using jquery $.ajax to call a PHP function
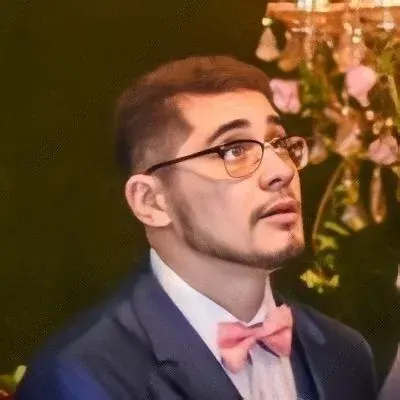
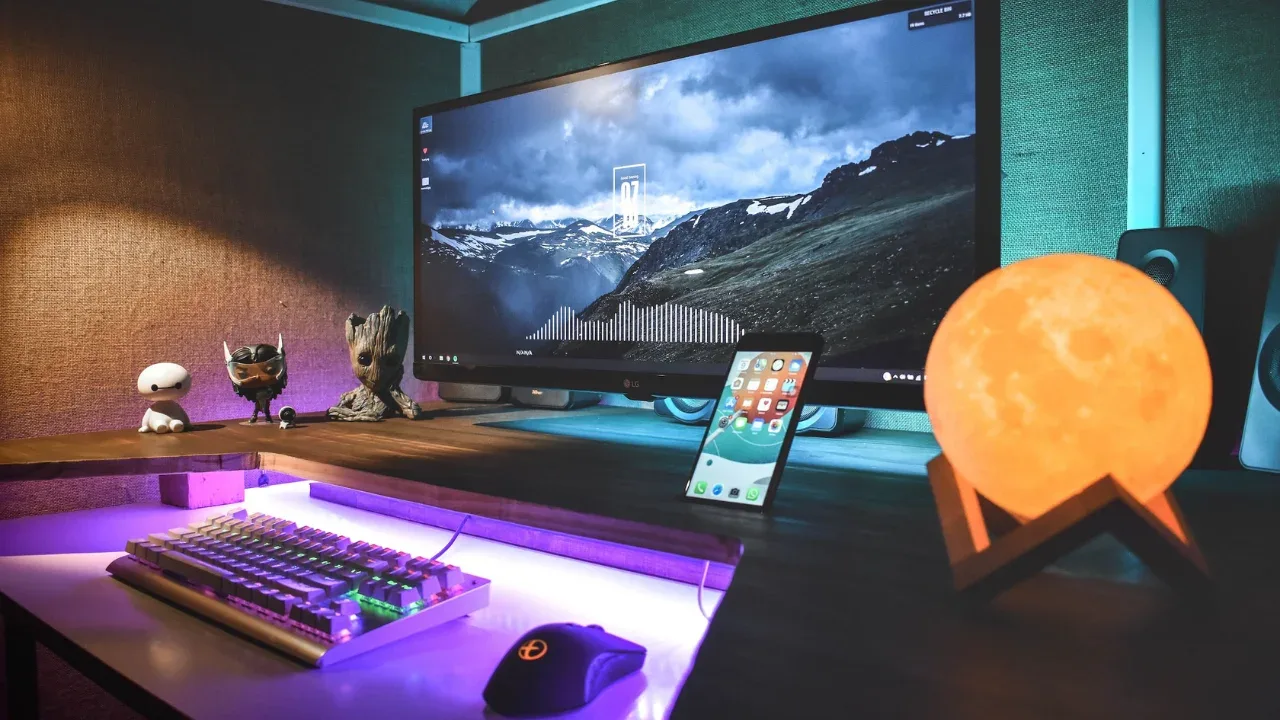
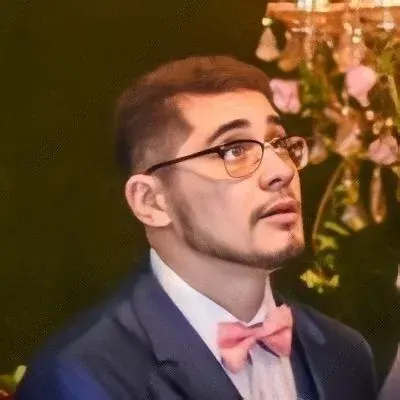
š Blog Post: Using jQuery $.ajax to Call a PHP Function
š” Have you ever wondered how to call a PHP function from JavaScript using jQuery's powerful $.ajax method? Look no further! In this post, we will address this common issue and provide you with easy solutions and practical examples to achieve the desired functionality.
š¤ The Problem: Calling a PHP Function from JavaScript
Let's start by understanding the problem at hand. Imagine you have a PHP script that performs a specific task, and you want to encapsulate that script within a function. You then want to call this PHP function from a JavaScript function using the $.ajax method.
Here's a simplified example of the code structure:
PHP Script:
<?php
if(isset($_POST['something'])) {
// Do something
}
?>
Refactored PHP Script within a Function:
<?php
function test() {
if(isset($_POST['something'])) {
// Do something
}
}
?>
The question arises: How can we call the test()
function in JavaScript using the $.ajax method?
š The Solution: Using $.ajax to Invoke the PHP Function
To call a PHP function using $.ajax, you need to make a POST request to the PHP file containing the function and provide the necessary parameters. Let's break it down into simple steps:
Step 1: Create the JavaScript function that calls the PHP function.
function callPHPFunction() {
$.ajax({
url: 'path/to/your/php/file.php',
method: 'POST',
data: { // Optional data to send to the PHP file
something: 'value',
},
success: function(response) {
// Handle the response from the PHP function
console.log(response);
},
error: function(xhr, status, error) {
// Handle any errors during the request
console.error(error);
}
});
}
Step 2: Ensure you have the correct path to your PHP file in the url
property.
Step 3: Within the PHP file, call the desired function.
<?php
include 'path/to/your/php/file.php';
test(); // Calling the function
// Additional logic or output here if needed
?>
š You're Ready to Go!
By following these steps, you can successfully call a PHP function from JavaScript using $.ajax. Feel free to modify the code snippets according to your specific requirements.
š Join the Conversation
We hope this guide has helped you overcome the challenge of using $.ajax to call a PHP function. If you have any further questions or suggestions, let us know in the comments below. Share your own experiences and code examples to enrich the discussion.
Remember, sharing is caring. Don't forget to share this blog post with your fellow developers who might also find it helpful.
Happy coding! š©āš»šØāš»