HTTP Request in Swift with POST method
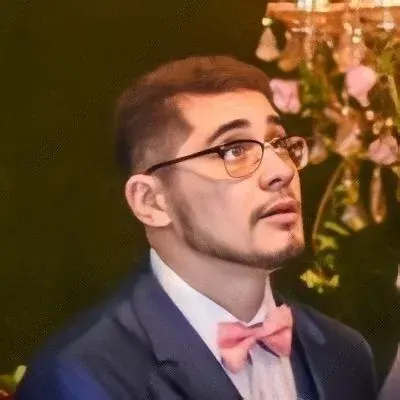
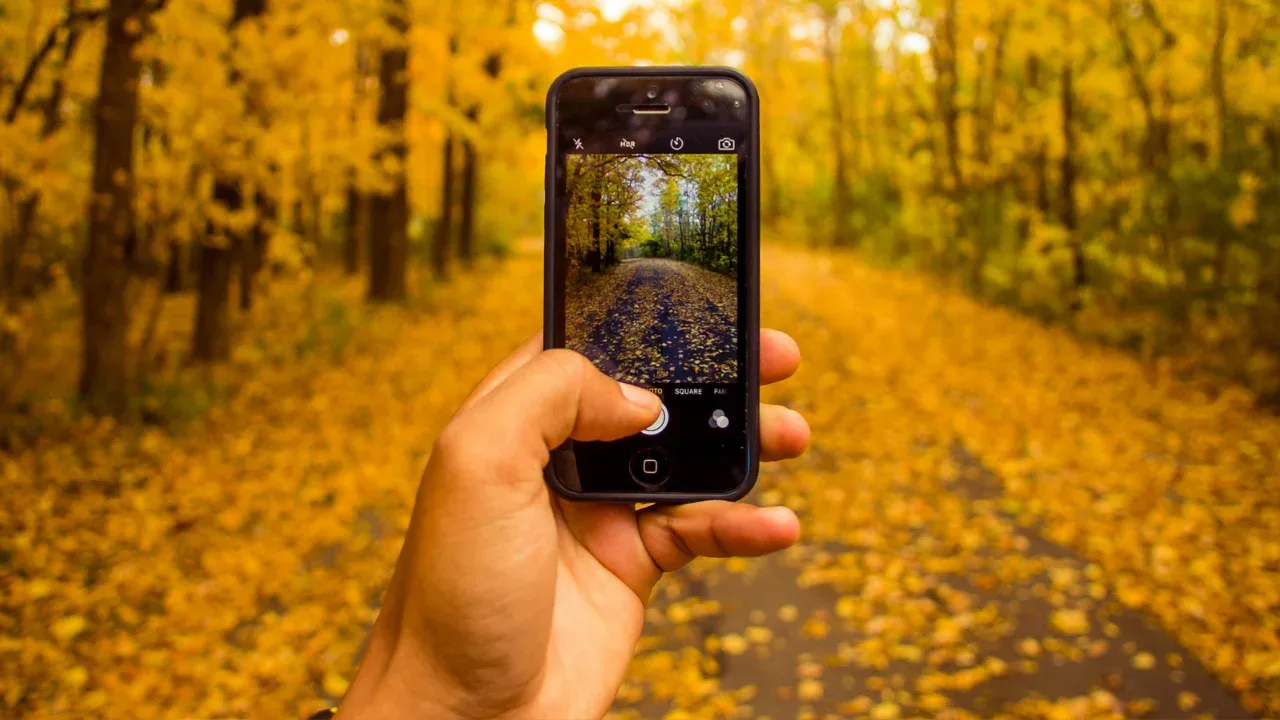
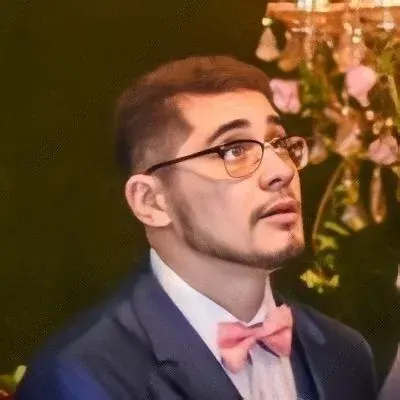
📝 The Easiest Way to Send HTTP Request in Swift with POST Method
Are you struggling to send an HTTP request with the POST method in Swift? Do you want to send parameters to a URL without bothering about reading the response? We got you covered! In this blog post, we'll guide you through the simplest way to achieve that. So let's dive right in! 💪
Understanding the Problem
You mentioned that you want to POST two parameters, id
and name
, to a specific URL. Here's an example of how your request should look:
Link: www.thisismylink.com/postName.php
Params:
id = 13
name = Jack
Now, let's break down the problem and find a solution that makes your life easier.
The Solution
To send an HTTP request with the POST method in Swift, we can leverage the power of URLSession
. Here's a step-by-step guide on how to achieve this:
Create a URL object with your desired URL, including the PHP file endpoint:
guard let url = URL(string: "http://www.thisismylink.com/postName.php") else {
print("Invalid URL")
return
}
Create a URLRequest object and set its
httpMethod
property to "POST":
var request = URLRequest(url: url)
request.httpMethod = "POST"
Define your parameters as a dictionary:
let parameters = [
"id": "13",
"name": "Jack"
]
Convert the parameter dictionary to a Data object:
guard let postData = try? JSONSerialization.data(withJSONObject: parameters, options: []) else {
print("Failed to encode parameters")
return
}
Set the request's
httpBody
property with the postData:
request.httpBody = postData
Create a URLSession and a data task:
let session = URLSession.shared
let task = session.dataTask(with: request) { (data, response, error) in
if let error = error {
print("Error: \(error)")
return
}
// Optionally handle the response here
}
Finally, resume the data task to send the HTTP request:
task.resume()
And voila! 🎉 You have successfully sent an HTTP POST request with your parameters!
Call-to-Action
Now that you have a simple solution to send HTTP requests in Swift, we encourage you to try it out in your own code. Experiment with different endpoints, URL formats, and parameters to get the hang of it.
If you have any questions or run into any issues, feel free to leave a comment below. We'll be more than happy to assist you! 😊
Happy coding! 💻🚀