Woocommerce get products
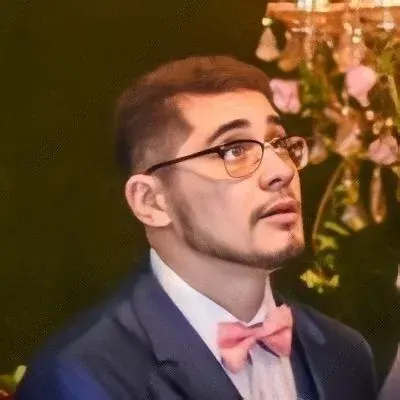
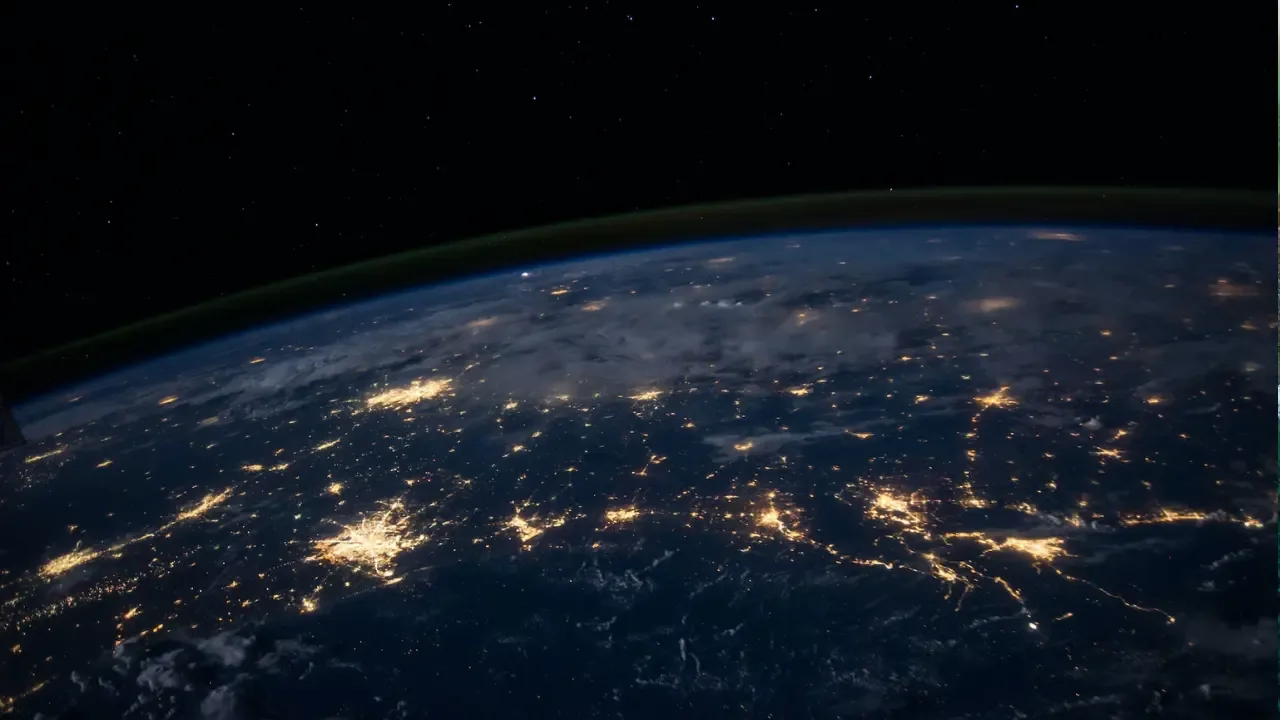
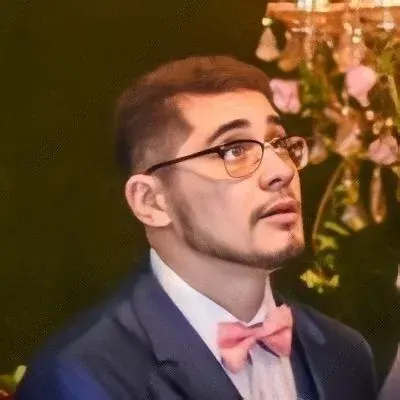
📢🔔📢 Hey tech enthusiasts! 👋👋 Welcome back to another exciting blog post where we dive into the wonderful world of WooCommerce! 🛒💻 Today, we tackle a common question that many developers face when working with WooCommerce: "How do I get the list of products for a particular category?" 🤔
🧐💭 Let's start by looking at the code snippet that was provided:
<?php
$taxonomy = 'product_cat';
$orderby = 'name';
$show_count = 0; // 1 for yes, 0 for no
$pad_counts = 0; // 1 for yes, 0 for no
$hierarchical = 0; // 1 for yes, 0 for no
$title = '';
$empty = 0;
$args = array(
'taxonomy' => $taxonomy,
'orderby' => $orderby,
'show_count' => $show_count,
'pad_counts' => $pad_counts,
'hierarchical' => $hierarchical,
'title_li' => $title,
'hide_empty' => $empty
);
?>
<?php $all_categories = get_categories( $args );
foreach ($all_categories as $cat) {
if($cat->category_parent == 0) {
$category_id = $cat->term_id;
?>
<?php
echo '<br /><a href="'. get_term_link($cat->slug, 'product_cat') .'">'. $cat->name .'</a>'; ?>
<?php
$args2 = array(
'taxonomy' => $taxonomy,
'child_of' => 0,
'parent' => $category_id,
'orderby' => $orderby,
'show_count' => $show_count,
'pad_counts' => $pad_counts,
'hierarchical' => $hierarchical,
'title_li' => $title,
'hide_empty' => $empty
);
$sub_cats = get_categories( $args2 );
if($sub_cats) {
foreach($sub_cats as $sub_category) {
echo $sub_category->name ;
}
}
}
}
?>
👀📝 As you can see, this code snippet successfully retrieves the list of product categories in WooCommerce. However, the challenge arises when trying to get a list of products for a specific category. 😓
🎯💡 To achieve this, we need to leverage the power of WooCommerce's built-in functions. Let's take a look at how we can modify the existing code to get the desired result. 🚀
👉🧰 Here's an example of how you can retrieve all the products for a specific category, using cat_id=34
as an example:
$category_id = 34; // Replace with the desired category ID
$args = array(
'post_type' => 'product',
'posts_per_page' => -1,
'tax_query' => array(
array(
'taxonomy' => 'product_cat',
'field' => 'term_id',
'terms' => $category_id,
),
),
);
$products = new WP_Query( $args );
if ( $products->have_posts() ) {
while ( $products->have_posts() ) {
$products->the_post();
echo '<h3>' . get_the_title() . '</h3>';
echo '<p>' . get_the_content() . '</p>';
}
wp_reset_postdata();
} else {
echo 'No products found in the specified category.';
}
✨🚀 And just like that, we have the solution to our problem! By utilizing the WP_Query
class and the tax_query
parameter, we can easily retrieve all the products assigned to a specific category. 🙌🔍
🔊📣 Now, it's your turn to try it out! Replace the category_id
variable with the desired category ID and watch the magic happen! You'll be amazed at how simple and efficient it is to get the list of products for a particular category in WooCommerce. 💪💻
🚀🌟 If you found this blog post helpful, don't forget to share it with your fellow WooCommerce enthusiasts and spread the knowledge! And, as always, feel free to leave your questions or comments down below – we'd love to hear from you! 🗣️📩
💡🙌 Stay tuned for more exciting tech tips and tricks from our blog. Until next time, happy coding! ✨🎉