Postgres: INSERT if does not exist already
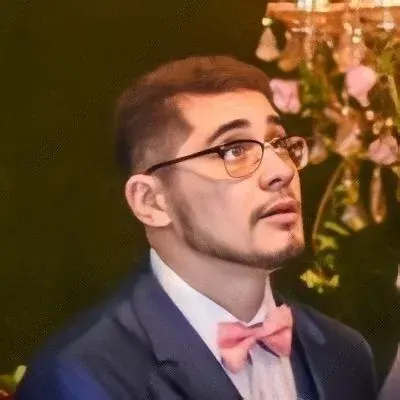
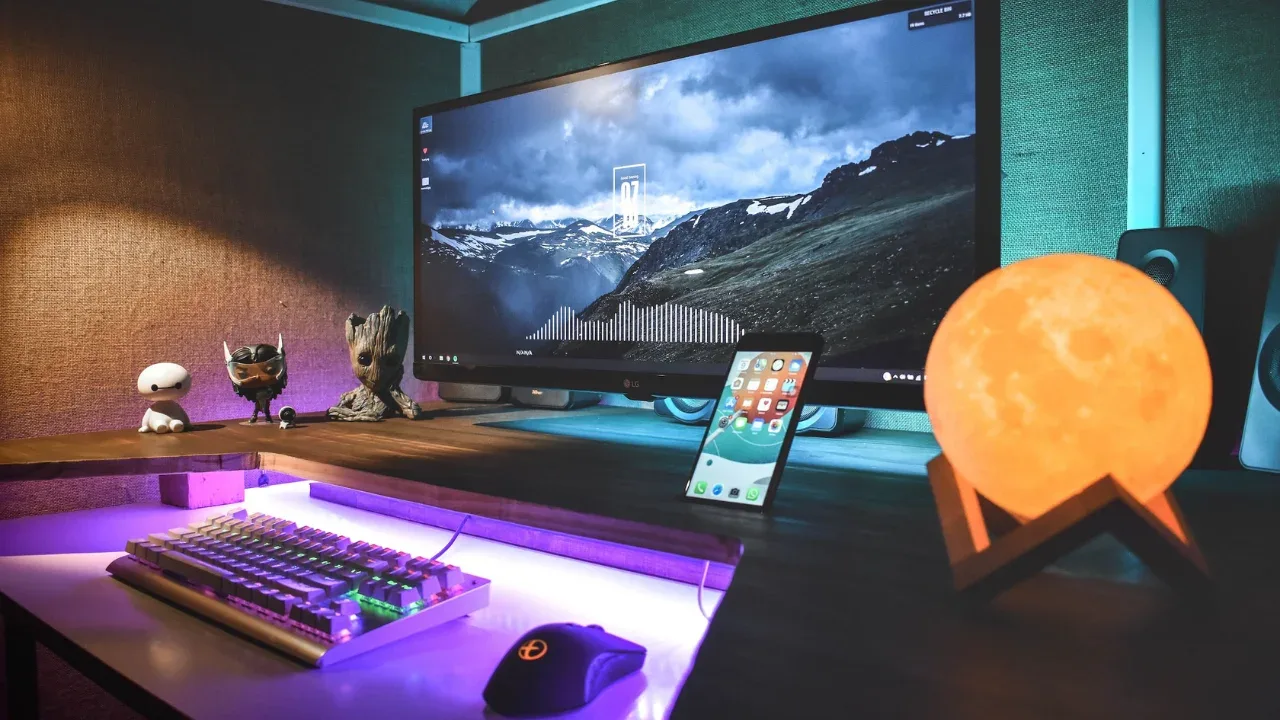
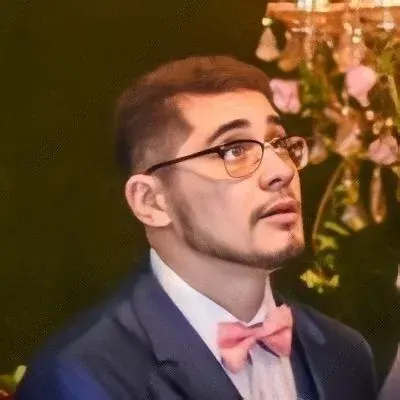
Postgres: INSERT if does not exist already
Are you struggling with duplicate key errors when trying to insert data into a Postgres database? Fear not, because we have the solution for you! In this blog post, we'll address the common issue of trying to insert a row only if it doesn't already exist, and provide you with easy and efficient solutions.
The Problem
Let's set the stage: you're using Python to write to a Postgres database, and you have a piece of code that inserts data into a table. However, when executing this code, you encounter the dreaded psycopg2.IntegrityError: duplicate key value violates unique constraint
error. 🚫😫
The Solution: UPSERT to the Rescue!
To solve this problem, we can utilize the UPSERT (INSERT ON CONFLICT DO NOTHING) feature in Postgres. UPSERT allows you to insert a row into a table, but only if it doesn't conflict with an existing row. If a conflict occurs, the statement will simply do nothing, avoiding any integrity errors. 🙌🔧
PostgreSQL UPSERT Syntax
The syntax for UPSERT is straightforward. Here's an example that you can adapt to your specific scenario:
INSERT INTO table_name (column1, column2, ...)
VALUES (value1, value2, ...)
ON CONFLICT (unique_column)
DO NOTHING;
In the above example, table_name
is the name of your table, column1
and column2
are the names of the columns you want to insert data into, and value1
and value2
are the corresponding values you want to insert. unique_column
is the column that has a unique constraint, preventing duplicate rows.
Adapting Your Code
Now, let's apply this UPSERT syntax to your specific case. Replace your existing code with the following:
sql_string = "INSERT INTO hundred (name, name_slug, status) VALUES (%s, %s, %s)"
data = (hundred, hundred_slug, status)
cursor.execute(sql_string, data)
By using parameterized queries with placeholders (%s), you not only avoid SQL injection vulnerabilities but also allow the UPSERT statement to be executed easily. 📊🔒
Easy Execution
You may be wondering how to execute this UPSERT statement as a simple string. Well, worry not! You can execute it just like any other SQL statement in Python by using the cursor.execute()
method.
Make sure you have the psycopg2 library installed, as it provides the necessary tools for connecting to your Postgres database and executing the query.
Share Your Success Story!
We hope this blog post has helped you understand how to perform an 'INSERT unless this row already exists' operation in Postgres. Now it's your turn to try it out and see the errors disappear. 🎉
If you have any questions, suggestions, or success stories using this UPSERT approach, we'd love to hear from you in the comments section below. Let's share our knowledge and help each other out! 🤝💬
Remember, the next time you encounter duplicate key errors in Postgres, just UPSERT and leave your worries behind. Happy coding! 💻💡
Note: Don't forget to backup your database before making any significant changes or performing operations that could affect your data integrity.