How do you find the row count for all your tables in Postgres
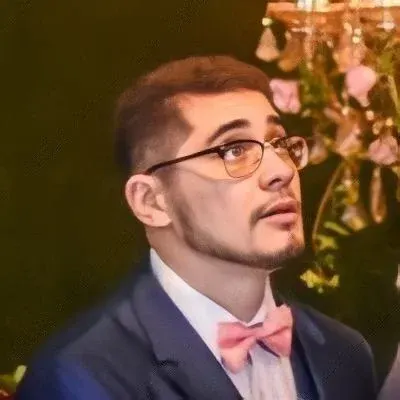
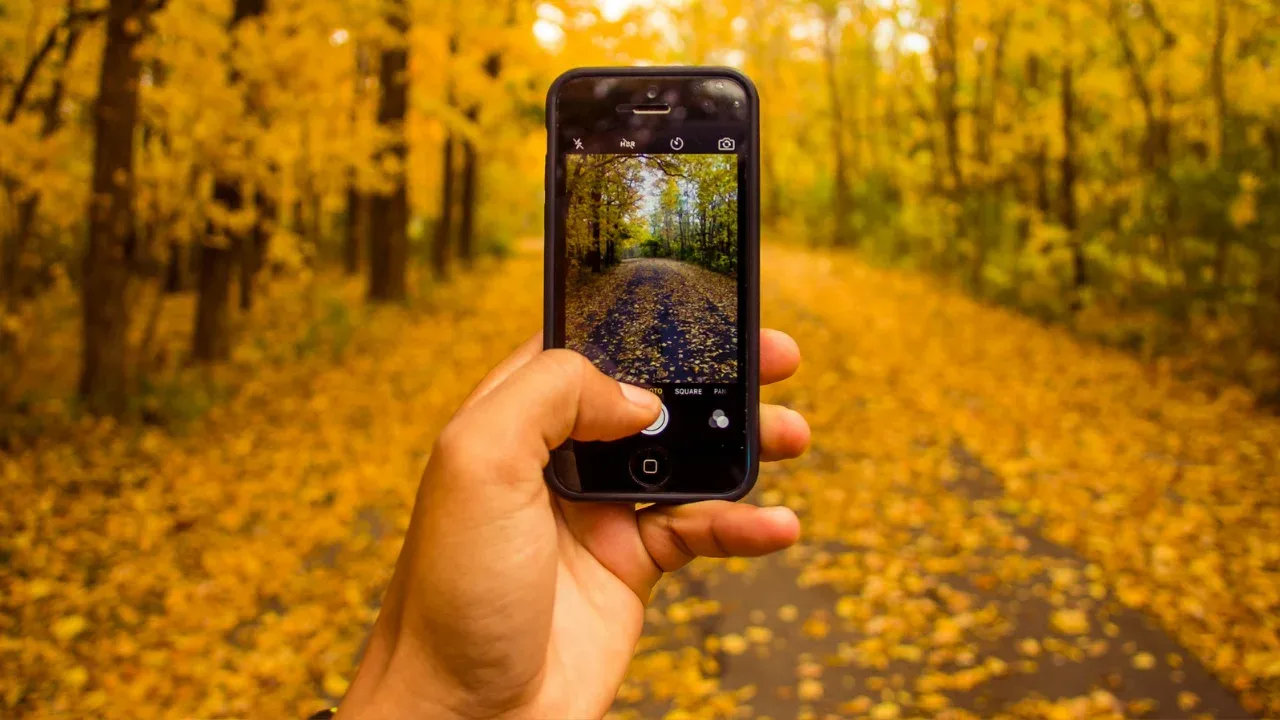
How to Get the Row Count for All Your Tables in Postgres 📊📈
Are you curious about the size of the tables in your Postgres database? Maybe you want to know which tables have the most rows or simply get an idea of how big your database is overall. Whatever your reasons are, we've got you covered! In this guide, we'll show you an easy and efficient way to obtain the row count for all your tables in Postgres. So let's dive right in! 💪
The Problem: Counting Rows One Table at a Time ⏰
You might already be familiar with finding the row count for a single table in Postgres using the following SQL query:
SELECT count(*) FROM table_name;
This approach works perfectly fine when you want to assess the size of one specific table. However, it becomes cumbersome and time-consuming if you have multiple tables and need to count rows for each one individually.
The Solution: Querying the Information Schema 📝🔍
To obtain the row count for all your tables in one go and even order them by their size, we can leverage the information_schema
in Postgres. The information_schema
is a system catalog that provides essential metadata about your database objects.
Here's an example of a query that retrieves the row count for all tables and sorts them in descending order:
SELECT table_name,
(SELECT reltuples::bigint AS row_count
FROM pg_class
WHERE relname = table_name)
AS row_count
FROM information_schema.tables
WHERE table_type = 'BASE TABLE'
AND table_schema NOT LIKE 'pg_%'
ORDER BY row_count DESC;
Let's break down what this query does:
We start by selecting the
table_name
from theinformation_schema.tables
view.Next, we use a subquery to fetch the row count from the
pg_class
table using therelname
(table name) andreltuples
(approximate number of rows) columns.We alias the row count column as
row_count
for clarity.The
FROM
clause restricts the query to only include base tables (excluding views) and filters out system tables (those starting with "pg_").Finally, we order the results by the row count in descending order using the
ORDER BY
clause.
That's it! 🎉 Running this query will provide you with the row count for all your tables, allowing you to gauge their sizes effortlessly.
Take It to the Next Level: Automating the Process with a Function 🔄🤖
If you find yourself frequently needing to retrieve the row count for all your tables, you can take it a step further and create a function. This function will encapsulate the query for you, making it even easier to fetch the information you need in a snap!
Here's an example of how you could define a function called get_table_row_counts
that returns the table name and row count:
CREATE OR REPLACE FUNCTION get_table_row_counts()
RETURNS TABLE (table_name text, row_count bigint) AS
$func$
BEGIN
RETURN QUERY
SELECT table_name,
(SELECT reltuples::bigint AS row_count
FROM pg_class
WHERE relname = t.table_name)
FROM information_schema.tables t
WHERE table_type = 'BASE TABLE'
AND table_schema NOT LIKE 'pg_%'
ORDER BY row_count DESC;
END
$func$ LANGUAGE plpgsql;
With this function in place, you can now simply call it whenever you need to get the row count for all your tables:
SELECT * FROM get_table_row_counts();
Engage with Us! 🙌🗣
Did this blog post help you effortlessly find the row count for all your tables in Postgres? We hope it did! If you have any additional questions or want to share your thoughts, feel free to leave a comment below. We'd love to hear from you! 😊✨
And don't forget to hit the share button and spread the knowledge with your fellow developers! 🚀🌐
Happy querying! 🎉📊
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
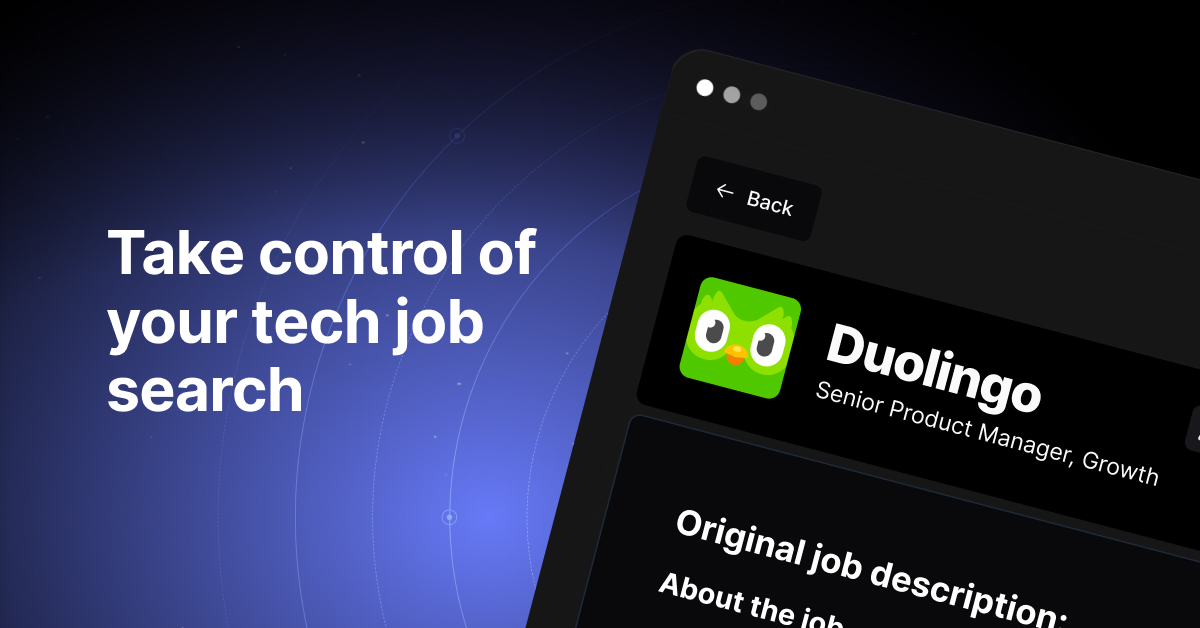