How to import CSV file data into a PostgreSQL table
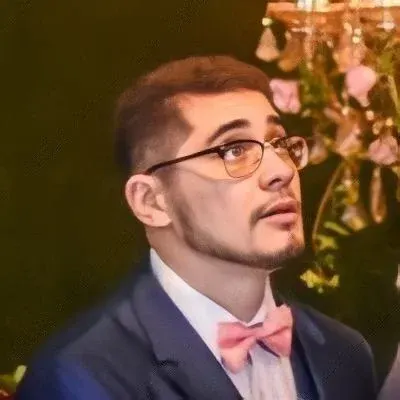
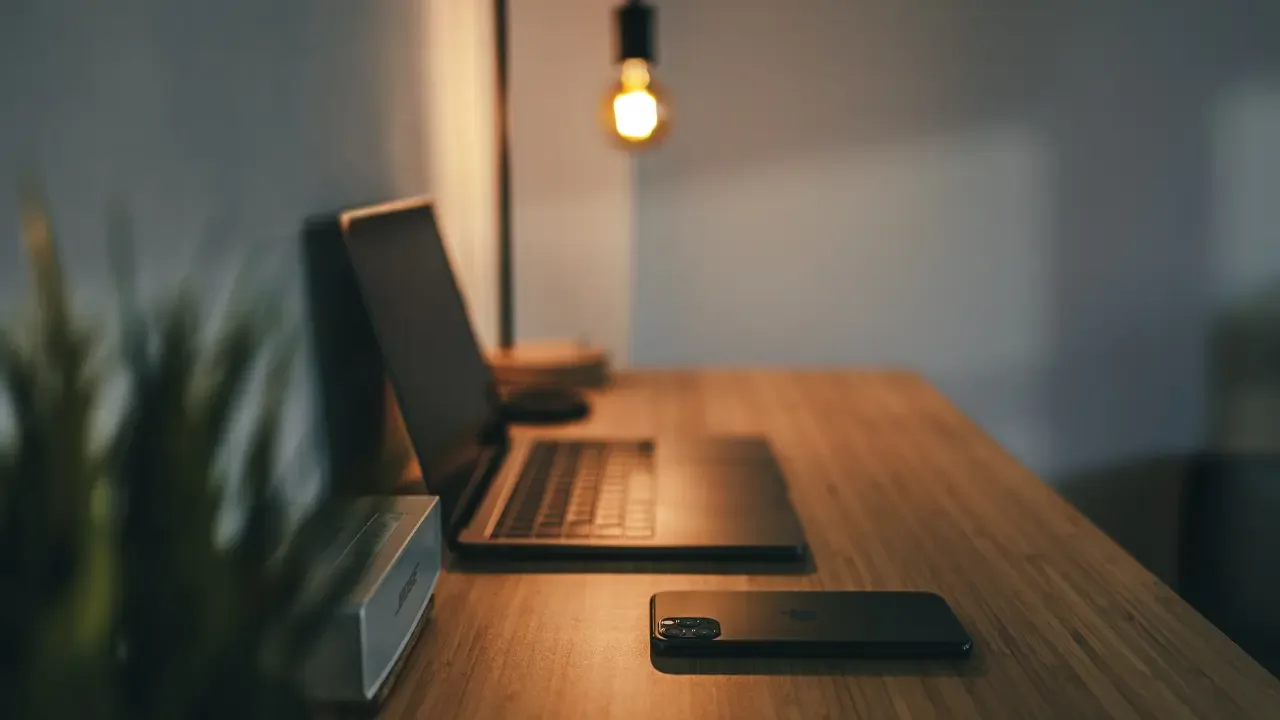
📝 Blog Post: How to 📥 Import CSV File Data into a PostgreSQL Table
Are you struggling with importing CSV file data into a PostgreSQL table? 🤔 Don't worry, you're not alone! Many developers encounter this problem while working with databases. In this blog post, we'll guide you through the steps of importing CSV file data into a PostgreSQL table, addressing common issues and providing easy solutions. So let's dive right in! 🚀
Common Issue: Writing a Stored Procedure
The question mentioned a desire to write a stored procedure to facilitate the data import process. Writing a stored procedure can indeed be a useful approach, especially if you need to perform repetitive tasks or encapsulate complex logic. Let's take a look at how we can achieve this. 💡
Assuming you already have a PostgreSQL table set up and your CSV file ready, follow these steps:
Create a new stored procedure using the
CREATE OR REPLACE FUNCTION
statement. Here's an example:CREATE OR REPLACE FUNCTION import_data_from_csv() RETURNS void AS $$ DECLARE csv_file text := 'path/to/your/csv/file.csv'; BEGIN -- Your logic to import data from the CSV file END; $$ LANGUAGE plpgsql;
In the above code, we define a new function named
import_data_from_csv()
that returnsvoid
. Modify thecsv_file
variable to the actual path of your CSV file.Implement the logic in the function to import data from the CSV file. PostgreSQL provides the
COPY
command, which allows us to load data from a CSV file into a table. Here's an example:COPY your_table_name (column1, column2, column3) FROM csv_file WITH (FORMAT csv, HEADER true, DELIMITER ',');
Replace
your_table_name
with the actual name of your table and specify the columns to import.Save the changes and execute the stored procedure using the following command:
SELECT import_data_from_csv();
This will trigger the execution of the stored procedure, importing the data from the CSV file into the specified table. 🎉
Easy Solution: Direct Copy Command
If you prefer a simpler solution to import CSV file data into a PostgreSQL table without using stored procedures, you can use the COPY
command directly. Here's how:
Open the PostgreSQL command-line interface, such as
psql
or any GUI tool that allows executing SQL statements.Run the following command to import data from the CSV file into the table:
COPY your_table_name (column1, column2, column3) FROM 'path/to/your/csv/file.csv' WITH (FORMAT csv, HEADER true, DELIMITER ',');
Replace
your_table_name
with the actual name of your table and specify the columns to import.Execute the command, and PostgreSQL will load the data from the CSV file into your table seamlessly. 🎉
Call-to-Action: Share Your Experience!
We hope this article helped you resolve the challenge of importing CSV file data into a PostgreSQL table. Now it's time for you to put it into practice! Try out the methods mentioned above and let us know your experience in the comments below. Did it work smoothly, or did you encounter any issues? We're eager to hear your success stories and help you with any difficulties you may face. Don't forget to share this blog post with your coder friends who might also find it helpful! 😄
Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
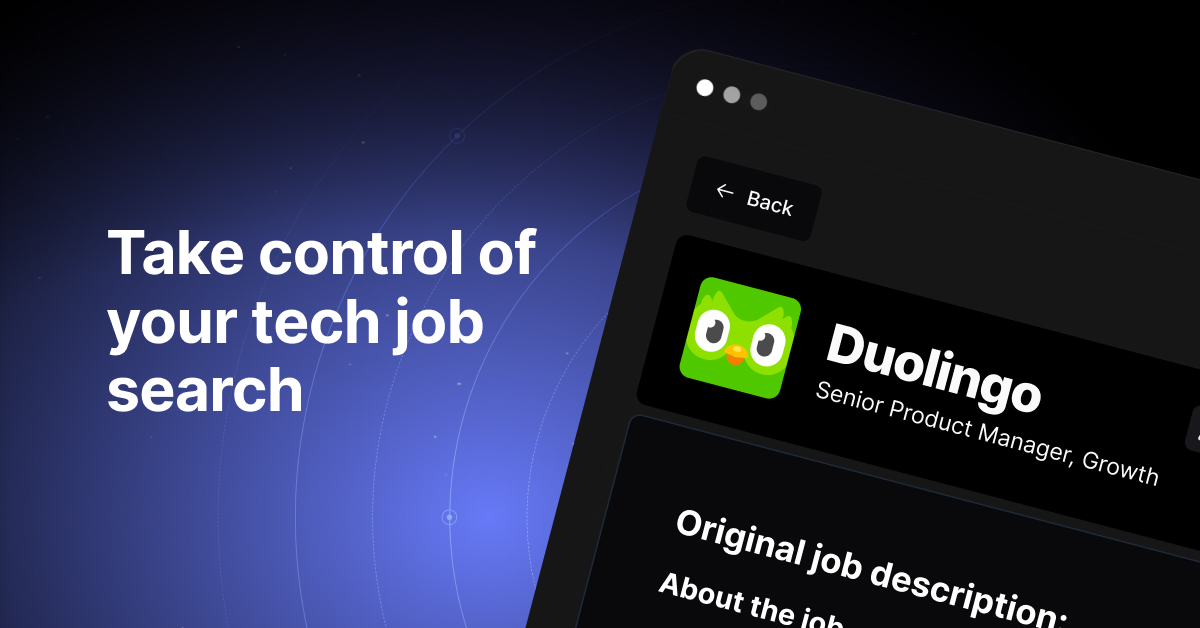