What is the difference between find(), findOrFail(), first(), firstOrFail(), get(), list(), toArray()
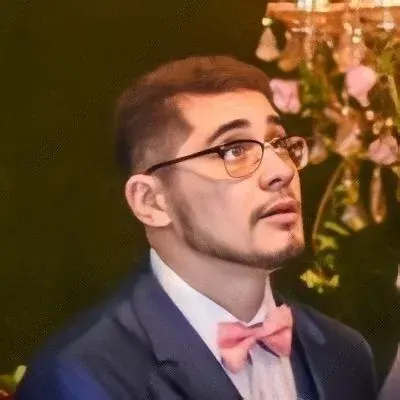
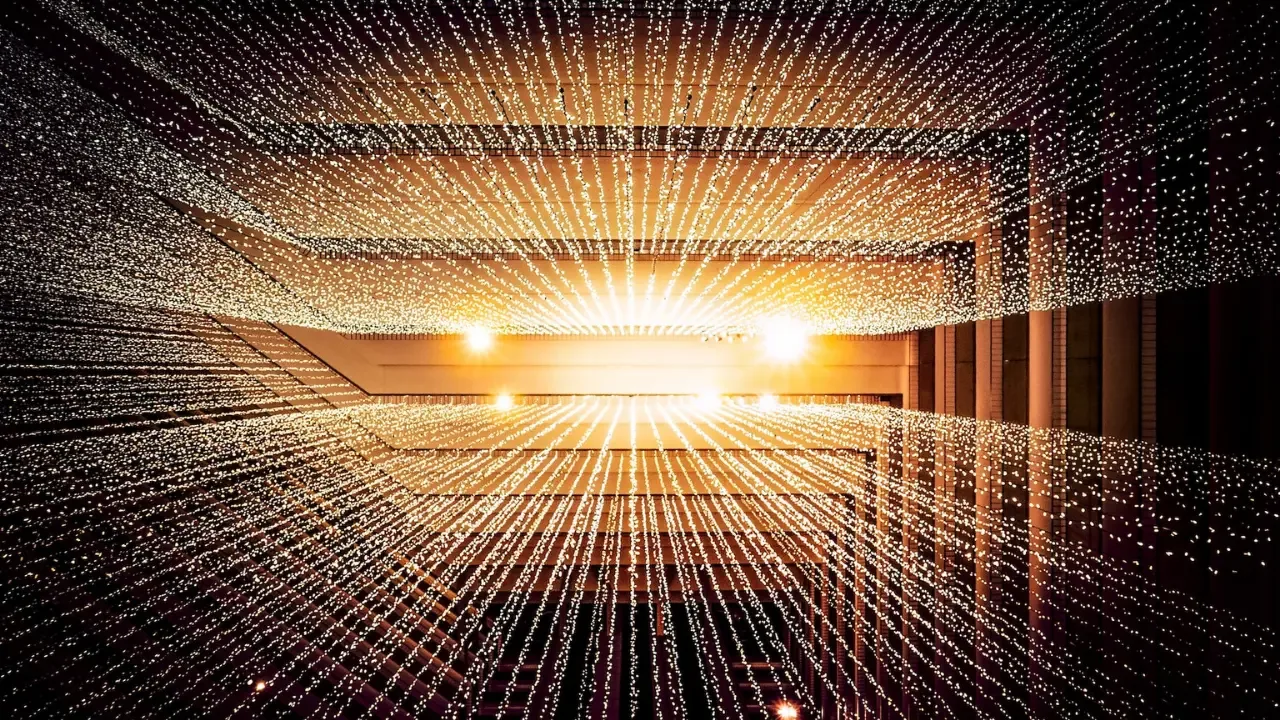
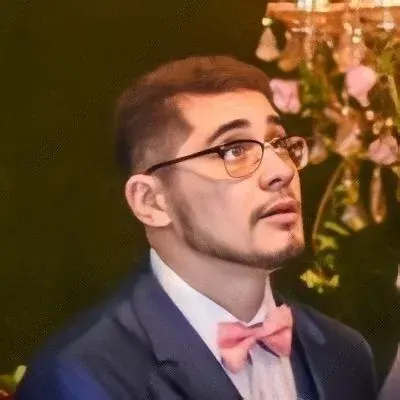
Understanding the Differences: find(), findOrFail(), first(), firstOrFail(), get(), list(), toArray() ๐ค๐๐
Are you confused about the differences between the Laravel Eloquent methods find()
, findOrFail()
, first()
, firstOrFail()
, get()
, list()
, and toArray()
? ๐คทโโ๏ธ Don't worry, you're not alone! Many developers stumble upon this issue and find it challenging to understand when and how to use each of these methods.
In this blog post, we'll explain the purpose of each method, clarify the differences between them, and provide easy solutions to common problems. So, let's dive in and demystify these Eloquent methods! ๐ป๐กโจ
1. find()
๐
The find()
method is used to retrieve a single record from the database based on its primary key. It accepts the primary key value as an argument and returns the matching record as an Eloquent model or null
if no record is found.
$user = User::find(1);
2. findOrFail()
โ๏ธ๐
Similarly to find()
, the findOrFail()
method retrieves a single record based on the primary key. However, if no record is found, it throws a ModelNotFoundException
exception. This can be particularly useful when you expect the record to exist and want to handle the case of not finding it gracefully.
try {
$user = User::findOrFail(1);
} catch (ModelNotFoundException $e) {
// Handle the not found case here
}
3. first()
โ๏ธ
The first()
method returns the first record retrieved from the database based on the query constraints. It is commonly used when you want to retrieve the first result of a query and don't need to specify a primary key.
$user = User::where('is_admin', true)->first();
4. firstOrFail()
โ๏ธโ๏ธ
Just like first()
, the firstOrFail()
method returns the first record that matches the query constraints. However, if no record is found, it throws a ModelNotFoundException
exception.
try {
$user = User::where('is_admin', true)->firstOrFail();
} catch (ModelNotFoundException $e) {
// Handle the not found case here
}
5. get()
โ๏ธ
The get()
method retrieves all records that match the query constraints. It returns a collection of Eloquent models, allowing you to iterate over the results and perform various operations on them.
$users = User::where('is_admin', true)->get();
6. list()
๐
The list()
method, deprecated since Laravel 5.2, was used to build an array containing specific columns from the retrieved records. However, this method can now be replaced with the pluck()
method, which provides a more concise and flexible way of achieving the same result.
$names = User::where('is_admin', true)->pluck('name');
7. toArray()
๐
Finally, the toArray()
method can be used to convert Eloquent models or collections into a plain PHP array. This is particularly handy when you need to return data in an array format, like when serializing data for API responses.
$userArray = $user->toArray();
$usersArray = User::where('is_admin', true)->get()->toArray();
Conclusion and Next Steps ๐
Now that you have a clearer understanding of the differences between find()
, findOrFail()
, first()
, firstOrFail()
, get()
, list()
, and toArray()
, it's time to put your knowledge into action! ๐ฉโ๐ป๐จโ๐ป
Remember to consider your specific use case and choose the appropriate method accordingly. If you're still unsure, consult the Laravel documentation or ask for help on online communities like Stack Overflow.
So go ahead and confidently fetch and manipulate your data using the right Eloquent methods! If you found this blog post helpful, leave a comment and share it with your fellow developers. We'd love to hear about your experiences and any additional tips or tricks you have. Happy coding! ๐๐ป๐ฌ