Trying to get Laravel 5 email to work
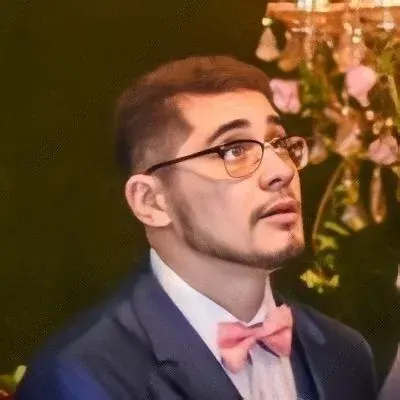
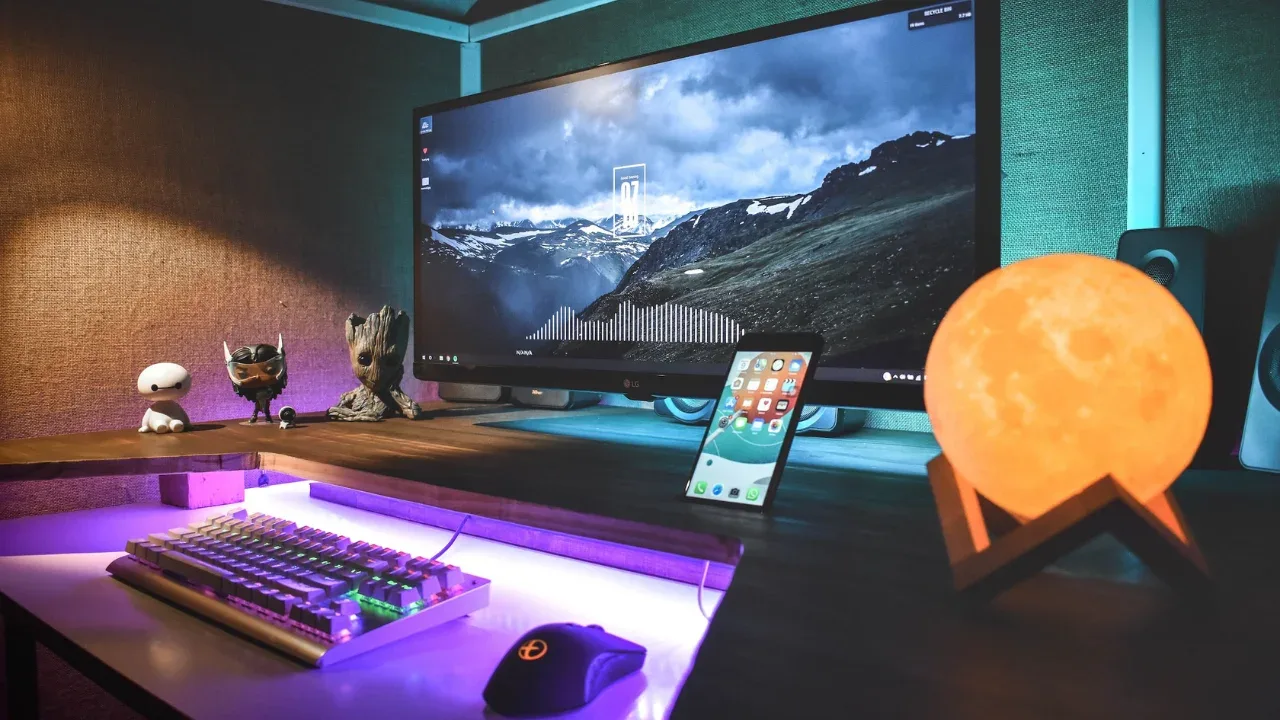
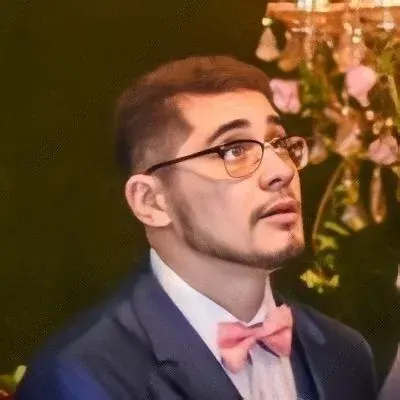
๐ How to Get Laravel 5 Email to Work with Gmail ๐
So, you're trying to send an email in Laravel 5 using Gmail as your mail provider, but you're running into an error. Don't worry, I've got you covered! In this guide, I'll walk you through common issues and provide easy solutions to get your email working seamlessly. Let's dive in!
๐จ The Error You mentioned getting the following error message:
Swift_TransportException in AbstractSmtpTransport.php line 383:
Expected response code 250 but got code "530", with message "530 5.7.1 Authentication required"
๐ Investigating the Issue This error typically indicates that Gmail requires authentication before allowing you to send emails. Let's fix this!
๐ป Solution
Update your
'driver'
,'host'
, and'port'
values inmail.php
file to the following:
'driver' => env('MAIL_DRIVER', 'smtp'),
'host' => env('MAIL_HOST', 'smtp.gmail.com'),
'port' => env('MAIL_PORT', 587),
Make sure you have the correct Gmail username and password in your
.env
file:
MAIL_USERNAME=YourGmailUsername@gmail.com
MAIL_PASSWORD=YourGmailPassword
Modify your
routes/web.php
file to include the necessary route for sending the email:
use Illuminate\Support\Facades\Mail;
Route::get('test', function () {
Mail::send('Email.test', [], function ($message) {
$message->to('example@gmail.com', 'HisName')->subject('Welcome!');
});
});
In your
MailController.php
, update yourSending_Email
method to:
use Illuminate\Support\Facades\Artisan;
use Illuminate\Support\Facades\View;
class MailController extends Controller
{
public function Sending_Email()
{
Artisan::call('config:clear');
return View::make('Email.test');
}
}
๐ Congrats! You Did It!
At this point, your email setup should be working smoothly. Now, go ahead and try sending an email by accessing the /test
route in your browser. ๐งโจ
๐ฃ Engage with the Community Did this guide help you? Have any questions or suggestions? Let's chat! Leave a comment below or reach out to me on Twitter [@YourTwitterHandle]. I can't wait to hear about your successful email sending experience! ๐
Now, go spread the word and share this guide with fellow Laravel enthusiasts who might be facing the same issue. Together, let's make email sending in Laravel 5 a breeze! ๐ช๐ป
Happy coding! ๐โจ