What is a Python equivalent of PHP"s var_dump()?
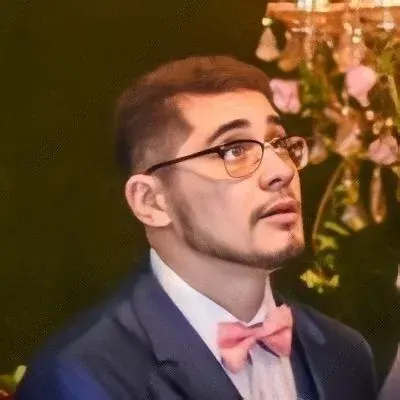
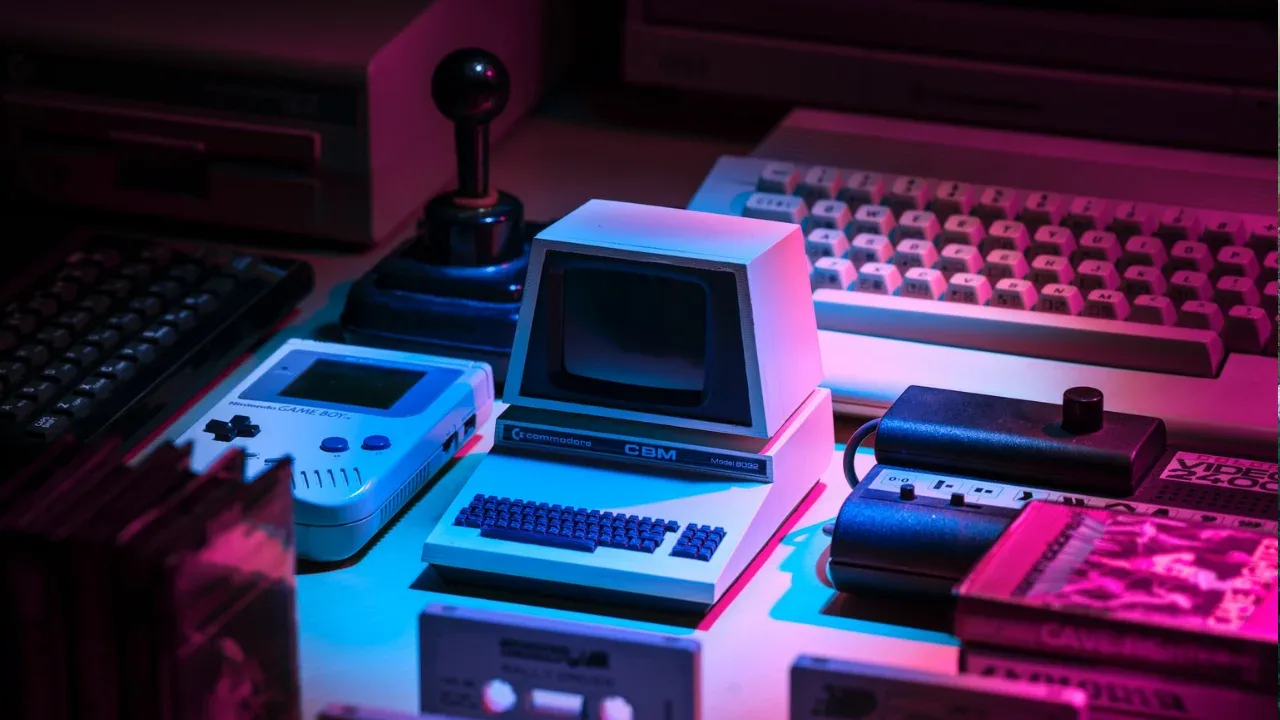
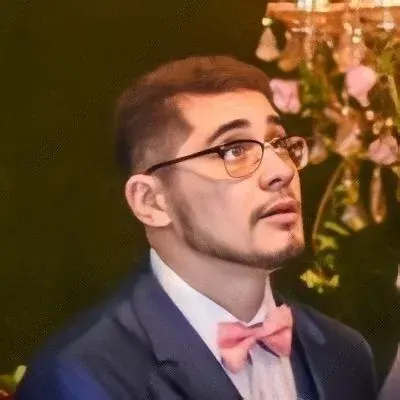
What is a Python equivalent of PHP's var_dump()?
šš
When it comes to debugging in PHP, the handy var_dump() function comes to the rescue. It allows you to quickly inspect the contents and values of variables. But what about Python? Is there an equivalent that can make debugging a breeze? š¤
Fortunately, Python provides a powerful alternative for this called the pprint module. š
Introducing pprint: The Pythonic Debugger š
The pprint module in Python stands for "pretty printer." Its primary purpose is to format complex data structures in a readable way. In other words, pprint makes your data pretty and organized when it comes to debugging. š
Compared to the straightforward var_dump() in PHP, pprint provides more structured and visually appealing output. It's especially useful when dealing with nested lists, dictionaries, or objects.
How to Use pprint in Python š„ļø
To utilize pprint in your Python code, you'll need to import it first using the following line of code:
import pprint
Once imported, you can invoke the pprint() function and pass in your variable as an argument. Let's take a look at an example:
import pprint
my_variable = [1, 2, [3, 4, [5, 6], 7], 8]
pprint.pprint(my_variable)
Running the code above will yield the following output:
[1, 2, [3, 4, [5, 6], 7], 8]
As you can see, pprint arranges the data structure in an organized manner, making it easy to visualize the variable's contents. It's quite similar to the tabular output provided by var_dump().
š Pro Tip: Using pprint with JSON š
One cool aspect of pprint is its compatibility with JSON. If you're working with JSON data and want to dive deep into its structure, pprint can be your best friend. By combining the json module and pprint, you can easily prettify JSON output. š
Here's an example:
import json
import pprint
my_json = '{"name": "John", "age": 30, "city": "New York"}'
parsed_json = json.loads(my_json)
pprint.pprint(parsed_json)
Running this code will produce the following output:
{'name': 'John', 'age': 30, 'city': 'New York'}
Embrace the Power of pprint š¤©
The pprint module in Python might not have the exact same name as var_dump() in PHP, but it serves the same purpose: helping you effectively debug and understand your code.
By using pprint, you can visualize complex data structures more clearly, making it easier to identify and resolve issues. Whether you're working on a small script or a large project, pprint is a fantastic tool to have in your debugging toolbox. š§°
So why not give pprint a try today? Upgrade your debugging game and let pprint make your Python code look pretty! Don't be afraid to dive into those deep nested data structures and explore every bit of your variables. šµļøāāļø
Feel free to leave a comment below and share your debugging experiences or any other Python tips and tricks you find helpful! Let's debug together! šāØ