this scenario, we have two classes ā Shape
and Square
. The Shape
class has a property called numberOfSides
and an initializer that takes in a name
parameter. On the other hand, the Square
class is a subclass of Shape
and adds a sideLength
property and an additional initializer that also takes in a name
parameter.
class Shape {
var numberOfSides = 0
var name: String
init(name: String) {
self.name = name
}
func simpleDescription() -> String {
return "A shape with \(numberOfSides) sides."
}
}
class Square: Shape {
var sideLength: Double
init(sideLength: Double, name: String) {
super.init(name: name) // Error here
self.sideLength = sideLength
numberOfSides = 4
}
func area() -> Double {
return sideLength * sideLength
}
}
The Error š„
When trying to initialize an instance of the Square
class, you encounter the following error message:
property 'self.sideLength' not initialized at super.init call
super.init(name: name)
š¤ Why do you have to set self.sideLength
before calling super.init
? Let's break it down! š”
Understanding the Error š¤
In Swift, during the initialization of a subclass, you need to ensure that all properties of the subclass are properly initialized before calling the superclass's initializer using super.init
. This requirement ensures that the subclass's properties are fully initialized and ready to be used by both the subclass and the superclass.
The Solution āļø
To resolve the error and comply with Swift's initialization rules, you need to initialize the sideLength
property of the Square
class before calling super.init
. Simply move the initialization of sideLength
above the super.init
call, like this:
init(sideLength: Double, name: String) {
self.sideLength = sideLength // Move above super.init
super.init(name: name)
numberOfSides = 4
}
By making this change, you ensure that sideLength
is properly initialized before invoking the superclass's initializer, avoiding the error altogether. š
Summing It Up š
In this blog post, we covered the common error of "property not initialized at super.init call" in Swift classes. We've learned that proper subclass initialization involves initializing all properties before calling super.init
. By following this simple rule, we can keep our code error-free and our classes functioning as expected!
Now it's time for you to put this knowledge into practice! Share your thoughts, experiences, and questions in the comments section below. Let's learn and grow together! šš
Happy coding, everyone! š»āØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
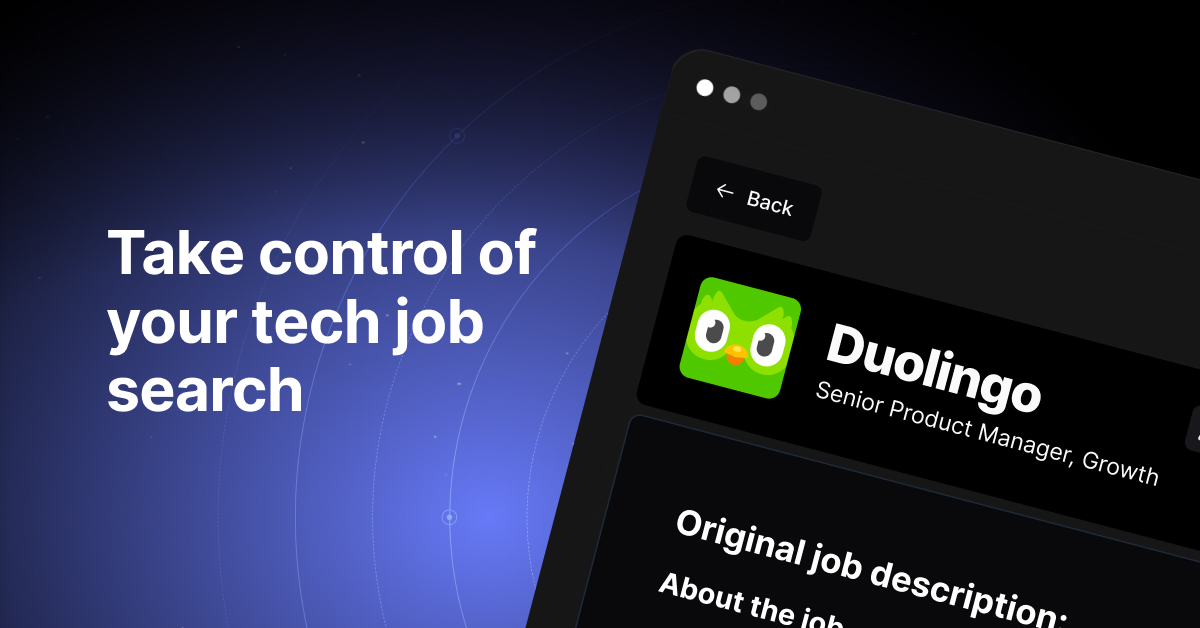